介紹:
在電影《鋼鐵俠》中,我們看到托尼·斯塔克在建造設備時與人工智能賈維斯交流。托尼向賈維斯描述了他需要的零件,賈維斯控制機械臂協助托尼完成任務。隨著當今技術的發展,這種實現只是時間問題。因此,我決定嘗試自己實現這個功能,用語音控制來操作機械臂,實現人工智能的簡單應用。
我全權負責連接和控制機械臂和語音開發板,通過特定命令觸發機械臂的運動。這是一個具有挑戰性的項目,因為語音控制技術涉及多個方面,如語音識別、信號處理和機器人控制,需要我學習和理解相關知識。通過這篇文章,我希望分享我的項目實施過程、成就和經驗教訓,以激勵和幫助在機器人和語音控制領域工作的同學。
為了實施這個項目,我首先需要解決兩個主要問題:如何處理語音信號以及如何控制機械臂。
處理語音信號:
處理語音信號的步驟:
● 硬件選擇:選擇合適的開發板(Arduino、樹莓派等)。
● 麥克風信號處理:選擇合適的麥克風進行采集,通過模數轉換器將采集到的語音信號轉換為數字信號。
● 信號處理:采集到的信號通過降噪、濾波等方式進行處理。提取有用的特征,并使用語音識別算法處理特征信號,以將數字信號輸出到計算機。
控制機械臂:
● 使用簡單易操作的機械臂。
以上是解決兩個主要問題的方法。然而,經過研究,開發可以處理語音信號的開發板需要大量的工作!幸運的是,現在市場上有集成語音開發板,我們可以直接購買一個來使用。
HLK-V20 語音開發板
HLK-V20 開發板是一個具有兩個 IO 接口的復雜板 - 一個用于語音輸出,另一個用于語音輸入。它可以通過串行端口連接到計算機。
MyCobot 280 M5Stack 2023 機械臂
對于機械臂,我選擇了流行的桌面機械臂,myCobot 280 M5Stack 2023,由大象機器人公司開發。該機械臂最近進行了重大的軟件更新,實現了20ms的串行通信速度,并提供與Python和C++等流行編程語言兼容的豐富接口。我選擇這個機械臂有幾個原因,包括它緊湊的尺寸、豐富的 Python API 接口(我擅長編程)以及易于安裝和使用。
編程語言: Python 3.7+
平臺: windows11
使用的Python庫:serial,pymycobot,time。
項目的發展
在項目的開發中,涉及四個主要步驟:
● 語音輸入
● 開發板檢索語音信息并傳輸到PC
● PC處理接收到的信息
● 向機械臂發送運動控制命令以進行運動
為了觀察開發板如何傳輸數據以及返回的數據類型,使用Python中的串行庫來操作串行端口。該庫具有從串行端口打開、關閉、讀取和寫入數據的方法。
'''
For instance, the serial.Serial() method is used to open the serial port and connect it to the PC, while the serial.read() method reads the data transmitted from the serial port.
'''
import serial
s = serial.Serial("COM9",115200)
r = s.read()
while True:
print(r)
復制
這是從 read() 獲取的數據。
通過分析從開發板傳輸的數據,開發板的喚醒詞被識別為“wakeup_uni”,而關鍵字“uArTcp”表示下一個命令的開始。
發現接收到的數據連接在一起,每次輸入命令時,都會與前一個命令連接在一起。
發現接收到的數據連接在一起,每次輸入命令時,都會與前一個命令連接在一起。為了檢查開發板是否已收到語音輸入,使用了 if a in b: 語句,并預先準備了輸入命令及其相應的關鍵字。例如,喚醒詞設置為“wakeup_uni”,并設置了 4 個提示詞以進行測試。
#set prompt word
hi_mycobot = b'openled'
dancing = b'zengjialiangdu'
nod_head = b'jianxiaoliangdu'
go_back = b'closeled'
# run frame
while True:
r += s.read()
if wake_up in r:
print("wake")
wake = True
r = b''
if wake and hi_mycobot in r:
print("Hi myCobot")
r = b''
if wake and dancing in r:
print("dancing")
r = b''
if wake and nod_head in r:
print('nod your head')
r = b''
if wake and go_back in r:
print('Go back')
r = b''
if wake and _exit in r:
print("exit")
r = b''
# print(r)s
復制
這是測試結果
pymycobot庫是由Elephant Robotics開發的機械臂API調用接口庫。具體的使用方法在Gitbook(由Elephant Robotics提供)上。
# import library
from pymycobot import Mycobot
import time
# Create an instance object for later use
mc = Mycobot('COM9',115200)
#Control the mechanical arm to move at a speed of 70 according to the angle in the list,send_angles([angles],speed)
