步驟1:操作(數據傳輸)
這是裝配體工作原理圖。
步驟2:使用的資源
1 Arduino Nano ATmega328
1 Arduino以太網模塊ENC28J60
1氣體傳感器模塊MQ2
1濕度和溫度傳感器DHT22( AM2302)
2330歐姆電阻
1 4k7歐姆電阻
1蜂鳴器5V
1個綠色LED
1紅色LED
跳線
步驟3:功能-發送方
1 ESP32 LORA Display
1氣體傳感器模塊MQ2
1濕度和溫度傳感器DHT22(AM2302)
1個4k7歐姆電阻器
跳線
第4步:使用的功能-接收器
1個ESP32 LORA OLED顯示屏
1個Arduino Nano ATmega328
1個用于Arduino的以太網模塊ENC28J60
2330 ohm r電阻器
1個蜂鳴器5V
1個綠色LED
1個紅色LED
跳線
步驟5:MQ-2氣體傳感器靈敏度
傳感器的電阻(Rs/Ro)根據現有的氣體濃度(ppm)更高或更低。此濃度可以通過引腳A0的輸出來顯示。
MQ-2氣體傳感器對氣體具有高靈敏度:
?LPG(液化石油氣);
?丙烷(C3H8);
?氫氣(H2);
?甲烷(CH4);
?燃料氣體,例如丙烷, CO,酒精和丁烷(用于打火機)。
步驟6:發送器安裝--- Pinout ESP32 LORA OLED
步驟7 :發送器電路
步驟8:發送器安裝
步驟9:安裝接收器---引腳排列Arduino Nano ATmega328
步驟10:接收器電路
步驟11:接收器安裝
綠色LED指示Arduino已連接到以太網客戶端。
紅色LED指示已發送SMS。
發送SMS時,Arduino已斷開連接,因此不再發送任何消息。
步驟12:DHT庫安裝
1。轉到草圖-》包含庫-》庫管理器。
2。搜索SimpleDHT,然后單擊“安裝”。
步驟13:發件人-代碼的組織
設置 》
循環
-readDhtSensor:負責讀取傳感器和獲取溫度和濕度值。
-gasDetected:負責讀取傳感器并驗證是否已檢測到氣體的功能。
-sendPacket:負責通過LORA發送包裹的功能
-showDisplay:負責顯示在顯示屏上獲得的消息和值的功能。
步驟14:發件人代碼[包含和定義]
首先,我們將包含定義引腳的庫。
#include //serial peripheral interface (SPI) library
#include //wifi lora library
#include //communication i2c library
#include “SSD1306.h” //display communication library
#include //dht communication library
//Descomment the line below which dht sensor type are you using
//#define DHTTYPE DHT11 // DHT 11
//#define DHTTYPE DHT21 // DHT 21 (AM2301)
#define DHTTYPE DHT22 // DHT 22 (AM2302), AM2321
我們將處理
// DHT Sensor
const int DHTPin = 23;
int analog_value; //variable used to receive the analog signal from sensor (from 100 to 10000)
int dig_value; //variable used to receive the digital signal from sensor
int gas_limit = 0; //used to indicates the minimum value to active the gas sensor (that value changes as the sensor screw is adjusted)
//h = humidity; c = Celsius temperature
float h, c;
String packSize; //variable used to receive the size of package converted in string
String packet = “OK”; //part of packet, that informs the status of sensor. That variable will be concatenate with the string “values”
String values = “|-|-”; //humidity and temperature values, separated by pipe
//parameters: address,SDA,SCL
