1.vector容器介紹
向量(Vector)是一個封裝了動態大小數組的順序容器(Sequence Container)。跟任意其它類型容器一樣,它能夠存放各種類型的對象。可以簡單的認為,向量是一個能夠存放任意類型的動態數組。
1.1 vector和普通數組區別
普通數組是靜態的,在初始化是就確定空間大小,不支持動態擴展;
vector則可以看做是一個動態的數組,可以存放任意數據(基本數據類型和自定義數據類型均可以),支持動態擴展空間;
2.vector容器的構造函數
vector容器,可以看做是一個單端數組,構造函數有:無參構造、有參構造、拷貝構造。
vector 容器:--->單端數組
vector和普通數組的區別:
普通數組是靜態空間,創建是就分配好
vector支持動態擴展
vector容器常用迭代器:
v.rend() -->指向第一個元素的前一個位置
v.end() -->指向最后一個元素的下一個位置
v.begin() -->指向最后一個元素
vector容器的迭代器是支持隨機訪問的迭代器
vector構造函數:
無參構造:vector v;
有參構造:vector(v.begin(),b.end()); --將begin到end之間的內容拷貝
vector(n,elem); //將n個elem內容拷貝
拷貝構造:vector(const vector &v);
vector構造函數使用示例:
#include
using namespace std;
#include
void PrintVector(const vector& ptr)
{
//若傳入的vector是一個常量,則才是需要迭代器是需要使用:const_iterator
for ( vector:: const_iterator v = ptr.begin(); v != ptr.end(); v++)
{
cout vtr;//默認構造
for (int i = 0; i < 5; i++)
{
vtr.push_back(i);
}
PrintVector(vtr);
vector v2(vtr.begin(), vtr.end());//將begin~end之間的內容拷貝
PrintVector(v2);
vector v3(10, 5);//賦值10個5
PrintVector(v3);
vectorv4(v3);//拷貝構造
PrintVector(v4);
}
int main()
{
test();
system("pause");
}
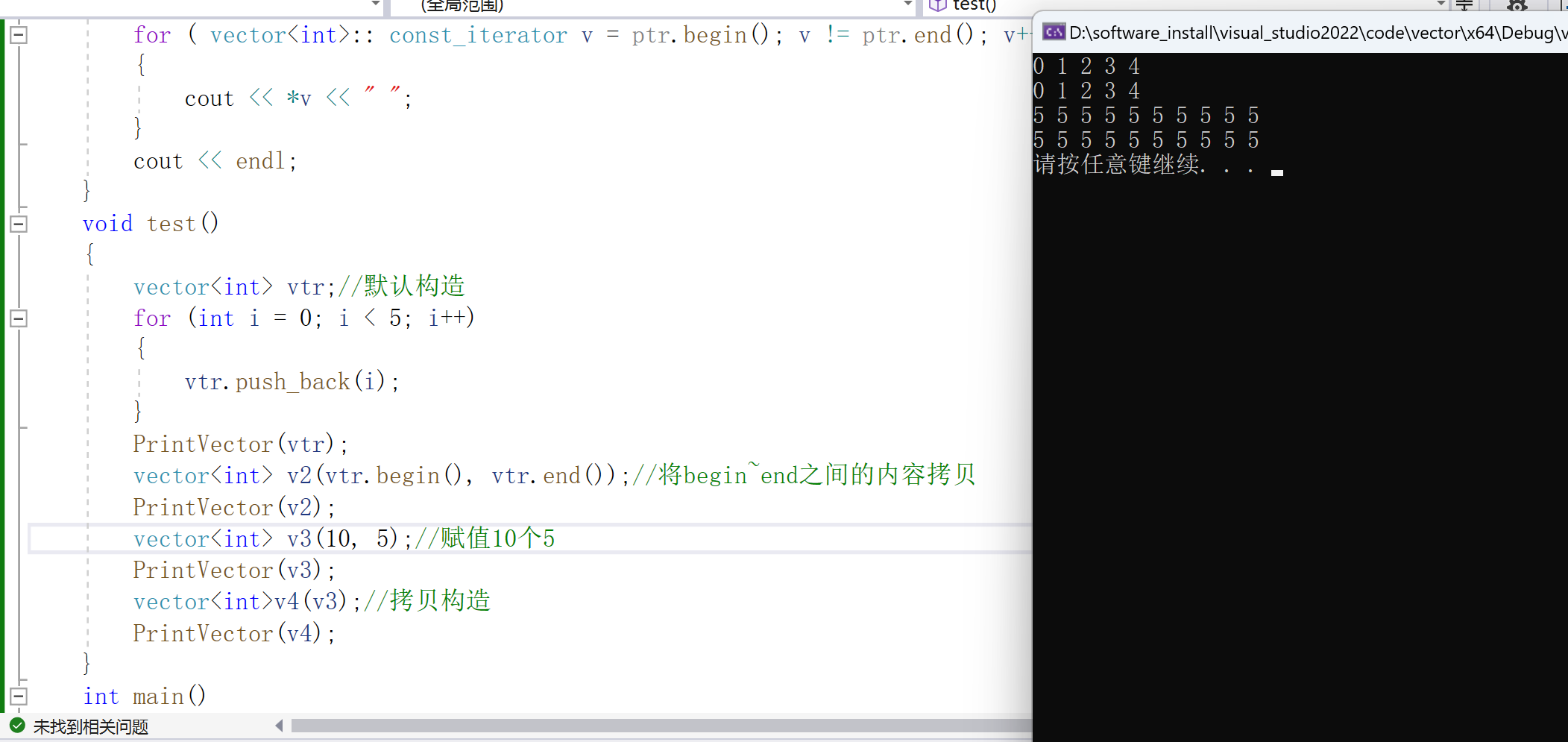
