首先粘貼出官方提供的can例程代碼,根據(jù)需要我將部分內(nèi)容做了修改,供大家參考!
can_sample中包換以下函數(shù): 接收數(shù)據(jù)回調(diào)函數(shù) static rt_err_t can_rx_call(rt_device_t dev, rt_size_t size)
定義can接收線程:static void can_rx_thread(void *parameter)
查找can設(shè)備:rt_device_t rt_device_find(const char* name);
打開can設(shè)備:rt_err_t rt_device_open(rt_device_t dev, rt_uint16_t oflags);
發(fā)生can數(shù)據(jù):rt_size_t rt_device_write(rt_device_t dev, rt_off_t pos, const void* buffer, rt_size_t size);
在int can_sample(int argc, char *argv[])中調(diào)用can接收線程
其中對于描述是有兩個(gè)段代碼可供參考
/*
* 程序清單:這是一個(gè) CAN 設(shè)備使用例程
* 例程導(dǎo)出了 can_sample 命令到控制終端
* 命令調(diào)用格式:can_sample can1
* 命令解釋:命令第二個(gè)參數(shù)是要使用的 CAN 設(shè)備名稱,為空則使用默認(rèn)的 CAN 設(shè)備
* 程序功能:通過 CAN 設(shè)備發(fā)送一幀,并創(chuàng)建一個(gè)線程接收數(shù)據(jù)然后打印輸出。
*/
#include
#include "rtdevice.h"
#define CAN_DEV_NAME "can1" /* CAN 設(shè)備名稱 */
static struct rt_semaphore rx_sem; /* 用于接收消息的信號量 */
static rt_device_t can_dev; /* CAN 設(shè)備句柄 */
/* 接收數(shù)據(jù)回調(diào)函數(shù) */
static rt_err_t can_rx_call(rt_device_t dev, rt_size_t size)
{
/* CAN 接收到數(shù)據(jù)后產(chǎn)生中斷,調(diào)用此回調(diào)函數(shù),然后發(fā)送接收信號量 */
rt_sem_release(&rx_sem);
return RT_EOK;
}
static void can_rx_thread(void *parameter)
{
int i;
rt_err_t res;
struct rt_can_msg rxmsg = {0};
/* 設(shè)置接收回調(diào)函數(shù) */
rt_device_set_rx_indicate(can_dev, can_rx_call);
while (1)
{
/* hdr 值為 - 1,表示直接從 uselist 鏈表讀取數(shù)據(jù) */
rxmsg.hdr = -1;
/* 阻塞等待接收信號量 */
rt_sem_take(&rx_sem, RT_WAITING_FOREVER);
/* 從 CAN 讀取一幀數(shù)據(jù) */
rt_device_read(can_dev, 0, &rxmsg, sizeof(rxmsg));
/* 打印數(shù)據(jù) ID 及內(nèi)容 */
rt_kprintf("ID:%x", rxmsg.id);
for (i = 0; i < 8; i++)
{
