在前面的章節中,我們討論了如何在只有 60000 張圖像的 Fashion-MNIST 訓練數據集上訓練模型。我們還描述了 ImageNet,這是學術界使用最廣泛的大規模圖像數據集,它有超過 1000 萬張圖像和 1000 個對象。然而,我們通常遇到的數據集的大小介于這兩個數據集之間。
假設我們要從圖片中識別出不同類型的椅子,然后向用戶推薦購買鏈接。一種可能的方法是首先識別 100 把普通椅子,為每把椅子拍攝 1000 張不同角度的圖像,然后在收集的圖像數據集上訓練分類模型。雖然這個椅子數據集可能比 Fashion-MNIST 數據集大,但示例數量仍然不到 ImageNet 中的十分之一。這可能會導致適用于 ImageNet 的復雜模型在此椅子數據集上過度擬合。此外,由于訓練示例數量有限,訓練模型的準確性可能無法滿足實際要求。
為了解決上述問題,一個顯而易見的解決方案是收集更多的數據。然而,收集和標記數據可能會花費大量時間和金錢。例如,為了收集 ImageNet 數據集,研究人員花費了數百萬美元的研究經費。雖然目前的數據采集成本已經大幅降低,但這一成本仍然不容忽視。
另一種解決方案是應用遷移學習,將從源數據集中學到的知識遷移到目標數據集中。例如,盡管 ImageNet 數據集中的大部分圖像與椅子無關,但在此數據集上訓練的模型可能會提取出更一般的圖像特征,這有助于識別邊緣、紋理、形狀和物體組成。這些相似的特征也可能對識別椅子有效。
14.2.1。腳步
在本節中,我們將介紹遷移學習中的一種常用技術:微調。如圖 14.2.1所示,微調包括以下四個步驟:
在源數據集(例如,ImageNet 數據集)上預訓練神經網絡模型,即源模型。
創建一個新的神經網絡模型,即目標模型。這將復制源模型上除輸出層之外的所有模型設計及其參數。我們假設這些模型參數包含從源數據集中學到的知識,并且這些知識也適用于目標數據集。我們還假設源模型的輸出層與源數據集的標簽密切相關;因此它不在目標模型中使用。
向目標模型添加一個輸出層,其輸出數量為目標數據集中的類別數量。然后隨機初始化該層的模型參數。
在目標數據集(例如椅子數據集)上訓練目標模型。輸出層將從頭開始訓練,而所有其他層的參數將根據源模型的參數進行微調。
圖 14.2.1微調。
當目標數據集遠小于源數據集時,微調有助于提高模型的泛化能力。
14.2.2。熱狗識別
讓我們通過一個具體案例來演示微調:熱狗識別。我們將在一個小型數據集上微調 ResNet 模型,該數據集是在 ImageNet 數據集上預訓練的。這個小數據集包含數千張有熱狗和沒有熱狗的圖像。我們將使用微調模型從圖像中識別熱狗。
%matplotlib inline import os import torch import torchvision from torch import nn from d2l import torch as d2l
%matplotlib inline import os from mxnet import gluon, init, np, npx from mxnet.gluon import nn from d2l import mxnet as d2l npx.set_np()
14.2.2.1。讀取數據集
我們使用的熱狗數據集取自在線圖像。該數據集包含 1400 張包含熱狗的正類圖像,以及包含其他食物的大量負類圖像。兩個類別的 1000 張圖像用于訓練,其余用于測試。
解壓下載的數據集后,我們得到兩個文件夾 hotdog/train和hotdog/test. 這兩個文件夾都有hotdog和 not-hotdog子文件夾,其中任何一個都包含相應類別的圖像。
#@save d2l.DATA_HUB['hotdog'] = (d2l.DATA_URL + 'hotdog.zip', 'fba480ffa8aa7e0febbb511d181409f899b9baa5') data_dir = d2l.download_extract('hotdog')
#@save d2l.DATA_HUB['hotdog'] = (d2l.DATA_URL + 'hotdog.zip', 'fba480ffa8aa7e0febbb511d181409f899b9baa5') data_dir = d2l.download_extract('hotdog')
Downloading ../data/hotdog.zip from http://d2l-data.s3-accelerate.amazonaws.com/hotdog.zip...