mc.send_angles([0.87,(-50.44),47.28,0.35,(-0.43),(-0.26)],70)
# Execute the next command after a delay of x seconds (the movement of the robotic arm takes time)
time.sleep(x)
復制
接下來,將代碼的兩個部分組合在一起。
import serial
from pymycobot import MyCobot
import time
s = serial.Serial("COM6",115200)
mc = MyCobot('COM3',115200)
r = b''
wake_up = b'wakeup_uni'
_exit = b'exitUni'
hi_mycobot = b'openled'
dancing = b'zengjialiangdu'
nod_head = b'jianxiaoliangdu'
go_back = b'closeled'
wake = False
while True:
r += s.read()
if wake_up in r:
print("wake")
wake = True
r = b''
if wake and hi_mycobot in r:
print("Hi myCobot")
# say hi shake with you
mc.send_angles([0.87,(-50.44),47.28,0.35,(-0.43),(-0.26)],70)
time.sleep(1)
for count in range(3):
mc.send_angle(1,30,80)
time.sleep(1)
mc.send_angle(1,(-30),80)
time.sleep(1)
mc.send_angles([0,0,0,0,0,0],70)
r = b''
if wake and dancing in r:
print("dancing")
mc.send_angles([0,0,0,0,0,0],80)
time.sleep(1)
for count in range(2):
mc.send_angles([(-0.17),(-94.3),118.91,(-39.9),59.32,(-0.52)],80)
time.sleep(1.2)
mc.send_angles([67.85,(-3.42),(-116.98),106.52,23.11,(-0.52)],80)
time.sleep(1.7)
mc.send_angles([(-38.14),(-115.04),116.63,69.69,3.25,(-11.6)],80)
time.sleep(1.7)
mc.send_angles([2.72,(-26.19),140.27,(-110.74),(-6.15),(-11.25)],80)
time.sleep(1)
mc.send_angles([0,0,0,0,0,0],80)
r = b''
if wake and nod_head in r:
print('nod your head')
mc.send_angles([0,0,0,0,0,0],70)
time.sleep(1)
mc.send_angles([3.07,(-86.3),75.32,11.86,2.72,(-0.35)],70)
time.sleep(0.5)
for count in range(4):
mc.send_angle(4,13,70)
time.sleep(0.5)
mc.send_angle(4,(-60),70)
time.sleep(1)
mc.send_angle(4,13,70)
time.sleep(0.5)
mc.send_angles([0,0,0,0,0,0],70)
r = b''
if wake and go_back in r:
print('Go back')
mc.send_angles([12.83,(-138.95),156.09,(-12.3),(-12.91),35.41],70)
r = b''
if wake and _exit in r:
print("exit")
r = b''
三. 經驗和教訓
在完成這個項目的過程中,我收獲了很多寶貴的經驗和見解。首先,我意識到一個項目的完成不僅取決于對技術的掌握,還需要事先的研究和理解。當我在研究語音識別開發板的制作時,我發現工作量非常大,實現起來會非常困難。但是,市場上已經有許多成熟的技術和工具。我們只需要選擇合理的組合和整合。其次,項目的圓滿完成需要明確的框架和充分的準備。在這個項目中,我需要了解語音識別算法、機械臂設計和控制技術,掌握硬件設備和軟件開發工具的使用方法和性能特點。
在這個項目中,我成功地將語音識別技術與機械臂控制技術相結合,實現了機械臂的語音控制。雖然這個項目的規模相對較小,但它代表了人工智能技術在現實生活中的應用和發展趨勢。雖然這只是一個人工智能項目的原型,但它的完成對我來說是一次寶貴的經驗。在以后的研究中,我將繼續探索更多相關信息,以進一步完善這個項目。如果您有任何好的想法,請在下面發表評論。
審核編輯 黃宇
-
語音控制
+關注
關注
5文章
484瀏覽量
28268 -
機械臂
+關注
關注
12文章
515瀏覽量
24634
發布評論請先 登錄
相關推薦
【原創】 drawbot 平面機械臂scara寫字畫畫機器人DIY教程貼
奧比中光推出2.0版大模型機械臂
大象機器人開源協作機械臂機械臂接入GPT4o大模型!