rt_kprintf("%2x", rxmsg.data[i]);
}
rt_kprintf("\n");
}
}
int can_sample(int argc, char *argv[])
{
struct rt_can_msg msg = {0};
rt_err_t res;
rt_size_t size;
rt_thread_t thread;
char can_name[RT_NAME_MAX];
if (argc == 2)
{
rt_strncpy(can_name, argv[1], RT_NAME_MAX);
}
else
{
rt_strncpy(can_name, CAN_DEV_NAME, RT_NAME_MAX);
}
/* 查找 CAN 設(shè)備 */
can_dev = rt_device_find(can_name);
if (!can_dev)
{
rt_kprintf("find %s failed!\n", can_name);
return RT_ERROR;
}
/* 初始化 CAN 接收信號量 */
rt_sem_init(&rx_sem, "rx_sem", 0, RT_IPC_FLAG_FIFO);
/* 以中斷接收及發(fā)送方式打開 CAN 設(shè)備 */
res = rt_device_open(can_dev, RT_DEVICE_FLAG_INT_TX | RT_DEVICE_FLAG_INT_RX);
RT_ASSERT(res == RT_EOK);
/* 創(chuàng)建數(shù)據(jù)接收線程 */
thread = rt_thread_create("can_rx", can_rx_thread, RT_NULL, 1024, 25, 10);
if (thread != RT_NULL)
{
rt_thread_startup(thread);
}
else
{
rt_kprintf("create can_rx thread failed!\n");
}
msg.id = 0x78; /* ID 為 0x78 */
msg.ide = RT_CAN_STDID; /* 標(biāo)準(zhǔn)格式 */
msg.rtr = RT_CAN_DTR; /* 數(shù)據(jù)幀 */
msg.len = 8; /* 數(shù)據(jù)長度為 8 */
/* 待發(fā)送的 8 字節(jié)數(shù)據(jù) */
msg.data[0] = 0x00;
msg.data[1] = 0x11;
msg.data[2] = 0x22;
msg.data[3] = 0x33;
msg.data[4] = 0x44;
msg.data[5] = 0x55;
msg.data[6] = 0x66;
msg.data[7] = 0x77;
/* 發(fā)送一幀 CAN 數(shù)據(jù) */
size = rt_device_write(can_dev, 0, &msg, sizeof(msg));
if (size == 0)
{
rt_kprintf("can dev write data failed!\n");
}
return res;
}
void can_send_test(void)
{
struct rt_can_msg msg = {0};
rt_size_t size;
static rt_uint8_t num = 0;
msg.id = 0x78; /* ID 為 0x78 */
msg.ide = RT_CAN_STDID; /* 標(biāo)準(zhǔn)格式 */
msg.rtr = RT_CAN_DTR; /* 數(shù)據(jù)幀 */
msg.len = 8; /* 數(shù)據(jù)長度為 8 */
/* 待發(fā)送的 8 字節(jié)數(shù)據(jù) */