我們創建兩個實例來分別讀取訓練和測試數據集中的所有圖像文件。
train_imgs = torchvision.datasets.ImageFolder(os.path.join(data_dir, 'train')) test_imgs = torchvision.datasets.ImageFolder(os.path.join(data_dir, 'test'))
train_imgs = gluon.data.vision.ImageFolderDataset( os.path.join(data_dir, 'train')) test_imgs = gluon.data.vision.ImageFolderDataset( os.path.join(data_dir, 'test'))
前 8 個正面示例和最后 8 個負面圖像如下所示。如您所見,圖像的大小和縱橫比各不相同。
hotdogs = [train_imgs[i][0] for i in range(8)] not_hotdogs = [train_imgs[-i - 1][0] for i in range(8)] d2l.show_images(hotdogs + not_hotdogs, 2, 8, scale=1.4);
hotdogs = [train_imgs[i][0] for i in range(8)] not_hotdogs = [train_imgs[-i - 1][0] for i in range(8)] d2l.show_images(hotdogs + not_hotdogs, 2, 8, scale=1.4);
在訓練過程中,我們首先從圖像中裁剪出一個隨機大小和隨機縱橫比的隨機區域,然后將這個區域縮放到 224×224輸入圖像。在測試過程中,我們將圖像的高度和寬度都縮放到 256 像素,然后裁剪一個中心 224×224區域作為輸入。此外,對于三個 RGB(紅色、綠色和藍色)顏色通道,我們逐通道標準化它們的值。具體來說,就是用該通道的每個值減去該通道的平均值,然后除以該通道的標準差。
# Specify the means and standard deviations of the three RGB channels to # standardize each channel normalize = torchvision.transforms.Normalize( [0.485, 0.456, 0.406], [0.229, 0.224, 0.225]) train_augs = torchvision.transforms.Compose([ torchvision.transforms.RandomResizedCrop(224), torchvision.transforms.RandomHorizontalFlip(), torchvision.transforms.ToTensor(), normalize]) test_augs = torchvision.transforms.Compose([ torchvision.transforms.Resize([256, 256]), torchvision.transforms.CenterCrop(224), torchvision.transforms.ToTensor(), normalize])
# Specify the means and standard deviations of the three RGB channels to # standardize each channel normalize = gluon.data.vision.transforms.Normalize( [0.485, 0.456, 0.406], [0.229, 0.224, 0.225]) train_augs = gluon.data.vision.transforms.Compose([ gluon.data.vision.transforms.RandomResizedCrop(224), gluon.data.vision.transforms.RandomFlipLeftRight(), gluon.data.vision.transforms.ToTensor(), normalize]) test_augs = gluon.data.vision.transforms.Compose([ gluon.data.vision.transforms.Resize(256), gluon.data.vision.transforms.CenterCrop(224), gluon.data.vision.transforms.ToTensor(), normalize])
14.2.2.2。定義和初始化模型
我們使用在 ImageNet 數據集上預訓練的 ResNet-18 作為源模型。在這里,我們指定pretrained=True自動下載預訓練模型參數。如果是第一次使用這個模型,需要連接互聯網才能下載。
pretrained_net = torchvision.models.resnet18(pretrained=True)
預訓練源模型實例包含多個特征層和一個輸出層fc。這種劃分的主要目的是為了便于微調除輸出層之外的所有層的模型參數。fc下面給出源模型的成員變量。
pretrained_net.fc
Linear(in_features=512, out_features=1000, bias=True)
pretrained_net = gluon.model_zoo.vision.resnet18_v2(pretrained=True)
The pretrained source model instance contains two member variables: features and output. The former contains all layers of the model except the output layer, and the latter is the output layer of the model. The main purpose of this division is to facilitate the fine-tuning of model parameters of all layers but the output layer. The member variable output of source model is shown below.
pretrained_net.output
Dense(512 -> 1000, linear)
作為全連接層,它將 ResNet 最終的全局平均池化輸出轉化為 ImageNet 數據集的 1000 類輸出。然后我們構建一個新的神經網絡作為目標模型。它的定義方式與預訓練源模型相同,不同之處在于它在最后一層的輸出數量設置為目標數據集中的類數(而不是 1000)。
在下面的代碼中,目標模型實例的輸出層之前的模型參數finetune_net被初始化為源模型中相應層的模型參數。由于這些模型參數是通過在 ImageNet 上進行預訓練獲得的,因此它們是有效的。因此,我們只能使用較小的學習率來 微調此類預訓練參數。相比之下,輸出層中的模型參數是隨機初始化的,通常需要更大的學習率才能從頭開始學習。讓基礎學習率成為η, 學習率10η將用于迭代輸出層中的模型參數。
finetune_net = torchvision.models.resnet18(pretrained=True) finetune_net.fc = nn.Linear(finetune_net.fc.in_features, 2) nn.init.xavier_uniform_(finetune_net.fc.weight);
finetune_net = gluon.model_zoo.vision.resnet18_v2(classes=2) finetune_net.features = pretrained_net.features finetune_net.output.initialize(init.Xavier()) # The model parameters in the output layer will be iterated using a learning # rate ten times greater finetune_net.output.collect_params().setattr('lr_mult', 10)