SSD1306 display(0x3c, 4, 15); //display object
SimpleDHT22 dht22;
// Pins definition
#define SCK 5 // GPIO5 -- SX127x‘s SCK
#define MISO 19 // GPIO19 -- SX127x’s MISO
#define MOSI 27 // GPIO27 -- SX127x‘s MOSI
#define SS 18 // GPIO18 -- SX127x’s CS
#define RST 14 // GPIO14 -- SX127x‘s RESET
#define DI00 26 // GPIO26 -- SX127x’s IRQ(Interrupt Request)
#define MQ_analog 12 //analog gas sensor pin
#define MQ_dig 13 //digital gas sensor pin
#define BAND 433E6 //Radio frequency, we can still use: 433E6, 868E6, 915E6
步驟15:發送方除了定義氣體傳感器的最小觸發值外,還帶有用于接收模擬和數字信號的變量的傳感器。代碼[設置]
在設置中,我們將首先配置引腳和顯示。
void setup()
{
//set the humidity and temperature values with zero
h = c = 0;
//initialize the Serial with 9600b per second
Serial.begin(9600);
//configures analog pin as input
pinMode(MQ_analog, INPUT);
//configures digital pin as input
pinMode(MQ_dig, INPUT);
//configures oled pins as output
pinMode(16,OUTPUT);
//reset oled
digitalWrite(16, LOW);
//wait 50ms
delay(50);
//while the oled is on, GPIO16 must be HIGH
digitalWrite(16, HIGH);
//initializes the display
display.init();
//flip vertically the display
display.flipScreenVertically();
接下來,我們將在顯示屏上定義打印特性,并開始與LORA進行串行通信。然后,我們將繼續進行配置。
//set display font
display.setFont(ArialMT_Plain_10);
//wait 1500ms
delay(1500);
//clear the display
display.clear();
//initializes serial interface
SPI.begin(SCK,MISO,MOSI,SS);
//set Lora pins
LoRa.setPins(SS,RST,DI00);
//initializes the lora, seting the radio frequency
if (!LoRa.begin(BAND))
{
//draw in the position 0,0 the message in between quotation marks
display.drawString(0, 0, “Starting LoRa failed!”);
//turns on the LCD display
display.display();
//do nothing forever
while (true);
}
}
步驟16:發件人代碼[循環]
在循環中,我們還使用顯示器的特性,并指示讀取傳感器的步驟以及LORA的氣體檢測和警報發送的方法。
void loop()
{
//clear the display
display.clear();
//set the text alignment to left
display.setTextAlignment(TEXT_ALIGN_LEFT);
//sets the text font
display.setFont(ArialMT_Plain_16);
//draw in the position 0,0 the message in between quotation marks
display.drawString(0, 0, “Running.。.”);
//reads temperature sensor values
readDhtSensor();
