在過去的十年中,深度學習見證了某種形式的寒武紀大爆發(fā)。技術、應用和算法的絕對數量遠遠超過了前幾十年的進步。這是由于多種因素的偶然組合,其中之一是許多開源深度學習框架提供的強大的免費工具。Theano (Bergstra等人,2010 年)、DistBelief (Dean等人,2012 年)和 Caffe (Jia等人,2014 年)可以說代表了被廣泛采用的第一代此類模型。與 SN2 (Simulateur Neuristique) 等早期(開創(chuàng)性)作品相比 (Bottou 和 Le Cun,1988),它提供了類似 Lisp 的編程體驗,現代框架提供了自動微分和 Python 的便利性。這些框架使我們能夠自動化和模塊化實現基于梯度的學習算法的重復性工作。
在3.4 節(jié)中,我們僅依靠 (i) 張量進行數據存儲和線性代數;(ii) 計算梯度的自動微分。在實踐中,由于數據迭代器、損失函數、優(yōu)化器和神經網絡層非常普遍,現代圖書館也為我們實現了這些組件。在本節(jié)中,我們將向您展示如何 使用深度學習框架的高級 API 簡潔地實現3.4 節(jié)中的線性回歸模型。
import numpy as np import torch from torch import nn from d2l import torch as d2l
from mxnet import autograd, gluon, init, np, npx from mxnet.gluon import nn from d2l import mxnet as d2l npx.set_np()
import jax import optax from flax import linen as nn from jax import numpy as jnp from d2l import jax as d2l
No GPU/TPU found, falling back to CPU. (Set TF_CPP_MIN_LOG_LEVEL=0 and rerun for more info.)
import numpy as np import tensorflow as tf from d2l import tensorflow as d2l
3.5.1. 定義模型
當我們在第 3.4 節(jié)中從頭開始實現線性回歸時 ,我們明確定義了我們的模型參數并編寫了計算代碼以使用基本線性代數運算生成輸出。你應該知道如何做到這一點。但是一旦您的模型變得更加復雜,并且一旦您幾乎每天都必須這樣做,您就會很高興獲得幫助。這種情況類似于從頭開始編寫自己的博客。做一兩次是有益和有益的,但如果你花一個月重新發(fā)明輪子,你將成為一個糟糕的 Web 開發(fā)人員。
對于標準操作,我們可以使用框架的預定義層,這使我們能夠專注于用于構建模型的層,而不用擔心它們的實現。回想一下圖 3.1.2中描述的單層網絡的架構。該層稱為全連接層,因為它的每個輸入都通過矩陣向量乘法連接到它的每個輸出。
在 PyTorch 中,全連接層定義在Linear和 LazyLinear(自版本 1.8.0 起可用)類中。后者允許用戶僅指定輸出維度,而前者額外詢問有多少輸入進入該層。指定輸入形狀很不方便,這可能需要大量的計算(例如在卷積層中)。因此,為簡單起見,我們將盡可能使用此類“惰性”層。
class LinearRegression(d2l.Module): #@save """The linear regression model implemented with high-level APIs.""" def __init__(self, lr): super().__init__() self.save_hyperparameters() self.net = nn.LazyLinear(1) self.net.weight.data.normal_(0, 0.01) self.net.bias.data.fill_(0)
In Gluon, the fully connected layer is defined in the Dense class. Since we only want to generate a single scalar output, we set that number to 1. It is worth noting that, for convenience, Gluon does not require us to specify the input shape for each layer. Hence we do not need to tell Gluon how many inputs go into this linear layer. When we first pass data through our model, e.g., when we execute net(X) later, Gluon will automatically infer the number of inputs to each layer and thus instantiate the correct model. We will describe how this works in more detail later.
class LinearRegression(d2l.Module): #@save """The linear regression model implemented with high-level APIs.""" def __init__(self, lr): super().__init__() self.save_hyperparameters() self.net = nn.Dense(1) self.net.initialize(init.Normal(sigma=0.01))
class LinearRegression(d2l.Module): #@save """The linear regression model implemented with high-level APIs.""" lr: float def setup(self): self.net = nn.Dense(1, kernel_init=nn.initializers.normal(0.01))
In Keras, the fully connected layer is defined in the Dense class. Since we only want to generate a single scalar output, we set that number to 1. It is worth noting that, for convenience, Keras does not require us to specify the input shape for each layer. We do not need to tell Keras how many inputs go into this linear layer. When we first try to pass data through our model, e.g., when we execute net(X) later, Keras will automatically infer the number of inputs to each layer. We will describe how this works in more detail later.
