# SwipeGesture
用于觸發(fā)滑動(dòng)事件,滑動(dòng)速度大于100vp/s時(shí)可識(shí)別成功。
參數(shù)名稱 | 參數(shù)類型 | 必填 | 參數(shù)描述 |
---|---|---|---|
fingers | number | 否 | 觸發(fā)滑動(dòng)的最少手指數(shù),默認(rèn)為1,最小為1指,最大為10指。 默認(rèn)值:1 |
direction | SwipeDirection | 否 | 觸發(fā)滑動(dòng)手勢(shì)的滑動(dòng)方向。 默認(rèn)值:SwipeDirection.All |
speed | number | 否 | 識(shí)別滑動(dòng)的最小速度(默認(rèn)為100VP/秒)。 默認(rèn)值:100 |
SwipeDirection的三個(gè)枚舉值
declare enum SwipeDirection {
/**
* Default.
* @since 8
*/
None,
/**
* Sliding horizontally.
* @since 8
*/
Horizontal,
/**
* Sliding Vertical
* @since 8
*/
Vertical,
/**
* Sliding in all directions.
* @since 8
*/
All
}
- All:所有方向。
- Horizontal:水平方向,手指滑動(dòng)方向與x軸夾角小于45度時(shí)觸發(fā)。
- Vertical:豎直方向,手指滑動(dòng)方向與y軸夾角小于45度時(shí)觸發(fā)。
- None:任何方向均不可觸發(fā)。
事件
* Slide gesture recognition success callback.
* @since 8
*/
onAction(event: (event?: GestureEvent) => void): SwipeGestureInterface;
完整代碼
@Entry
@Component
struct SwipeGestureExample {
@State rotateAngle: number = 0
@State speed: number = 1
?
build() {
Column() {
Column() {
Text("滑動(dòng) 速度" + this.speed)
Text("滑動(dòng) 角度" + this.rotateAngle)
}
.border({ width: 3 })
.width(300)
.height(200)
.margin(100)
.rotate({
?
?
angle: this.rotateAngle,})
// 單指豎直方向滑動(dòng)時(shí)觸發(fā)該事件
.gesture(
SwipeGesture({
fingers:2,
direction: SwipeDirection.All })
.onAction((event: GestureEvent) => {
this.speed = event.speed
this.rotateAngle = event.angle
})
)
}.width('100%')
}
}
RotationGesture
用于觸發(fā)旋轉(zhuǎn)手勢(shì)事件,觸發(fā)旋轉(zhuǎn)手勢(shì)的最少手指為2指,最大為5指,最小改變度數(shù)為1度。
數(shù)名稱 | 參數(shù)類型 | 必填 | 參數(shù)描述 |
---|---|---|---|
fingers | number | 否 | 觸發(fā)旋轉(zhuǎn)的最少手指數(shù), 最小為2指,最大為5指。 默認(rèn)值:2 |
angle | number | 否 | 觸發(fā)旋轉(zhuǎn)手勢(shì)的最小改變度數(shù),單位為deg。 默認(rèn)值:1 |
提供了四種事件
事件
- ** onActionStart(event: (event?: GestureEvent) => void):Rotation手勢(shì)識(shí)別成功回調(diào)。**
- onActionUpdate(event: (event?: GestureEvent) => void):Rotation手勢(shì)移動(dòng)過(guò)程中回調(diào)。
- ** onActionEnd(event: (event?: GestureEvent) => void):Rotation手勢(shì)識(shí)別成功,手指抬起后觸發(fā)回調(diào)。**
- ** onActionCancel(event: () => void):Rotation手勢(shì)識(shí)別成功,接收到觸摸取消事件觸發(fā)回調(diào)。**
* Pan gesture recognition success callback.
* @since 7
*/
onActionStart(event: (event?: GestureEvent) => void): RotationGestureInterface;
/**
* Callback when the Pan gesture is moving.
* @since 7
*/
onActionUpdate(event: (event?: GestureEvent) => void): RotationGestureInterface;
/**
* The Pan gesture is successfully recognized. When the finger is lifted, the callback is triggered.
* @since 7
*/
onActionEnd(event: (event?: GestureEvent) => void): RotationGestureInterface;
/**
* The Pan gesture is successfully recognized and a callback is triggered when the touch cancel event is received.
