1- 簡介
每個系統任務都會有一個任務通知。然后每個任務通知都具有掛起或者未掛起的狀態,以及32位的通知。常量configTASK_NOTIFICATION_ARRAY_ENTRIES()是用來設置任務通知索引的數組。
任務通知是直接發送給任務事件,不是通過中間對象(隊列、事件組、信號量)間接發送給任務的。
當任務發送任務通知時,會將目標任務通知的狀態設定位掛起狀態。就像任務阻塞中間對象一樣,例如,信號在等待信號量的可用情況,任務組織這個等待通知的狀態變為掛起的狀態。
任務通知也可以是以下幾種方式:
- 值覆蓋,不管接受任務是否已經讀取被覆蓋的值。
- 值覆蓋,僅當接收任務已讀取被覆蓋的值時。
- 在設置值中設置一個或多個位。
- 遞增值(加1)
注意:
每個數組中的通知都是獨立的,一個任務一次只能阻塞數組中的一個通知,而且不會通過發送到任何其他數組索引的直通解除阻塞狀態。
1.1 優勢和使用限制
任務通知的靈活性允許它們在需要創建單獨隊列、二進制信號量、計數信號量或事件組的情況下使用。使用直接通知解除RTOS任務的阻塞速度比使用中間對象(如二進制信號量)解除阻塞的速度快45% *,并且使用的RAM更少。正如所料,這些性能優勢需要一些用例限制。
1-當只有一個任務可以接收事件時,才可以使用RTOS任務通知。然而,實際應用中的大多數用例都滿足這個條件,例如中斷解除阻塞,任務將處理由中斷接收到的數據。
2-只有在使用RTOS任務通知代替隊列的情況下:接收任務可以在阻塞狀態下等待通知(這樣不會消耗任何CPU時間),如果發送任務不能立即完成,則發送任務不能在阻塞狀態下等待發送完成。
1.2 用例
通知使用xTaskNotifyIndexed()和xTaskNotifyGiveIndexed() API函數(和它們的中斷安全等效)發送,并保持等待,直到接收RTOS任務調用xTaskNotifyWaitIndexed()或ulTaskNotifyTakeIndexed() API函數。這些API函數都有一個不帶“I索引”前綴的等價函數。非“索引”版本總是在數組索引0處的任務通知上操作。例如,xTaskNotifyGive(TargetTask)等價于xTaskNotifyGiveIndexed(TargetTask, 0) -兩者都在索引0處增加任務通知由任務處理的TargetTask引用的任務。
2-將 RTOS 任務通知用作輕量級二進制信號量
與使用二進制信號量解鎖任務相比,使用直接通知解鎖 RTOS 任務的速度快 45%,并且使用的 RAM 更少。
二進制信號量是最大計數為 1 的信號量,因此稱為“二進制”。任務只有在信號量可用時才可以“獲取”信號量,并且信號量僅 如果計數為 1,則可用。
當使用任務通知代替二進制信號量時,接收任務的通知值會代替二進制信號量的count值,并且會使用ulTaskNotifyTake()(或ulTaskNotifyTakeIndexed()) API函數代替信號量的xSemaphoreTake() API函數。
ulTaskNotifyTake()函數的xClearOnExit參數被設置為pdTRUE,因此每次收到通知時count值都返回0——模擬二進制信號量。
同樣,xTaskNotifyGive()(或xTaskNotifyGiveIndexed())或vTaskNotifyGiveFromISR()(或vTaskNotifyGiveIndexedFromISR())函數被用來代替信號量的xSemaphoreGive()和xSemaphoreGiveFromISR()函數。
2.1 使用范例
1/*這是通用外設驅動程序中的傳輸函數的一個例子。
2RTOS任務調用傳輸函數,然后處于阻塞狀態(因此不占用CPU時間),
3直到收到傳輸完成的通知。傳輸由DMA執行,DMA端中斷用于通知任務。 */
4
5/* 存儲傳輸完成時將收到通知的任務句柄。 */
6static TaskHandle_t xTaskToNotify = NULL;
7
8/* 目標任務要使用的任務通知數組中的索引。 */
9const UBaseType_t xArrayIndex = 1;
10
11/* 外設驅動的傳輸功能 */
12void StartTransmission( uint8_t *pcData, size_t xDataLength )
13{
14/*此時xTaskToNotify應該為NULL,因為沒有正在進行傳輸。
15如果有必要,可以使用互斥量來保護對外設的訪問。*/
16configASSERT( xTaskToNotify == NULL );
17
18/* 存儲調用任務的句柄。 */
19xTaskToNotify = xTaskGetCurrentTaskHandle();
20
21/* 開始傳輸:在傳輸完成時產生一個中斷。 */
22vStartTransmit( pcData, xDatalength );
23}
24/*-----------------------------------------------------------*/
25
26/* 結束中斷傳輸 */
27void vTransmitEndISR( void )
28{
29BaseType_t xHigherPriorityTaskWoken = pdFALSE;
