1 列表生成式和生成器
from numpy import random
a = random.random(10000)
lst = []
for i in a:
lst.append(i * i) # 不推薦做法
lst = [i * i for i in a] # 使用列表生成式
gen = (i * i for i in a) # 生成器更節(jié)省內(nèi)存
2 字典推導(dǎo)式創(chuàng)建子集
a = {'apple': 5.6, 'orange': 4.7, 'banana': 2.8}
da = {key: value for key, value in a.items() if value > 4.0}
print(da) # {'apple': 5.6, 'orange': 4.7}
3 Key使用itemgetter多字段排序
from operator import itemgetter
a = [{'date': '2019-12-15', 'weather': 'cloud'},
{'date': '2019-12-13', 'weather': 'sunny'},
{'date': '2019-12-14', 'weather': 'cloud'}]
a.sort(key=itemgetter('weather', 'date'))
print(a)
# [{'date': '2019-12-14', 'weather': 'cloud'}, {'date': '2019-12-15', 'weather': 'cloud'}, {'date': '2019-12-13', 'weather': 'sunny'}]
4 Key使用itemgetter分組
from operator import itemgetter
from itertools import groupby
a.sort(key=itemgetter('weather', 'date')) # 必須先排序再分組
for k, items in groupby(a, key=itemgetter('weather')):
print(k)
for i in items:
print(i)
5 sum類聚合函數(shù)與生成器
Python中的聚合類函數(shù)sum
,min
,max
第一個參數(shù)是iterable
類型,一般使用方法如下:
a = [4,2,5,1]
sum([i+1for i in a]) # 16
使用列表生成式[i+1 for i in a]
創(chuàng)建一個長度與a
一樣的臨時列表,這步完成后,再做sum
聚合。
試想如果你的數(shù)組a
長度是百萬級,再創(chuàng)建一個這樣的臨時列表就很不劃算,最好是一邊算一邊聚合,稍改動為如下:
a = [4,2,5,1]
sum(i+1for i in a) # 16
此時i+1 for i in a
是(i+1 for i in a)
的簡寫,得到一個生成器(generator
)對象,如下所示:
In [8]:(i+1for i in a)
OUT [8]: at 0x000002AC7FFA8CF0>
生成器每迭代一步吐出(yield
)一個元素并計算和聚合后,進入下一次迭代,直到終點。
6 ChainMap邏輯上合并多個字典
dic1 = {'x': 1, 'y': 2 }
dic2 = {'y': 3, 'z': 4 }
merged = {**dic1, **dic2} # {'x': 1, 'y': 3, 'z': 4}
修改merged['x']=10
,dic1中的x
值不變
ChainMap
只在邏輯上
合并,在內(nèi)部創(chuàng)建了一個容納這些字典的列表。
from collections import ChainMap
merged = ChainMap(dic1,dic2)
print(merged)
# ChainMap({'x': 1, 'y': 2}, {'y': 3, 'z': 4})
使用ChainMap
合并字典,修改merged['x']=10
,dic1中的x
值審核編輯:湯梓紅
-
內(nèi)存
+關(guān)注
關(guān)注
8文章
3037瀏覽量
74151 -
生成器
+關(guān)注
關(guān)注
7文章
317瀏覽量
21054 -
python
+關(guān)注
關(guān)注
56文章
4800瀏覽量
84821
發(fā)布評論請先 登錄
相關(guān)推薦
焊接機器人六個軸分別是什么作用
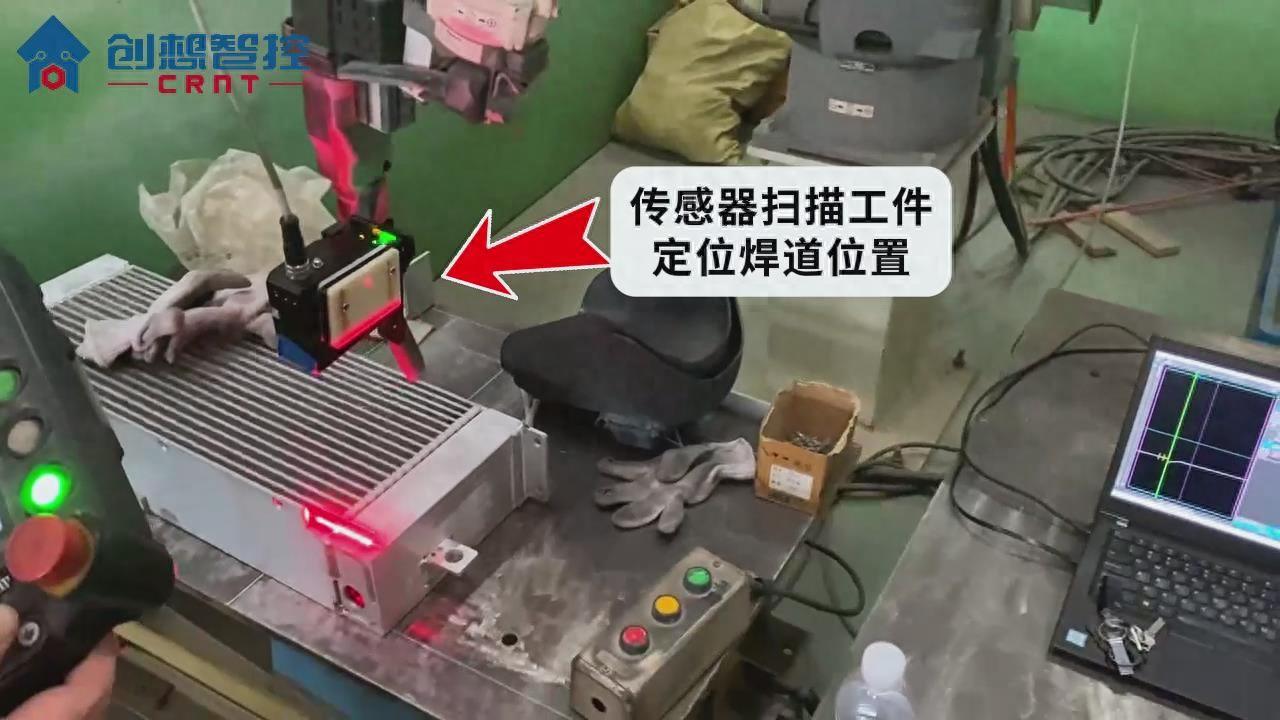
中央空管委將在六地試點eVTOL
邏輯異或運算符在Python中的用法
Python代理技術(shù)詳解:從入門到進階
無刷直流電機控制器六個功率管如何控制120度和60度的?
pytorch和python的關(guān)系是什么
如果有六個獨立的PWM通道都需要測量ADC,是不是單片的STM32H7不夠用?
具有六個200mA通道的TPS92391升壓/SEPIC 高調(diào)光性能LED驅(qū)動器數(shù)據(jù)表
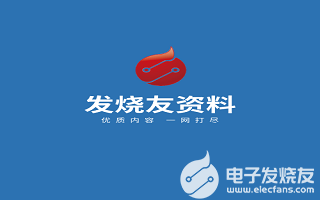
蘋果股價六連跌 蘋果最新市值
RK3568驅(qū)動指南|驅(qū)動基礎(chǔ)進階篇-進階8 內(nèi)核運行ko文件總結(jié)
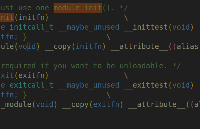
評論