python偏函數
假如一個函數定義了多個位置參數,那你每次調用時,都需要把這些個參數一個一個地傳遞進去。
比如下面這個函數,是用來計算 x的n次方
的。
def power(x, n):
s = 1
while n > 0:
n = n - 1
s = s * x
return s
那我每次計算 x 的 n 次方,都要傳遞兩個參數
>>> power(2, 2)
4
>>> power(3, 2)
9
后來我發現,我很多時候都是計算平方值,很多會去計算三次方,四次方。
那有什么辦法可以偷個懶嗎?
答案是,有。可以使用 偏函數
。
偏函數(Partial Function),可以將某個函數的常用參數進行固定,避免每次調用時都要指定。
使用偏函數,需要導入 functools.partial
,然后利用它創建一個新函數,新函數的 n 固定等2。
具體使用請看下面的示例
>>> from functools import partial
>>> power_2=partial(power, n=2)
>>> power_2(2)
4
>>> power_2(3)
9
python泛型函數
根據傳入參數類型的不同而調用不同的函數邏輯體,這種實現我們稱之為泛型。在 Python 中叫做 singledispatch
。
它使用方法極其簡單,只要被singledispatch
裝飾的函數,就是一個single-dispatch
的泛函數(generic functions
)。
單分派:根據一個參數的類型,以不同方式執行相同的操作的行為。
多分派:可根據多個參數的類型選擇專門的函數的行為。
泛函數:多個函數綁在一起組合成一個泛函數。
這邊舉個簡單的例子。
from functools import singledispatch
@singledispatch
def age(obj):
print('請傳入合法類型的參數!')
@age.register(int)
def _(age):
print('我已經{}歲了。'.format(age))
@age.register(str)
def _(age):
print('I am {} years old.'.format(age))
age(23) # int
age('twenty three') # str
age(['23']) # list
執行結果
我已經23歲了。
I am twenty three years old.
請傳入合法類型的參數!
說起泛型,其實在 Python 本身的一些內建函數中并不少見,比如 len()
, iter()
,copy.copy()
,pprint()
等
你可能會問,它有什么用呢?實際上真沒什么用,你不用它或者不認識它也完全不影響你編碼。
我這里舉個例子,你可以感受一下。
大家都知道,Python 中有許許多的數據類型,比如 str,list, dict, tuple 等,不同數據類型的拼接方式各不相同,所以我這里我寫了一個通用的函數,可以根據對應的數據類型對選擇對應的拼接方式拼接,而且不同數據類型我還應該提示無法拼接。以下是簡單的實現。
def check_type(func):
def wrapper(*args):
arg1, arg2 = args[:2]
if type(arg1) != type(arg2):
return '【錯誤】:參數類型不同,無法拼接!!'
return func(*args)
return wrapper
@singledispatch
def add(obj, new_obj):
raise TypeError
@add.register(str)
@check_type
def _(obj, new_obj):
obj += new_obj
return obj
@add.register(list)
@check_type
def _(obj, new_obj):
obj.extend(new_obj)
return obj
@add.register(dict)
@check_type
def _(obj, new_obj):
obj.update(new_obj)
return obj
@add.register(tuple)
@check_type
def _(obj, new_obj):
return (*obj, *new_obj)
print(add('hello',', world'))
print(add([1,2,3], [4,5,6]))
print(add({'name': 'wangbm'}, {'age':25}))
print(add(('apple', 'huawei'), ('vivo', 'oppo')))
# list 和 字符串 無法拼接
print(add([1,2,3], '4,5,6'))
輸出結果如下
hello, world
[1, 2, 3, 4, 5, 6]
{'name': 'wangbm', 'age': 25}
('apple', 'huawei', 'vivo', 'oppo')
【錯誤】:參數類型不同,無法拼接!!
如果不使用singledispatch 的話,你可能會寫出這樣的代碼。
def check_type(func):
def wrapper(*args):
arg1, arg2 = args[:2]
if type(arg1) != type(arg2):
return '【錯誤】:參數類型不同,無法拼接!!'
return func(*args)
return wrapper
@check_type
def add(obj, new_obj):
if isinstance(obj, str) :
obj += new_obj
return obj
if isinstance(obj, list) :
obj.extend(new_obj)
return obj
if isinstance(obj, dict) :
obj.update(new_obj)
return obj
if isinstance(obj, tuple) :
return (*obj, *new_obj)
print(add('hello',', world'))
print(add([1,2,3], [4,5,6]))
print(add({'name': 'wangbm'}, {'age':25}))
print(add(('apple', 'huawei'), ('vivo', 'oppo')))
# list 和 字符串 無法拼接
print(add([1,2,3], '4,5,6'))
輸出如下
hello, world
[1, 2, 3, 4, 5, 6]
{'name': 'wangbm', 'age': 25}
('apple', 'huawei', 'vivo', 'oppo')
【錯誤】:參數類型不同,無法拼接!!