30
31/* 此時,xTaskToNotify不應該為NULL,因為傳輸正在進行中。 */
32configASSERT( xTaskToNotify != NULL );
33
34/* Notify the task that the transmission is complete. */
35vTaskNotifyGiveIndexedFromISR( xTaskToNotify,
36xArrayIndex,
37&xHigherPriorityTaskWoken );
38
39/*此時,xTaskToNotify不應該為NULL,因為傳輸正在進行中。 */
40xTaskToNotify = NULL;
41
42/*如果xHigherPriorityTaskWoken現在設置為pdTRUE,
43那么應該執行切換,以確保中斷直接返回到最高優先級的任務。
44用于該目的的宏取決于所使用的端口,可以稱為portEND_SWITCHING_ISR()。 */
45portYIELD_FROM_ISR( xHigherPriorityTaskWoken );
46}
47/*-----------------------------------------------------------*/
48
49/* 發起傳輸的任務,然后進入阻塞狀態(因此不消耗任何CPU時間),以等待傳輸完成。 */
50void vAFunctionCalledFromATask( uint8_t ucDataToTransmit,
51size_t xDataLength )
52{
53uint32_t ulNotificationValue;
54const TickType_t xMaxBlockTime = pdMS_TO_TICKS( 200 );
55
56/* 通過調用上面顯示的函數開始傳輸。 */
57StartTransmission( ucDataToTransmit, xDataLength );
58
59/* 等待傳輸完成的通知。注意,第一個參數是pdTRUE,
60它的作用是將任務的通知值清除回0,
61使通知值類似于二進制(而不是計數)信號量。 */
62ulNotificationValue = ulTaskNotifyTakeIndexed( xArrayIndex,
63pdTRUE,
64xMaxBlockTime );
65
66if( ulNotificationValue == 1 )
67{
68/* The transmission ended as expected. */
69}
70else
71{
72/* The call to ulTaskNotifyTake() timed out. */
73}
74}
3-使用 RTOS 任務通知作為輕量級計數信號量
與使用信號量解鎖任務相比,使用直接通知解鎖 RTOS 任務的速度快 45%,并且使用的 RAM 更少。
計數信號量是這樣一種信號量,其計數值可以為0,直到創建信號量時設置的最大值。只有當信號量可用時,任務才能獲取信號量,并且只有當信號量的計數大于0時,信號量才可用。
當使用任務通知來代替計數信號量時,接收任務的通知值會代替計數信號量的計數值,并且使用ulTaskNotifyTake()(或ulTaskNotifyTakeIndexed()) API函數來代替信號量的xSemaphoreTake() API函數。ulTaskNotifyTake()函數的xClearOnExit參數被設置為pdFALSE,因此每次收到通知時,計數值只減少(而不是清除)——模擬計數信號量。
同樣,xTaskNotifyGive()(或xTaskNotifyGiveIndexed())或vTaskNotifyGiveFromISR()(或vTaskNotifyGiveIndexedFromISR())函數被用來代替信號量的xSemaphoreGive()和xSemaphoreGiveFromISR()函數。
下面通過兩個例子來看。
下面的第一個例子使用接收任務的通知值作為計數信號量。第二個示例提供了高效的實現方式。
3.1 Example 1:
1/* An interrupt handler that does not process interrupts directly,
2but instead defers processing to a high priority RTOS task. The
3ISR uses RTOS task notifications to both unblock the RTOS task
4and increment the RTOS task's notification value. */
5void vANInterruptHandler( void )
6{
7BaseType_t xHigherPriorityTaskWoken;
8
9/* Clear the interrupt. */
10prvClearInterruptSource();
11
12/* xHigherPriorityTaskWoken must be initialised to pdFALSE.