msg.data[0] = 0x00;
msg.data[1] = num++; //can發(fā)送數(shù)據(jù)隨意更改
msg.data[2] = 0x22;
msg.data[3] = 0x33;
msg.data[4] = num++;
msg.data[5] = 0x55;
msg.data[6] = 0x66;
msg.data[7] = 0x77;
/* 發(fā)送一幀 CAN 數(shù)據(jù) */
size = rt_device_write(can_dev, 0, &msg, sizeof(msg));
if (size == 0)
{
rt_kprintf("can dev write data failed!\n");
}
}
/* 導(dǎo)出到 msh 命令列表中 */
MSH_CMD_EXPORT(can_sample, can device sample);
MSH_CMD_EXPORT(can_send_test, can send test);
另外一個(gè)版本,實(shí)現(xiàn)的功能是一樣的
/*
* 程序清單:這是一個(gè) CAN 設(shè)備使用例程
* 例程導(dǎo)出了 can_sample 命令到控制終端
* 命令調(diào)用格式:can_sample can1
* 命令解釋:命令第二個(gè)參數(shù)是要使用的 CAN 設(shè)備名稱,為空則使用默認(rèn)的 CAN 設(shè)備
* 程序功能:通過 CAN 設(shè)備發(fā)送一幀,并創(chuàng)建一個(gè)線程接收數(shù)據(jù)然后打印輸出。
*/
#include
#include "rtdevice.h"
#define CAN_DEV_NAME "can1" /* CAN 設(shè)備名稱 */
static struct rt_semaphore rx_sem; /* 用于接收消息的信號量 */
static rt_device_t can_dev; /* CAN 設(shè)備句柄 */
#define THREAD_PRIORITY 25
#define THREAD_STACK_SIZE 512
#define THREAD_TIMESLICE 5
static rt_thread_t tid1 = RT_NULL;
/* 接收數(shù)據(jù)回調(diào)函數(shù) */
static rt_err_t can_rx_call(rt_device_t dev, rt_size_t size) {
/* CAN 接收到數(shù)據(jù)后產(chǎn)生中斷,調(diào)用此回調(diào)函數(shù),然后發(fā)送接收信號量 */
rt_sem_release(&rx_sem);
return RT_EOK;
}
static void can_rx_thread(void *parameter) {
int i;
rt_err_t res;
struct rt_can_msg rxmsg = {0};
/* 設(shè)置接收回調(diào)函數(shù) */
rt_device_set_rx_indicate(can_dev, can_rx_call);
while (1) {
/* hdr 值為 - 1,表示直接從 uselist 鏈表讀取數(shù)據(jù) */
rxmsg.hdr = -1;
/* 阻塞等待接收信號量 */
rt_sem_take(&rx_sem, RT_WAITING_FOREVER);
/* 從 CAN 讀取一幀數(shù)據(jù) */
rt_device_read(can_dev, 0, &rxmsg, sizeof(rxmsg));
/* 打印數(shù)據(jù) ID 及內(nèi)容 */