14.2.2.3。微調模型
首先,我們定義了一個train_fine_tuning使用微調的訓練函數,因此它可以被多次調用。
# If `param_group=True`, the model parameters in the output layer will be # updated using a learning rate ten times greater def train_fine_tuning(net, learning_rate, batch_size=128, num_epochs=5, param_group=True): train_iter = torch.utils.data.DataLoader(torchvision.datasets.ImageFolder( os.path.join(data_dir, 'train'), transform=train_augs), batch_size=batch_size, shuffle=True) test_iter = torch.utils.data.DataLoader(torchvision.datasets.ImageFolder( os.path.join(data_dir, 'test'), transform=test_augs), batch_size=batch_size) devices = d2l.try_all_gpus() loss = nn.CrossEntropyLoss(reduction="none") if param_group: params_1x = [param for name, param in net.named_parameters() if name not in ["fc.weight", "fc.bias"]] trainer = torch.optim.SGD([{'params': params_1x}, {'params': net.fc.parameters(), 'lr': learning_rate * 10}], lr=learning_rate, weight_decay=0.001) else: trainer = torch.optim.SGD(net.parameters(), lr=learning_rate, weight_decay=0.001) d2l.train_ch13(net, train_iter, test_iter, loss, trainer, num_epochs, devices)
def train_fine_tuning(net, learning_rate, batch_size=128, num_epochs=5): train_iter = gluon.data.DataLoader( train_imgs.transform_first(train_augs), batch_size, shuffle=True) test_iter = gluon.data.DataLoader( test_imgs.transform_first(test_augs), batch_size) devices = d2l.try_all_gpus() net.collect_params().reset_ctx(devices) net.hybridize() loss = gluon.loss.SoftmaxCrossEntropyLoss() trainer = gluon.Trainer(net.collect_params(), 'sgd', { 'learning_rate': learning_rate, 'wd': 0.001}) d2l.train_ch13(net, train_iter, test_iter, loss, trainer, num_epochs, devices)
我們將基礎學習率設置為一個較小的值,以便微調 通過預訓練獲得的模型參數。基于前面的設置,我們將使用十倍大的學習率從頭開始訓練目標模型的輸出層參數。
train_fine_tuning(finetune_net, 5e-5)
loss 0.201, train acc 0.930, test acc 0.948 888.0 examples/sec on [device(type='cuda', index=0), device(type='cuda', index=1)]
train_fine_tuning(finetune_net, 0.01)
loss 0.190, train acc 0.931, test acc 0.946 19.3 examples/sec on [gpu(0), gpu(1)]
為了進行比較,我們定義了一個相同的模型,但將其所有模型參數初始化為隨機值。由于整個模型需要從頭開始訓練,我們可以使用更大的學習率。
scratch_net = torchvision.models.resnet18() scratch_net.fc = nn.Linear(scratch_net.fc.in_features, 2) train_fine_tuning(scratch_net, 5e-4, param_group=False)
loss 0.382, train acc 0.832, test acc 0.833 1571.7 examples/sec on [device(type='cuda', index=0), device(type='cuda', index=1)]
scratch_net = gluon.model_zoo.vision.resnet18_v2(classes=2) scratch_net.initialize(init=init.Xavier()) train_fine_tuning(scratch_net, 0.1)
loss 0.383, train acc 0.833, test acc 0.836 21.0 examples/sec on [gpu(0), gpu(1)]
正如我們所看到的,微調模型在同一時期往往表現更好,因為它的初始參數值更有效。
14.2.3。概括
遷移學習將從源數據集學到的知識遷移到目標數據集。微調是遷移學習的常用技術。
目標模型從源模型中復制所有模型設計及其參數(輸出層除外),并根據目標數據集微調這些參數。相比之下,目標模型的輸出層需要從頭開始訓練。
一般微調參數使用較小的學習率,而從頭訓練輸出層可以使用較大的學習率。
14.2.4。練習
不斷提高 的學習率finetune_net。模型的準確性如何變化?
在對比實驗中進一步調整 和 的超參數finetune_net。 scratch_net它們的準確性仍然不同嗎?
將輸出層之前的參數設置finetune_net為源模型的參數,并且在訓練期間不更新它們。模型的準確性如何變化?您可以使用以下代碼。
事實上,ImageNet數據集中有一個“熱狗”類。其在輸出層對應的權重參數可以通過以下代碼獲取。我們如何利用這個權重參數?
-
數據集
+關注
關注
4文章
1208瀏覽量
24726 -
pytorch
+關注
關注
2文章
808瀏覽量
13240
發布評論請先 登錄
相關推薦
Xilinx ISE Design Suite 14.2 安裝圖解
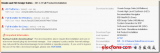
PyTorch教程-16.7。自然語言推理:微調 BERT
E2094P IO Libraries Suite 14.2數據表
如何往星光2板子里裝pytorch?
如何讓14.2版的Differential pair Con
iOS 14.2被破解!Checkra1n越獄更新!
PyTorch教程16.6之針對序列級和令牌級應用程序微調BERT
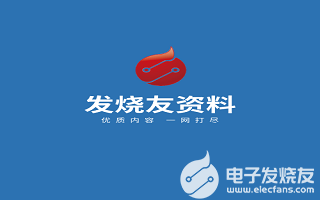
評論