13If calling vTaskNotifyGiveFromISR() unblocks the handling
14task, and the priority of the handling task is higher than
15the priority of the currently running task, then
16xHigherPriorityTaskWoken will be automatically set to pdTRUE. */
17xHigherPriorityTaskWoken = pdFALSE;
18
19/* Unblock the handling task so the task can perform
20any processing necessitated by the interrupt. xHandlingTask
21is the task's handle, which was obtained when the task was
22created. vTaskNotifyGiveFromISR() also increments
23the receiving task's notification value. */
24vTaskNotifyGiveFromISR( xHandlingTask, &xHigherPriorityTaskWoken );
25
26/* Force a context switch if xHigherPriorityTaskWoken is now
27set to pdTRUE. The macro used to do this is dependent on
28the port and may be called portEND_SWITCHING_ISR. */
29portYIELD_FROM_ISR( xHigherPriorityTaskWoken );
30}
31/*-----------------------------------------------------------*/
32
33/* A task that blocks waiting to be notified that the peripheral
34needs servicing. */
35void vHandlingTask( void *pvParameters )
36{
37BaseType_t xEvent;
38const TickType_t xBlockTime = pdMS_TO_TICS( 500 );
39uint32_t ulNotifiedValue;
40
41for( ;; )
42{
43/* Block to wait for a notification. Here the RTOS
44task notification is being used as a counting semaphore.
45The task's notification value is incremented each time
46the ISR calls vTaskNotifyGiveFromISR(), and decremented
47each time the RTOS task calls ulTaskNotifyTake() - so in
48effect holds a count of the number of outstanding interrupts.
49The first parameter is set to pdFALSE, so the notification
50value is only decremented and not cleared to zero, and one
51deferred interrupt event is processed at a time. See
52example 2 below for a more pragmatic approach. */
53ulNotifiedValue = ulTaskNotifyTake( pdFALSE,
54xBlockTime );
55
56if( ulNotifiedValue > 0 )
57{
58/* Perform any processing necessitated by the interrupt. */
59xEvent = xQueryPeripheral();
60
61if( xEvent != NO_MORE_EVENTS )
62{
63vProcessPeripheralEvent( xEvent );
64}
65}
66else
67{
68/* Did not receive a notification within the expected
69time. */
70vCheckForErrorConditions();
71}
72}
73}
3.2 Example 2:
1這個例子展示了一個更實用和高效的RTOS任務的實現。在這個實現中,ulTaskNotifyTake()的返回值用于知道有多少未處理的ISR事件必須被處理,允許RTOS任務的通知計數在每次ulTaskNotifyTake()被調用時被清除回零。中斷服務例程(ISR)假定如上例1所示。
2/* The index within the target task's array of task notifications
3to use. */
4const UBaseType_t xArrayIndex = 0;
5
6/* A task that blocks waiting to be notified that the peripheral
7needs servicing. */
8void vHandlingTask( void *pvParameters )
9{
10BaseType_t xEvent;
11const TickType_t xBlockTime = pdMS_TO_TICS( 500 );
12uint32_t ulNotifiedValue;
13
14for( ;; )
15{
16/* As before, block to wait for a notification form the ISR.