//it concatenates on string the humidity and temperature values separated by pipe
values=“|”+String(h)+“|”+String(c);
//if the digital signal of sensor is lower, it means gas detected (inverse logic)
if(gasDetected())
{
//sets the value of the packet string to “ALARM”
packet = “ALARM”;
//it concatenates the packet with the values
packet+=values;
//sends package by LoRa
sendPacket();
//shows display, true = gas detected
showDisplay(true);
}
我們定義了將通過SMS發送的信息。
else
{
//sets the value of the packet string to “OK”
packet = “OK”;
//it concatenates the packet with the values
packet+=values;
//sends package by LoRa
sendPacket();
//shows display, false = no gas detected
showDisplay(false);
}
//waits 250ms
delay(250);
}
步驟17:發件人代碼[showDisplay]
同樣,我們處理LORA顯示屏上的數據顯示。
void showDisplay(bool gasDetected)
{
//clear the display
display.clear();
//set the text alignment to left
display.setTextAlignment(TEXT_ALIGN_LEFT);
//sets the text font
display.setFont(ArialMT_Plain_16);
//draw in the position 0,0 the message in between quotation marks
display.drawString(0, 0, “Running.。.”);
//if flag = true
if(gasDetected)
{
//draw in the position 0,20 the message in between quotation marks
display.drawString(0, 20, “Status: ALARM”);
//draw in the position 0,40 the message in between quotation marks
display.drawString(0, 40, “Gas detected!”);
//turns on the LCD display
display.display();
}
else
{
//draw in the position 0,20 the message in between quotation marks
display.drawString(0, 20, “Status: OK”);
//draw in the position 0,40 the message in between quotation marks
display.drawString(0, 40, “H: ”+String(h)+“ T: ”+String(c)+“°”);
//turns on the LCD display
display.display();
}
}
步驟18:發件人代碼[gasDetected]
在這里,如果傳感器檢測到某種類型的氣體泄漏,我們具有觸發消息的功能。
bool gasDetected()
{
//reads the analog value of the sensor
analog_value = analogRead(MQ_analog);
//reads the digital value of the sensor
dig_value = digitalRead(MQ_dig);
//obs: the serial views in this code do not influence the operation of the prototype
//shows value to the serial
Serial.print(analog_value);
//shows tab “||” to the serial
Serial.print(“ || ”);
//inverse logic
if(dig_value == 0)