審核編輯:符乾江
-
函數
+關注
關注
3文章
4327瀏覽量
62571 -
python
+關注
關注
56文章
4792瀏覽量
84627
發布評論請先 登錄
相關推薦
SUMIF函數與SUMIFS函數的區別
Python常用函數大全
面試常考+1:函數指針與指針函數、數組指針與指針數組
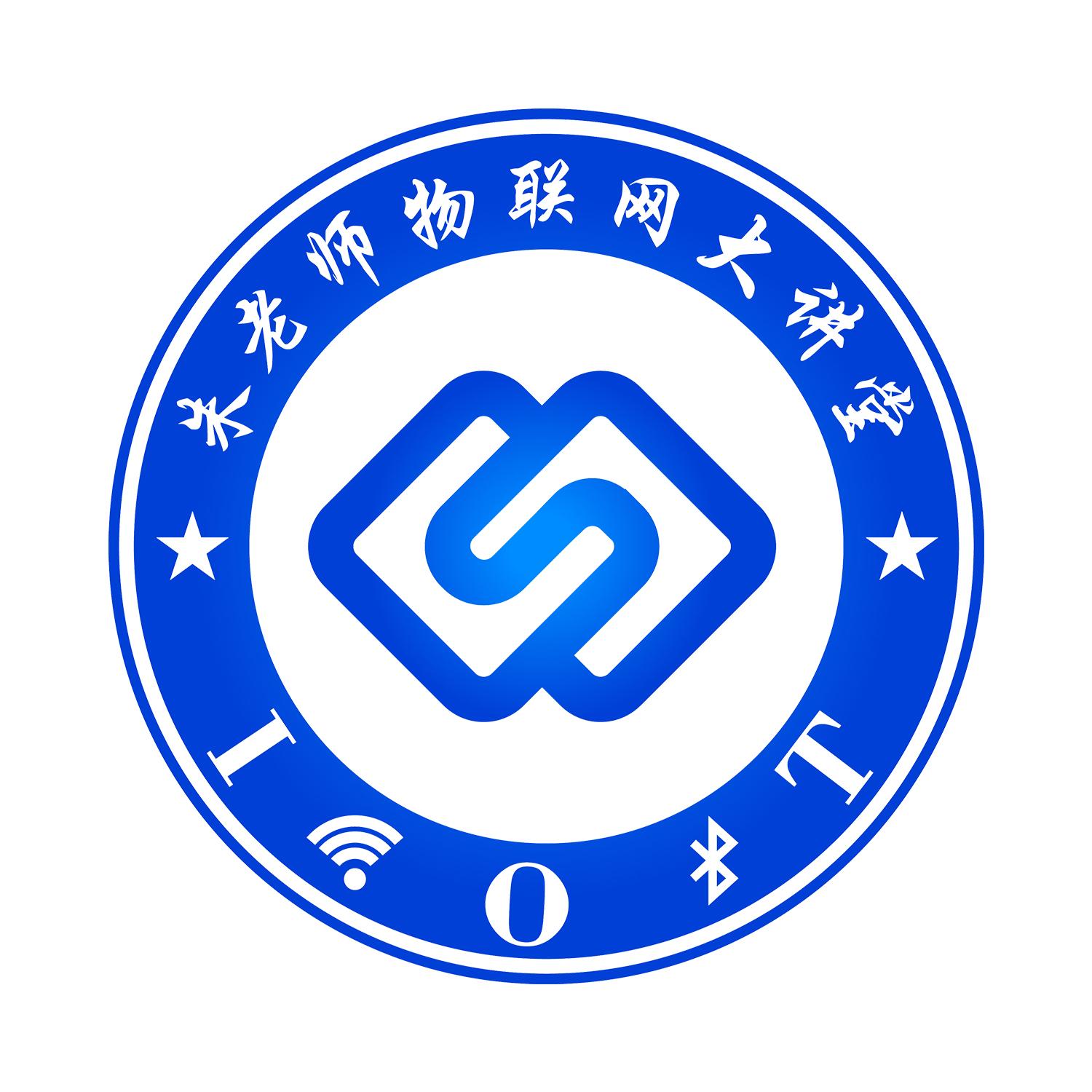
評論