class LinearRegression(d2l.Module): #@save """The linear regression model implemented with high-level APIs.""" def __init__(self, lr): super().__init__() self.save_hyperparameters() initializer = tf.initializers.RandomNormal(stddev=0.01) self.net = tf.keras.layers.Dense(1, kernel_initializer=initializer)
在forward方法中,我們只調用預定義層的內置__call__ 方法來計算輸出。
@d2l.add_to_class(LinearRegression) #@save def forward(self, X): return self.net(X)
@d2l.add_to_class(LinearRegression) #@save def forward(self, X): return self.net(X)
@d2l.add_to_class(LinearRegression) #@save def forward(self, X): return self.net(X)
@d2l.add_to_class(LinearRegression) #@save def forward(self, X): return self.net(X)
3.5.2. 定義損失函數
該類MSELoss計算均方誤差(沒有 1/2(3.1.5)中的因素)。默認情況下,MSELoss 返回示例的平均損失。它比我們自己實現更快(也更容易使用)。
@d2l.add_to_class(LinearRegression) #@save def loss(self, y_hat, y): fn = nn.MSELoss() return fn(y_hat, y)
The loss module defines many useful loss functions. For speed and convenience, we forgo implementing our own and choose the built-in loss.L2Loss instead. Because the loss that it returns is the squared error for each example, we use meanto average the loss across over the minibatch.
@d2l.add_to_class(LinearRegression) #@save def loss(self, y_hat, y): fn = gluon.loss.L2Loss() return fn(y_hat, y).mean()
@d2l.add_to_class(LinearRegression) #@save def loss(self, params, X, y, state): y_hat = state.apply_fn({'params': params}, *X) return optax.l2_loss(y_hat, y).mean()
The MeanSquaredError class computes the mean squared error (without the 1/2 factor in (3.1.5)). By default, it returns the average loss over examples.
@d2l.add_to_class(LinearRegression) #@save def loss(self, y_hat, y): fn = tf.keras.losses.MeanSquaredError() return fn(y, y_hat)
3.5.3. 定義優(yōu)化算法
Minibatch SGD 是用于優(yōu)化神經網絡的標準工具,因此 PyTorch 支持它以及模塊中該算法的許多變體optim。當我們實例化一個SGD實例時,我們指定要優(yōu)化的參數,可通過 和我們的優(yōu)化算法所需的self.parameters()學習率 ( ) 從我們的模型中獲得。self.lr
@d2l.add_to_class(LinearRegression) #@save def configure_optimizers(self): return torch.optim.SGD(self.parameters(), self.lr)
Minibatch SGD is a standard tool for optimizing neural networks and thus Gluon supports it alongside a number of variations on this algorithm through its Trainer class. Note that Gluon’s Trainer class stands for the optimization algorithm, while the Trainer class we created in Section 3.2 contains the training method, i.e., repeatedly call the optimizer to update the model parameters. When we instantiate Trainer, we specify the parameters to optimize over, obtainable from our model net via net.collect_params(), the optimization algorithm we wish to use (sgd), and a dictionary of hyperparameters required by our optimization algorithm.
@d2l.add_to_class(LinearRegression) #@save def configure_optimizers(self): return gluon.Trainer(self.collect_params(), 'sgd', {'learning_rate': self.lr})
@d2l.add_to_class(LinearRegression) #@save def configure_optimizers(self): return optax.sgd(self.lr)
Minibatch SGD is a standard tool for optimizing neural networks and thus Keras supports it alongside a number of variations on this algorithm in the optimizers module.
@d2l.add_to_class(LinearRegression) #@save def configure_optimizers(self): return tf.keras.optimizers.SGD(self.lr)
3.5.4. 訓練
您可能已經注意到,通過深度學習框架的高級 API 表達我們的模型需要更少的代碼行。我們不必單獨分配參數、定義損失函數或實施小批量 SGD。一旦我們開始處理更復雜的模型,高級 API 的優(yōu)勢就會顯著增加。現在我們已經準備好所有基本部分,訓練循環(huán)本身與我們從頭開始實施的循環(huán)相同。所以我們只需要調用 依賴于3.4節(jié)方法 實現的方法( 3.2.4fit節(jié)介紹)來訓練我們的模型。fit_epoch
model = LinearRegression(lr=0.03) data = d2l.SyntheticRegressionData(w=torch.tensor([2, -3.4]), b=4.2) trainer = d2l.Trainer(max_epochs=3) trainer.fit(model, data)