17This time however the first parameter is set to pdTRUE,
18clearing the task's notification value to 0, meaning each
19outstanding outstanding deferred interrupt event must be
20processed before ulTaskNotifyTake() is called again. */
21ulNotifiedValue = ulTaskNotifyTakeIndexed( xArrayIndex,
22pdTRUE,
23xBlockTime );
24
25if( ulNotifiedValue == 0 )
26{
27/* Did not receive a notification within the expected
28time. */
29vCheckForErrorConditions();
30}
31else
32{
33/* ulNotifiedValue holds a count of the number of
34outstanding interrupts. Process each in turn. */
35while( ulNotifiedValue > 0 )
36{
37xEvent = xQueryPeripheral();
38
39if( xEvent != NO_MORE_EVENTS )
40{
41vProcessPeripheralEvent( xEvent );
42ulNotifiedValue--;
43}
44else
45{
46break;
47}
48}
49}
50}
51}
4-將 RTOS 任務通知用作輕量級事件組
事件組是一組二進制標志(或位),應用程序編寫人員可以為每個標志指定含義。RTOS進程可以進入阻塞狀態,等待組內的一個或多個標志變為活動狀態。當RTOS任務處于阻塞狀態時,不會消耗任何CPU時間。
當使用任務通知代替事件組時,使用接收任務的通知值代替事件組,接收任務的通知值中的比特位用作事件標志,并且使用xTaskNotifyWait() API函數代替事件組的xEventGroupWaitBits() API函數。
同樣,使用xTaskNotify()和xTaskNotifyFromISR() API函數(其eAction參數設置為eSetBits)來代替xEventGroupSetBits()和xEventGroupSetBitsFromISR()函數。
與xEventGroupSetBitsFromISR()相比,xTaskNotifyFromISR()具有顯著的性能優勢,因為xTaskNotifyFromISR()完全在ISR中執行,而xEventGroupSetBitsFromISR()必須推遲一些處理到RTOS進程任務。
與使用事件組時不同,接收任務不能指定只在同時有多個比特位處于活動狀態時才離開阻塞狀態。相反,在任何比特位處于活動狀態時,進程將解除阻塞,并且必須自己測試比特位的組合。
1/* This example demonstrates a single RTOS task being used to process
2events that originate from two separate interrupt service routines -
3a transmit interrupt and a receive interrupt. Many peripherals will
4use the same handler for both, in which case the peripheral's
5interrupt status register can simply be bitwise ORed with the
6receiving task's notification value.
7
8First bits are defined to represent each interrupt source. */
9#define TX_BIT 0x01
10#define RX_BIT 0x02
11
12/* The handle of the task that will receive notifications from the
13interrupts. The handle was obtained when the task
14was created. */
15static TaskHandle_t xHandlingTask;
16
17/*-----------------------------------------------------------*/
18
19/* The implementation of the transmit interrupt service routine. */
20void vTxISR( void )
21{
22BaseType_t xHigherPriorityTaskWoken = pdFALSE;
23
24/* Clear the interrupt source. */
25prvClearInterrupt();
26
27/* Notify the task that the transmission is complete by setting the TX_BIT
28in the task's notification value. */
29xTaskNotifyFromISR( xHandlingTask,
30TX_BIT,
31eSetBits,
32&xHigherPriorityTaskWoken );
33
34/* If xHigherPriorityTaskWoken is now set to pdTRUE then a context switch
35should be performed to ensure the interrupt returns directly to the highest
36priority task. The macro used for this purpose is dependent on the port in
37use and may be called portEND_SWITCHING_ISR(). */
38portYIELD_FROM_ISR( xHigherPriorityTaskWoken );
39}
40/*-----------------------------------------------------------*/