rt_kprintf("ID:%x", rxmsg.id);
for (i = 0; i < 8; i++) {
rt_kprintf("%2x", rxmsg.data[i]);
}
rt_kprintf("\n");
}
}
/* 線程 1 的入口函數(shù) */
static void thread1_entry(void *parameter) {
struct rt_can_msg msg = {0};
unsigned long count = 0;
msg.id = 0x78; /* ID 為 0x78 */
msg.ide = RT_CAN_STDID; /* 標(biāo)準(zhǔn)格式 */
msg.rtr = RT_CAN_DTR; /* 數(shù)據(jù)幀 */
msg.len = 8; /* 數(shù)據(jù)長度為 3 */
/* 待發(fā)送的 3 字節(jié)數(shù)據(jù) */
msg.data[0] = 0x00;
msg.data[1] = 0x11;
msg.data[2] = 0x22;
msg.data[3] = 0x00;
msg.data[4] = 0x11;
msg.data[5] = 0x00;
msg.data[6] = 0x11;
msg.data[7] = 0x22;
rt_kprintf("send %ld \n", ++count);
while (1) {
/* 線程 1 采用低優(yōu)先級運(yùn)行,一直打印計(jì)數(shù)值 */
rt_device_write(can_dev, 0, &msg, sizeof(msg));
rt_kprintf("send %ld \n", ++count);
rt_thread_mdelay(500);
}
}
int can_sample(int argc, char *argv[]) {
rt_err_t res;
rt_size_t size;
rt_thread_t thread;
char can_name[RT_NAME_MAX];
if (argc == 2) {
rt_strncpy(can_name, argv[1], RT_NAME_MAX);
} else {
rt_strncpy(can_name, CAN_DEV_NAME, RT_NAME_MAX);
}
/* 查找 CAN 設(shè)備 */
can_dev = rt_device_find(can_name);
if (!can_dev) {
rt_kprintf("find %s failed!\n", can_name);
return RT_ERROR;
}
/* 初始化 CAN 接收信號量 */
rt_sem_init(&rx_sem, "rx_sem", 0, RT_IPC_FLAG_FIFO);
/* 以中斷接收及發(fā)送方式打開 CAN 設(shè)備 */
res = rt_device_open(can_dev, RT_DEVICE_FLAG_INT_TX | RT_DEVICE_FLAG_INT_RX);
/* 設(shè)置 CAN 的工作模式為正常工作模式 */
res = rt_device_control(can_dev, RT_CAN_CMD_SET_MODE, (void *)RT_CAN_MODE_NORMAL);
res = rt_device_control(can_dev, RT_CAN_CMD_SET_BAUD, (void *)CAN500kBaud);
RT_ASSERT(res == RT_EOK);
/* 創(chuàng)建數(shù)據(jù)接收線程 */
thread = rt_thread_create("can_rx", can_rx_thread, RT_NULL, 1024, 25, 10);