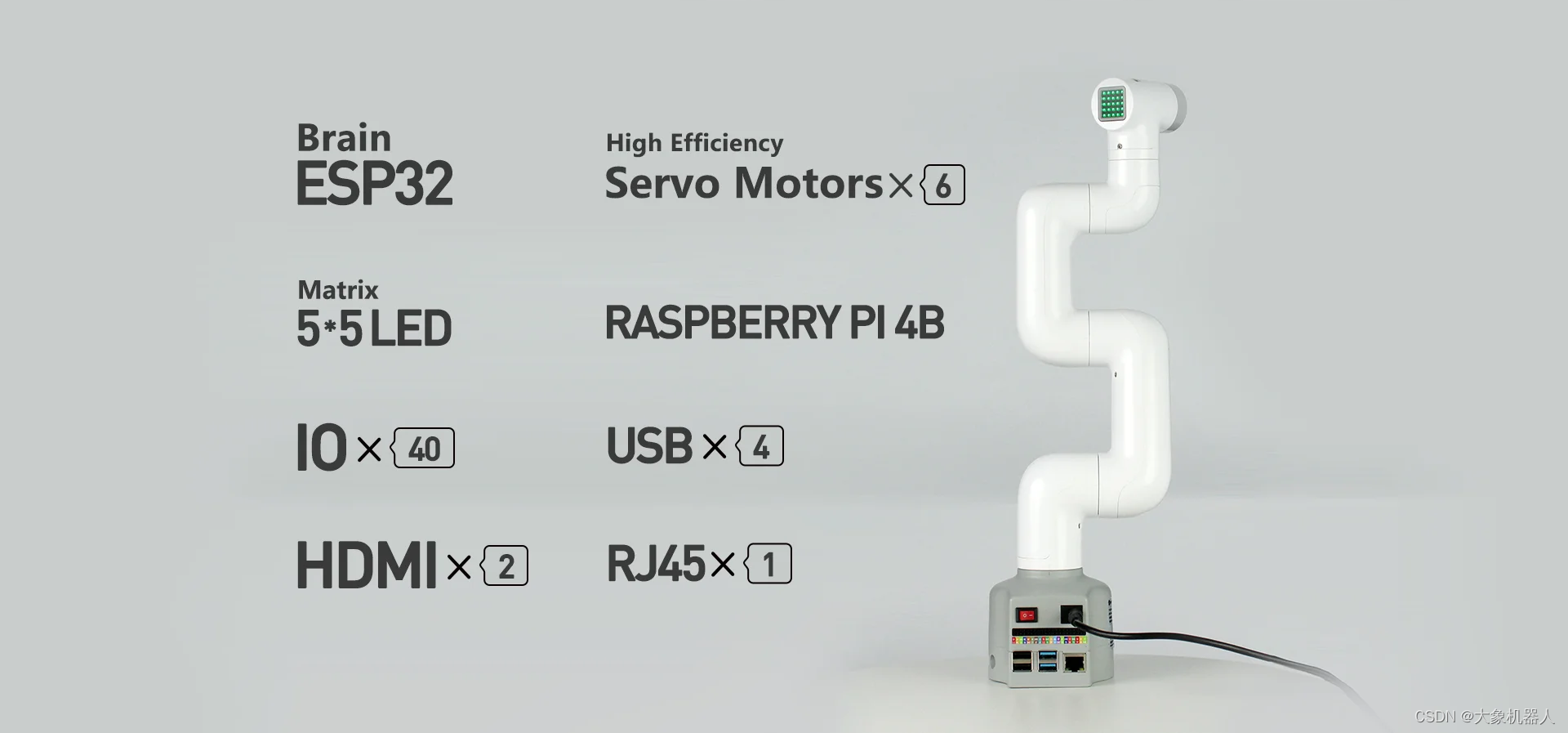
國產Cortex-A55人工智能教學實驗箱_基于Python機械臂跳舞實驗案例分享
基于六維力傳感器的機械臂自動裝配應用
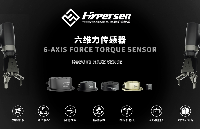
stm32f0怎么不使用語音IC做合成語音?
自然語言控制機械臂:ChatGPT與機器人技術的融合創新(下)
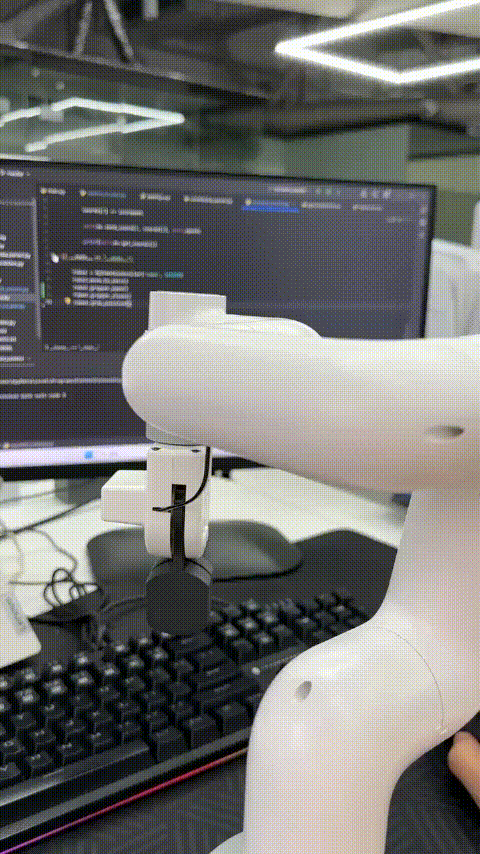
評論