model = LinearRegression(lr=0.03) data = d2l.SyntheticRegressionData(w=np.array([2, -3.4]), b=4.2) trainer = d2l.Trainer(max_epochs=3) trainer.fit(model, data)
model = LinearRegression(lr=0.03) data = d2l.SyntheticRegressionData(w=jnp.array([2, -3.4]), b=4.2) trainer = d2l.Trainer(max_epochs=3) trainer.fit(model, data)
model = LinearRegression(lr=0.03) data = d2l.SyntheticRegressionData(w=tf.constant([2, -3.4]), b=4.2) trainer = d2l.Trainer(max_epochs=3) trainer.fit(model, data)
下面,我們將通過有限數據訓練學習到的模型參數與生成數據集的實際參數進行比較。為了訪問參數,我們訪問了我們需要的層的權重和偏差。正如我們從頭開始實施一樣,請注意我們估計的參數接近于它們的真實對應物。
@d2l.add_to_class(LinearRegression) #@save def get_w_b(self): return (self.net.weight.data, self.net.bias.data) w, b = model.get_w_b() print(f'error in estimating w: {data.w - w.reshape(data.w.shape)}') print(f'error in estimating b: {data.b - b}')
error in estimating w: tensor([ 0.0022, -0.0069]) error in estimating b: tensor([0.0080])
@d2l.add_to_class(LinearRegression) #@save def get_w_b(self): return (self.net.weight.data(), self.net.bias.data()) w, b = model.get_w_b()
@d2l.add_to_class(LinearRegression) #@save def get_w_b(self, state): net = state.params['net'] return net['kernel'], net['bias'] w, b = model.get_w_b(trainer.state)
@d2l.add_to_class(LinearRegression) #@save def get_w_b(self): return (self.get_weights()[0], self.get_weights()[1]) w, b = model.get_w_b()
3.5.5. 概括
本節(jié)包含深度網絡(在本書中)的第一個實現,以利用現代深度學習框架提供的便利,例如 MXNet (Chen等人,2015 年)、JAX (Frostig等人,2018 年)、PyTorch (Paszke等人,2019 年)和 Tensorflow (Abadi等人,2016 年). 我們使用框架默認值來加載數據、定義層、損失函數、優(yōu)化器和訓練循環(huán)。每當框架提供所有必要的功能時,使用它們通常是個好主意,因為這些組件的庫實現往往會針對性能進行大量優(yōu)化,并針對可靠性進行適當測試。同時,盡量不要忘記這些模塊是可以直接實現的。這對于希望生活在模型開發(fā)前沿的有抱負的研究人員尤其重要,您將在其中發(fā)明任何當前庫中不可能存在的新組件。
在PyTorch中,該data模塊提供了數據處理的工具,該 nn模塊定義了大量的神經網絡層和常見的損失函數。我們可以通過用以結尾的方法替換它們的值來初始化參數_。請注意,我們需要指定網絡的輸入維度。雖然這目前微不足道,但當我們想要設計具有多層的復雜網絡時,它可能會產生重大的連鎖反應。需要仔細考慮如何參數化這些網絡以實現可移植性。
In Gluon, the data module provides tools for data processing, the nn module defines a large number of neural network layers, and the loss module defines many common loss functions. Moreover, the initializer gives access to many choices for parameter initialization. Conveniently for the user, dimensionality and storage are automatically inferred. A consequence of this lazy initialization is that you must not attempt to access parameters before they have been instantiated (and initialized).
In TensorFlow, the data module provides tools for data processing, the keras module defines a large number of neural network layers and common loss functions. Moreover, the initializers module provides various methods for model parameter initialization. Dimensionality and storage for networks are automatically inferred (but be careful not to attempt to access parameters before they have been initialized).
3.5.6. 練習
如果將小批量的總損失替換為小批量損失的平均值,您需要如何更改學習率?
查看框架文檔以查看提供了哪些損失函數。特別是,用 Huber 的穩(wěn)健損失函數替換平方損失。即使用損失函數
(3.5.1)l(y,y′)={|y?y′|?σ2if|y?y′|>σ12σ(y?y′)2otherwise
您如何訪問模型權重的梯度?
如果改變學習率和迭代次數,解決方案會如何變化?它是否不斷改進?
當您更改生成的數據量時,解決方案如何變化?
繪制估計誤差 w^?w和b^?b作為數據量的函數。提示:以對數方式而不是線性方式增加數據量,即 5、10、20、50、...、10,000 而不是 1,000、2,000、...、10,000。
為什么提示中的建議是合適的?
-
pytorch
+關注
關注
2文章
808瀏覽量
13250
發(fā)布評論請先 登錄
相關推薦
TensorFlow實現簡單線性回歸
TensorFlow實現多元線性回歸(超詳細)
使用PyMC3包實現貝葉斯線性回歸
PyTorch教程3.4之從頭開始執(zhí)行線性回歸
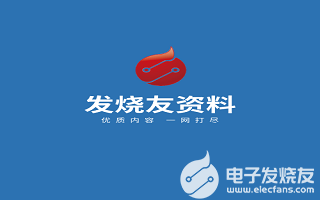
PyTorch教程4.4之從頭開始實現Softmax回歸
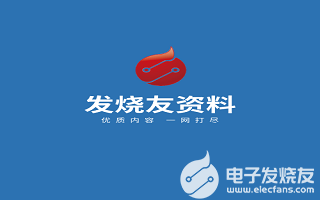
PyTorch教程9.6之遞歸神經網絡的簡潔實現
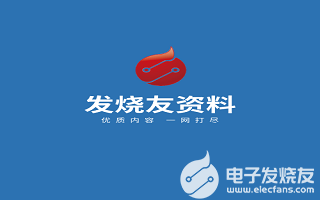
PyTorch教程13.6之多個GPU的簡潔實現
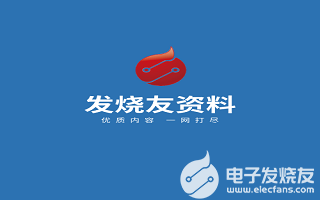
評論