if (thread != RT_NULL) {
rt_thread_startup(thread);
} else {
rt_kprintf("create can_rx thread failed!\n");
}
if (size == 0) {
rt_kprintf("can dev write data failed!\n");
}
/* 創(chuàng)建線程 1,名稱是 thread1,入口是 thread1_entry*/
tid1 = rt_thread_create("thread1",
thread1_entry, RT_NULL,
THREAD_STACK_SIZE,
THREAD_PRIORITY, THREAD_TIMESLICE);
/* 如果獲得線程控制塊,啟動(dòng)這個(gè)線程 */
if (tid1 != RT_NULL)
rt_thread_startup(tid1);
else
rt_kprintf("start can send fail\n");
return res;
}
/* 導(dǎo)出到 msh 命令列表中 */
MSH_CMD_EXPORT(can_sample, can device sample);
代碼編譯完成,下載。串口運(yùn)行:can_sample,打開創(chuàng)芯科技CAN卡,打開電腦的USB CAN工具
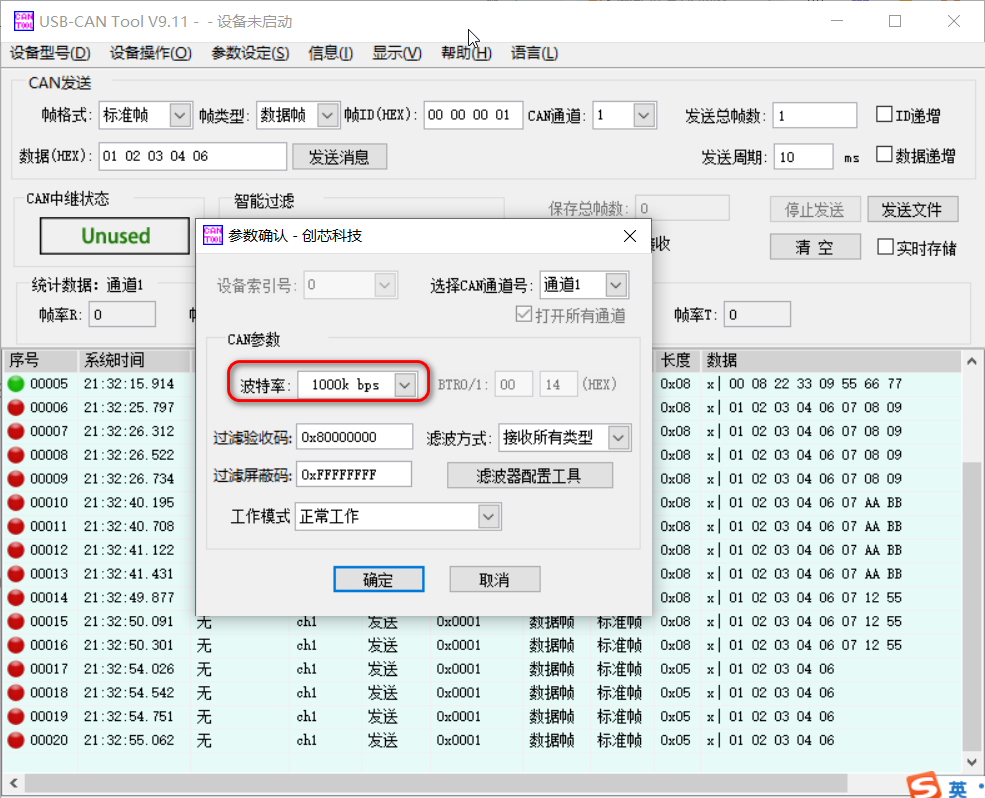
打開USB轉(zhuǎn)CAN,選擇對應(yīng)波特率,這里配置為1Mbps。
重啟,再次運(yùn)行can_sample,發(fā)現(xiàn),接收到STM32發(fā)出的CAN數(shù)據(jù)幀。
多次運(yùn)行 can_send_test,電腦端可以接受數(shù)據(jù)。
-
CAN
+關(guān)注
關(guān)注
57文章
2756瀏覽量
463893 -
RT-Thread
+關(guān)注
關(guān)注
31文章
1293瀏覽量
40228
發(fā)布評論請先 登錄
相關(guān)推薦
RT-Thread上CAN實(shí)踐
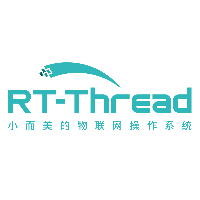
【原創(chuàng)精選】RT-Thread征文精選技術(shù)文章合集
RT-Thread編程指南
RT-Thread上的CAN總線介紹以及驅(qū)動(dòng)編寫
基于 Keil MDK 移植 RT-Thread Nano
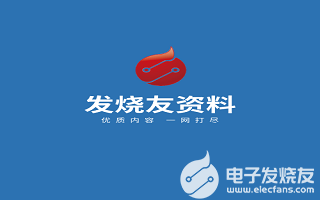
RT-Thread全球技術(shù)大會:Kconfig在RT-Thread中的工作機(jī)制
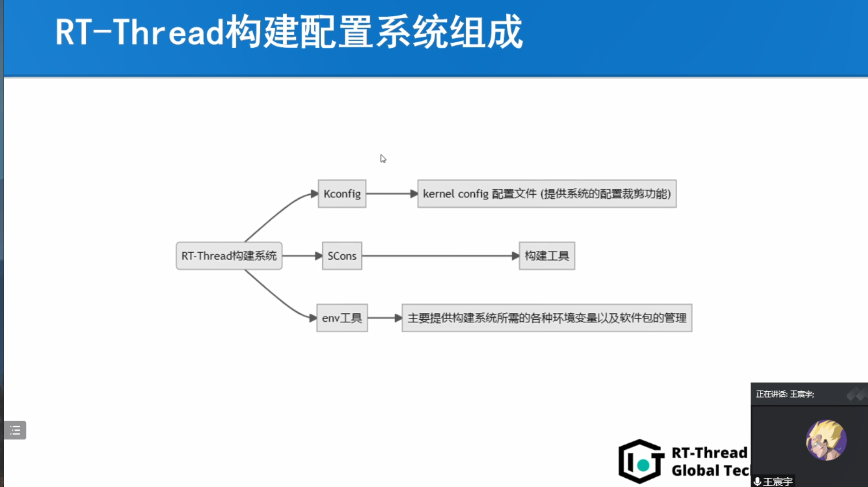
RT-Thread全球技術(shù)大會:RT-Thread對POSIX的實(shí)現(xiàn)情況介紹
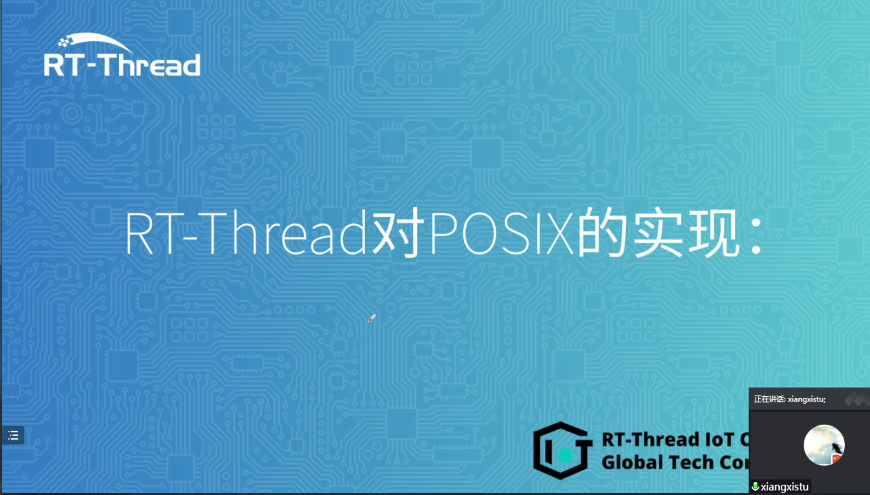
RT-Thread學(xué)習(xí)筆記 RT-Thread的架構(gòu)概述
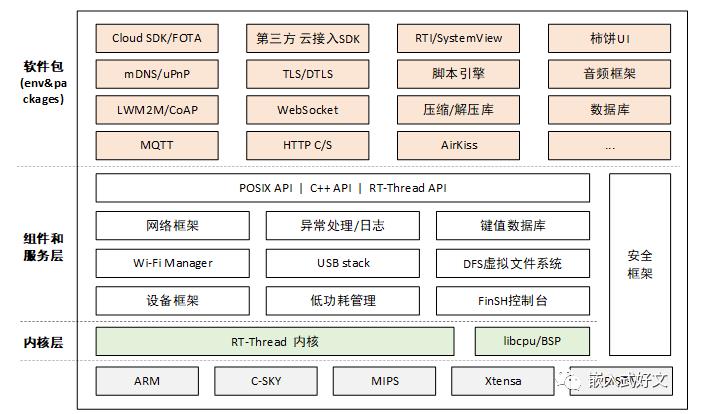
RT-Thread文檔_RT-Thread 潘多拉 STM32L475 上手指南
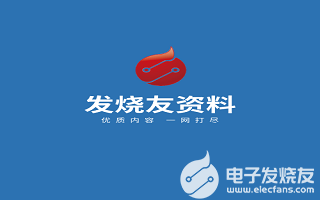
基于RT-Thread Studio學(xué)習(xí)
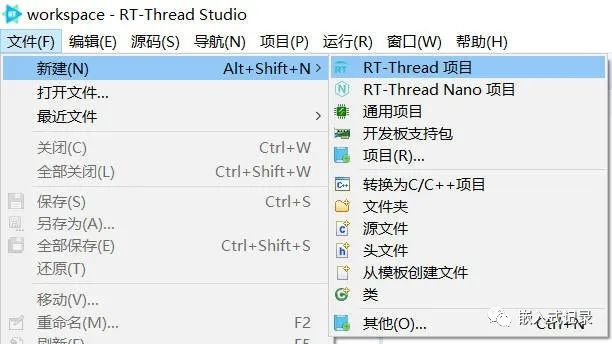
4月10日深圳場RT-Thread線下workshop,探索RT-Thread混合部署新模式!
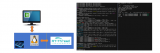
評論