{
//sets the minimum analog value
if(gas_limit == 0 || gas_limit 》 analog_value)
gas_limit = analog_value;
//shows ‘gas detected’ to the serial
Serial.println(“GAS DETECTED !!!”);
//shows the minimum gas limit to the serial
Serial.println(“Gas limit: ”+String(gas_limit));
//gas detected
return true;
}
else
{
//shows ‘no gas detected’ to the serial
Serial.println(“No gas detected.。.”);
//if first time, shows ‘X’ to the serial
if(gas_limit == 0)
Serial.println(“Gas limit: X”);
else //shows gas limit to the serial
Serial.println(“Gas limit: ”+String(gas_limit));
//no gas detected
return false;
}
}
第19步:發送方代碼[readDhtSensor]
void readDhtSensor()
{
// declaration of variables that will receive the new temperature and humidity
float novoC, novoH;
//waits 250ms
delay(250);
//set dht22 sensor values tovariables &novoC and &novoH
int err = dht22.read2(DHTPin, &novoC, &novoH, NULL);
//checks for an error
if (err != SimpleDHTErrSuccess)
{
//shows error in the serial
Serial.print(“Read DHT22 failed, err=”);
Serial.println(err);
return;
}
//if no error
//sets the variable values
c = novoC;
h = novoH;
//shows values in the serial
Serial.print((float)c); Serial.println(“ *C ”);
Serial.print((float)h); Serial.println(“ H”);
//waits 250ms
delay(250);
}
第20步:發送方代碼[sendPacket]
最后,我們打開了一個程序包,以添加用于SMS發送的數據。
void sendPacket()
{
//starts a connection to write UDP data
LoRa.beginPacket();
//send packet
LoRa.print(packet);
//returns an int: 1 if the packet was sent successfully, 0 if there was an error
LoRa.endPacket();
}
責任編輯:wv
-
傳感器
+關注
關注
2552文章
51366瀏覽量
755730 -
蜂鳴器
+關注
關注
12文章
893瀏覽量
46050 -
ESP32
+關注
關注
18文章
977瀏覽量
17463
發布評論請先 登錄
相關推薦
基于ESP32-C3FN4為核心自主研發的Wi-Fi+BT模塊-RF-WM-ESP32B1
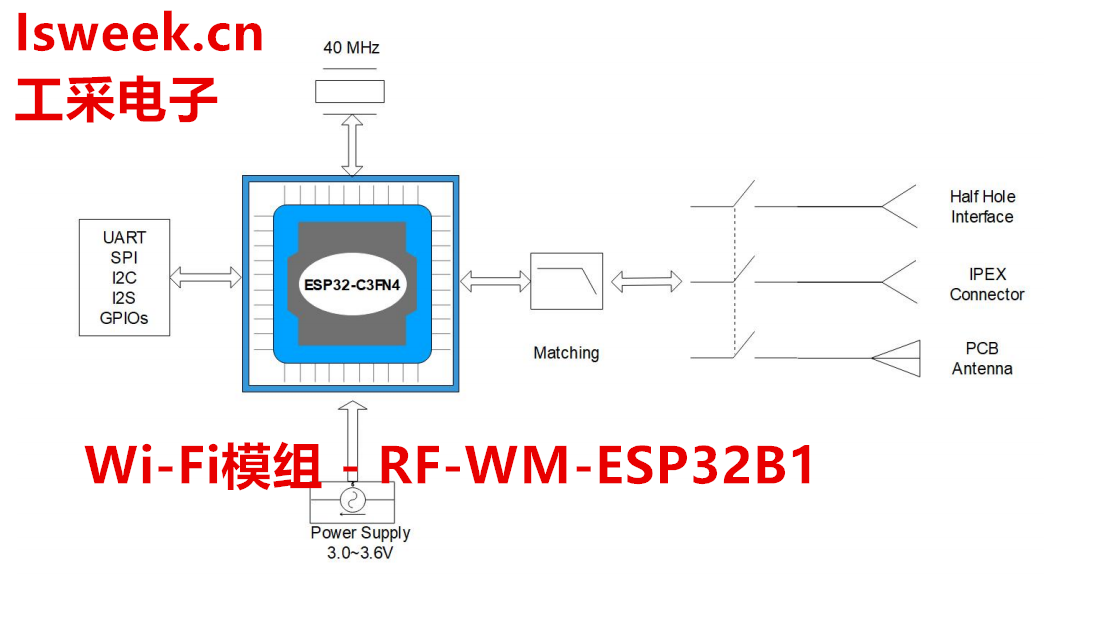
【AI技術支持】ESP32模組PSRAM的CS引腳上拉導致功耗上升處理
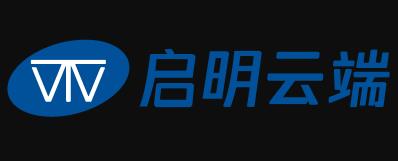
esp32上使用chatGPT做一些有意思的事情
esp8266和esp32區別是什么
esp32用什么軟件編程
ESP32-WROOM-32E、ESP32-WROOM-32D、ESP32-WROOM-32U 有什么區別?ESP32-WROOM-32 后綴字母代表的意思是?
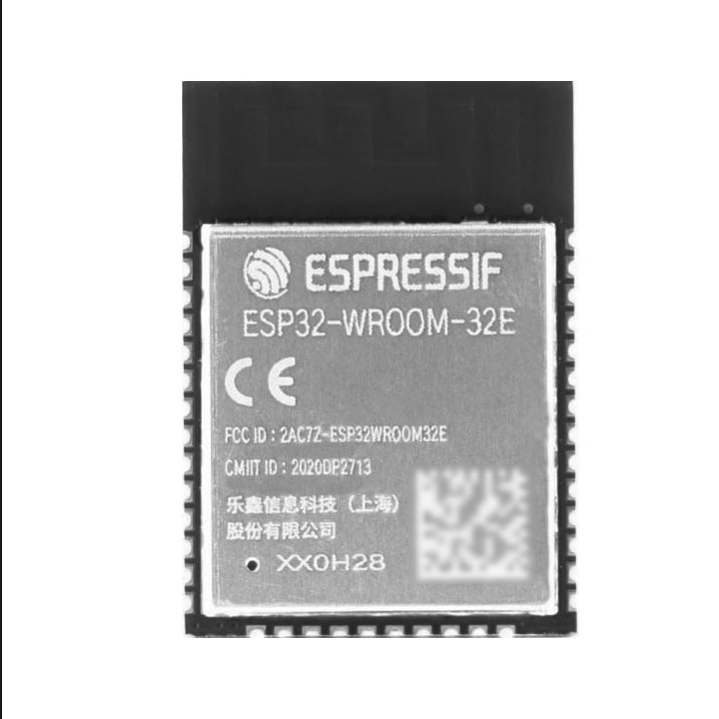
如何將AP憑據從移動設備發送到ESP模塊?
請問esp-now的安全模型是什么?
ESP32能取代STM32嗎?哪個更好?
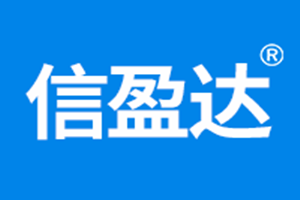
評論