41
42/* The implementation of the receive interrupt service routine is identical
43except for the bit that gets set in the receiving task's notification value. */
44void vRxISR( void )
45{
46BaseType_t xHigherPriorityTaskWoken = pdFALSE;
47
48/* Clear the interrupt source. */
49prvClearInterrupt();
50
51/* Notify the task that the reception is complete by setting the RX_BIT
52in the task's notification value. */
53xTaskNotifyFromISR( xHandlingTask,
54RX_BIT,
55eSetBits,
56&xHigherPriorityTaskWoken );
57
58/* If xHigherPriorityTaskWoken is now set to pdTRUE then a context switch
59should be performed to ensure the interrupt returns directly to the highest
60priority task. The macro used for this purpose is dependent on the port in
61use and may be called portEND_SWITCHING_ISR(). */
62portYIELD_FROM_ISR( xHigherPriorityTaskWoken );
63}
64/*-----------------------------------------------------------*/
65
66/* The implementation of the task that is notified by the interrupt service
67routines. */
68static void prvHandlingTask( void *pvParameter )
69{
70const TickType_t xMaxBlockTime = pdMS_TO_TICKS( 500 );
71BaseType_t xResult;
72
73for( ;; )
74{
75/* Wait to be notified of an interrupt. */
76xResult = xTaskNotifyWait( pdFALSE, /* Don't clear bits on entry. */
77ULONG_MAX, /* Clear all bits on exit. */
78&ulNotifiedValue, /* Stores the notified value. */
79xMaxBlockTime );
80
81if( xResult == pdPASS )
82{
83/* A notification was received. See which bits were set. */
84if( ( ulNotifiedValue & TX_BIT ) != 0 )
85{
86/* The TX ISR has set a bit. */
87prvProcessTx();
88}
89
90if( ( ulNotifiedValue & RX_BIT ) != 0 )
91{
92/* The RX ISR has set a bit. */
93prvProcessRx();
94}
95}
96else
97{
98/* Did not receive a notification within the expected time. */
99prvCheckForErrors();
100}
101}
102}
5-將 RTOS 任務通知用作輕量級郵箱
RTOS任務通知可用于向任務發送數據,但與RTOS隊列相比,發送數據的方式要嚴格得多,因為:
-
只能發送 32 位值
-
該值被保存為接收任務的通知值,并且在任何時間只能有一個通知值,因此短語“輕量級郵箱”優先于“輕量級隊列”。任務的通知值是郵箱值。
-
數據使用xTaskNotify()(或xTaskNotifyIndexed())和xTaskNotifyFromISR()(或xTaskNotifyIndexedFromISR()) API函數發送到任務,其eAction參數設置為eSetValueWithOverwrite或eSetValueWithoutOverwrite。如果eAction設置為eSetValueWithOverwrite,那么即使接收任務已經有一個待處理的通知,也會更新接收任務的通知值。如果eAction設置為esetvaluewithoutooverwrite,則只有在接收任務沒有待處理通知時才更新接收任務的通知值——因為更新通知值將覆蓋接收任務處理之前的值。
任務可以使用xTaskNotifyWait()(或xTaskNotifyWaitIndexed())讀取它自己的通知值。
-
函數
+關注
關注
3文章
4327瀏覽量
62573 -
RTOS
+關注
關注
22文章
811瀏覽量
119595 -
FreeRTOS
+關注
關注
12文章
484瀏覽量
62143
發布評論請先 登錄
相關推薦
FreeRTOS中的任務管理
FreeRTOS任務通知模擬二值信號量怎么都獲取不成功是怎么回事
FreeRTOS任務通知相關資料分享
FreeRTOS的直接任務(消息)通知
FreeRTOS —— 9.任務通知
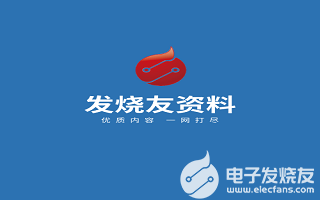
FreeRTOS高級篇8---FreeRTOS任務通知分析
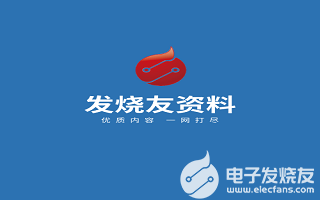
FreeRTOS系列第15篇---使用任務通知實現命令行解釋器
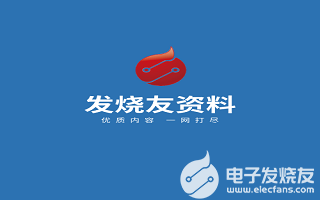
FreeRTOS系列第14篇---FreeRTOS任務通知
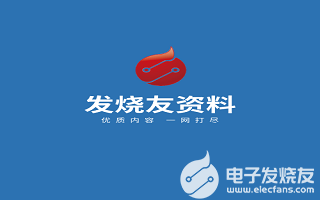
FreeRTOS系列第11篇---FreeRTOS任務控制
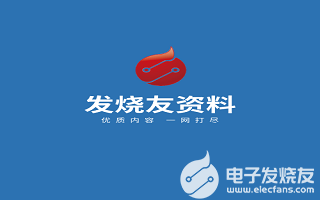
評論