* @since 7
*/
onActionCancel(event: () => void): RotationGestureInterface;
}
完整代碼:
// xxx.ets
@Entry
@Component
struct RotationGestureExample {
@State angle: number = 0
@State rotateValue: number = 0
?
build() {
Column() {
Column() {
Text('旋轉(zhuǎn)角度:' + this.angle)
}
.height(200)
.width(300)
.padding(20)
.border({ width: 3 })
.margin(80)
.rotate({ angle: this.angle })
// 雙指旋轉(zhuǎn)觸發(fā)該手勢(shì)事件
.gesture(
RotationGesture()
.onActionStart((event: GestureEvent) => {
console.info('Rotation start')
})
.onActionUpdate((event: GestureEvent) => {
this.angle = this.rotateValue + event.angle
?
})
.onActionEnd(() => {
this.rotateValue = this.angle
console.info('Rotation end')
}).onActionCancel(()=>{
console.info('Rotation onActionCancel')
})
)
}.width('100%')
}
}
用于觸發(fā)滑動(dòng)事件,滑動(dòng)速度大于100vp/s時(shí)可識(shí)別成功。
參數(shù)名稱 | 參數(shù)類型 | 必填 | 參數(shù)描述 |
---|---|---|---|
fingers | number | 否 | 觸發(fā)滑動(dòng)的最少手指數(shù),默認(rèn)為1,最小為1指,最大為10指。 默認(rèn)值:1 |
direction | SwipeDirection | 否 | 觸發(fā)滑動(dòng)手勢(shì)的滑動(dòng)方向。 默認(rèn)值:SwipeDirection.All |
speed | number | 否 | 識(shí)別滑動(dòng)的最小速度(默認(rèn)為100VP/秒)。 默認(rèn)值:100 |
SwipeDirection的三個(gè)枚舉值
declare enum SwipeDirection {
/**
* Default.
* @since 8
*/
None,
/**
* Sliding horizontally.
* @since 8
*/
Horizontal,
/**
* Sliding Vertical
* @since 8
*/
Vertical,
/**
* Sliding in all directions.
* @since 8
*/
All
}
- All:所有方向。
- Horizontal:水平方向,手指滑動(dòng)方向與x軸夾角小于45度時(shí)觸發(fā)。
- Vertical:豎直方向,手指滑動(dòng)方向與y軸夾角小于45度時(shí)觸發(fā)。
- None:任何方向均不可觸發(fā)。
事件
* Slide gesture recognition success callback.
* @since 8
*/
onAction(event: (event?: GestureEvent) => void): SwipeGestureInterface;
完整代碼
@Entry
@Component
struct SwipeGestureExample {
@State rotateAngle: number = 0
@State speed: number = 1
?
build() {
Column() {
Column() {
Text("滑動(dòng) 速度" + this.speed)
Text("滑動(dòng) 角度" + this.rotateAngle)
}
.border({ width: 3 })
.width(300)
.height(200)
.margin(100)
.rotate({
?
?
angle: this.rotateAngle,})
// 單指豎直方向滑動(dòng)時(shí)觸發(fā)該事件
.gesture(
SwipeGesture({
fingers:2,
direction: SwipeDirection.All })
.onAction((event: GestureEvent) => {
this.speed = event.speed
this.rotateAngle = event.angle
})
)
}.width('100%')
}
}
RotationGesture
用于觸發(fā)旋轉(zhuǎn)手勢(shì)事件,觸發(fā)旋轉(zhuǎn)手勢(shì)的最少手指為2指,最大為5指,最小改變度數(shù)為1度。
數(shù)名稱 | 參數(shù)類型 | 必填 | 參數(shù)描述 |
---|---|---|---|
fingers | number | 否 | 觸發(fā)旋轉(zhuǎn)的最少手指數(shù), 最小為2指,最大為5指。 默認(rèn)值:2 |
angle | number | 否 | 觸發(fā)旋轉(zhuǎn)手勢(shì)的最小改變度數(shù),單位為deg。 默認(rèn)值:1 |
提供了四種事件
事件
- ** onActionStart(event: (event?: GestureEvent) => void):Rotation手勢(shì)識(shí)別成功回調(diào)。**
- onActionUpdate(event: (event?: GestureEvent) => void):Rotation手勢(shì)移動(dòng)過(guò)程中回調(diào)。
- ** onActionEnd(event: (event?: GestureEvent) => void):Rotation手勢(shì)識(shí)別成功,手指抬起后觸發(fā)回調(diào)。**
- ** onActionCancel(event: () => void):Rotation手勢(shì)識(shí)別成功,接收到觸摸取消事件觸發(fā)回調(diào)。**
* Pan gesture recognition success callback.
* @since 7
*/
onActionStart(event: (event?: GestureEvent) => void): RotationGestureInterface;
/**
* Callback when the Pan gesture is moving.
* @since 7
*/
onActionUpdate(event: (event?: GestureEvent) => void): RotationGestureInterface;
/**
* The Pan gesture is successfully recognized. When the finger is lifted, the callback is triggered.
* @since 7
*/
onActionEnd(event: (event?: GestureEvent) => void): RotationGestureInterface;
/**
* The Pan gesture is successfully recognized and a callback is triggered when the touch cancel event is received.
* @since 7
*/
onActionCancel(event: () => void): RotationGestureInterface;
}
完整代碼:
// xxx.ets
@Entry
@Component
struct RotationGestureExample {
@State angle: number = 0
@State rotateValue: number = 0
?
build() {
Column() {
Column() {
Text('旋轉(zhuǎn)角度:' + this.angle)
}
.height(200)
.width(300)
.padding(20)
.border({ width: 3 })
.margin(80)
.rotate({ angle: this.angle })
// 雙指旋轉(zhuǎn)觸發(fā)該手勢(shì)事件
.gesture(
RotationGesture()
.onActionStart((event: GestureEvent) => {
console.info('Rotation start')
})
.onActionUpdate((event: GestureEvent) => {
this.angle = this.rotateValue + event.angle
?
})
.onActionEnd(() => {
this.rotateValue = this.angle
console.info('Rotation end')
}).onActionCancel(()=>{
console.info('Rotation onActionCancel')
})
)
}.width('100%')
}
}
-
手勢(shì)識(shí)別
+關(guān)注
關(guān)注
8文章
225瀏覽量
47786 -
觸摸
+關(guān)注
關(guān)注
7文章
198瀏覽量
64211 -
OpenHarmony
+關(guān)注
關(guān)注
25文章
3713瀏覽量
16254 -
OpenHarmony3.1
+關(guān)注
關(guān)注
0文章
11瀏覽量
622
發(fā)布評(píng)論請(qǐng)先 登錄
相關(guān)推薦
紅外手勢(shì)識(shí)別方案 紅外手勢(shì)感應(yīng)模塊 紅外識(shí)別紅外手勢(shì)識(shí)別
Android 手勢(shì)識(shí)別
使用SensorTile識(shí)別手勢(shì)
手勢(shì)識(shí)別控制器制作
HarmonyOS應(yīng)用API手勢(shì)方法-綁定手勢(shì)方法
HarmonyOS應(yīng)用API手勢(shì)方法-RotationGesture
HarmonyOS應(yīng)用API手勢(shì)方法-RotationGesture
HarmonyOS應(yīng)用API手勢(shì)方法-SwipeGesture
HarmonyOS/OpenHarmony(Stage模型)應(yīng)用開(kāi)發(fā)單一手勢(shì)(三)
HarmonyOS/OpenHarmony(Stage模型)應(yīng)用開(kāi)發(fā)組合手勢(shì)(三)互斥識(shí)別
基于加鎖機(jī)制的靜態(tài)手勢(shì)識(shí)別運(yùn)動(dòng)中的手勢(shì)
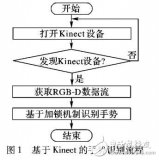
混合交互手勢(shì)模型設(shè)計(jì)
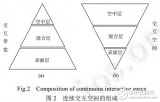
手勢(shì)識(shí)別技術(shù)及其應(yīng)用
車(chē)載手勢(shì)識(shí)別技術(shù)的原理及其應(yīng)用
鴻蒙ArkTS聲明式開(kāi)發(fā):跨平臺(tái)支持列表RotationGesture之基礎(chǔ)手勢(shì)
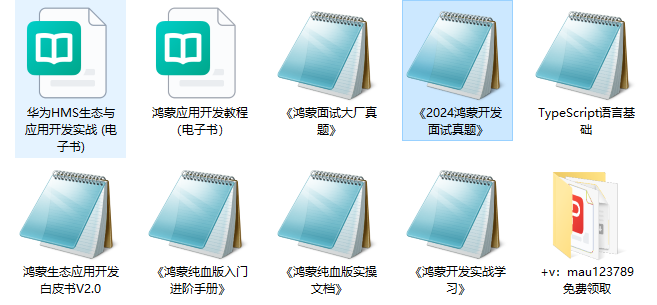
評(píng)論