3.vector賦值
vector賦值可以直接"="賦值,也可以使用成員函數assign賦值。
vector賦值:
vector &operator=(const vector &v);//重載=
assign(beg,end);//將beg~end區間進行賦值
assign(n,elem);//n個elem賦值
賦值操作示例:
#include
using namespace std;
#include
void PrintVector(const vector& p)
{
for (vector::const_iterator ptr = p.begin(); ptr != p.end(); ptr++)
{
cout v;
for (int i = 0; i < 5; i++)
{
v.push_back(i);
}
PrintVector(v);
vector v2 = v;//等號賦值
PrintVector(v2);
vector v3(v.begin(), v.end());//區間賦值
PrintVector(v3);
vector v4(5, 666);//5個666賦值
PrintVector(v4);
}
int main()
{
test();
system("pause");
}
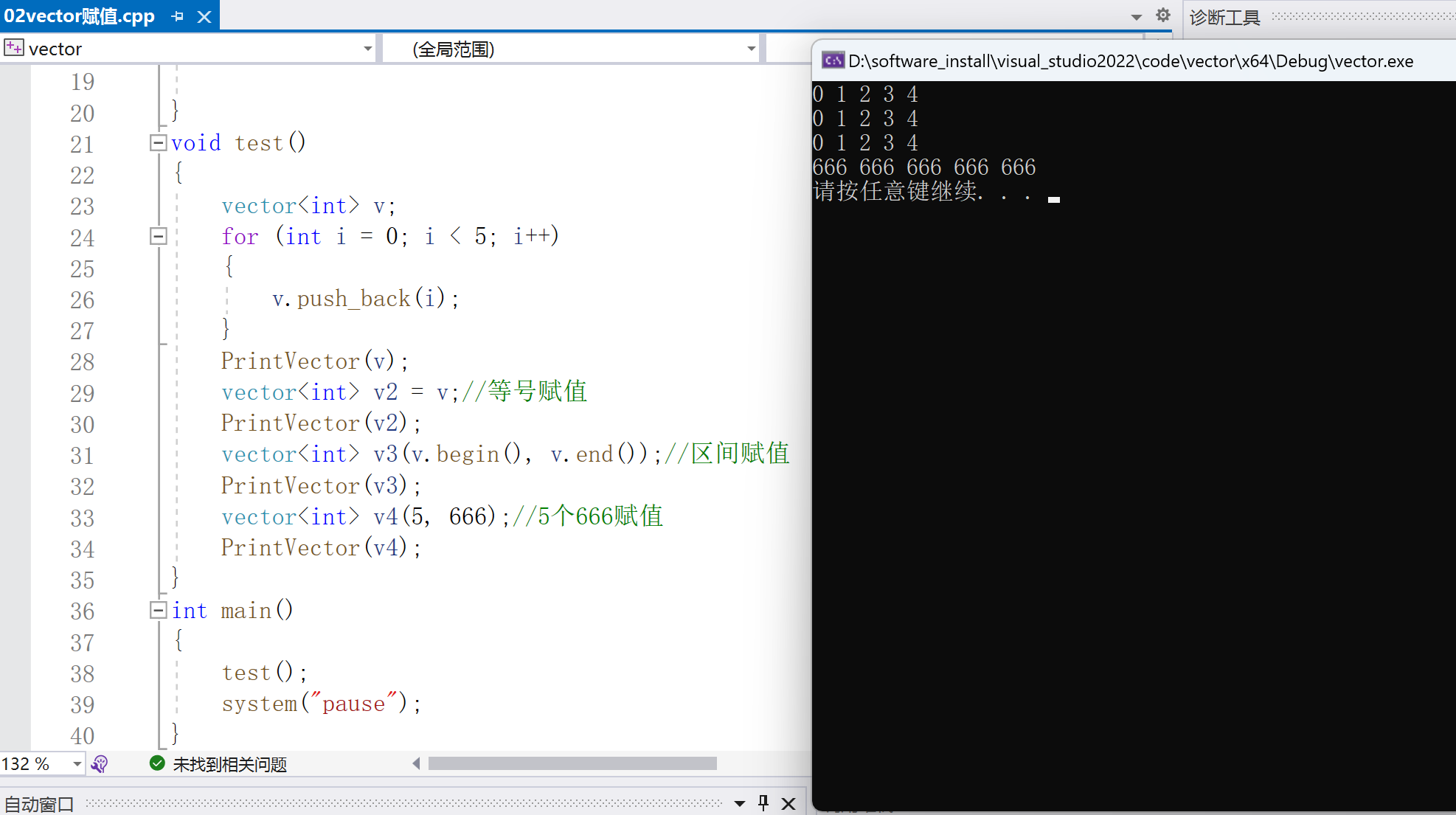
4.vector獲取容量和成員個數
vector和普通數組一樣,下標是從0開始的。獲取容量大小使用capacity()函數,判斷容器是否為空可以使用empty()函數,獲取成員個數使用size()函數。還可以使用resize函數指定容器大小;
vector容器的容量和成員個數:
判斷vector容器是否為空:empty()
容量:capacity()
容器中的元素個數:size()
指定容器長度為num:resize(int num);
若容器變長,則以默認值填充,默認值為0
若容器變小,則末尾超出的元素將被刪除
指向容器的長度為num:resize(int num,elem);
若容器變長,則用elem填充
若容器變小,則末尾超出的元素將被刪除
使用示例:
#include
using namespace std;
#include
void PrintVector(const vector& p)
{
for (vector::const_iterator ptr = p.begin(); ptr != p.end(); ptr++)
{
cout vtr;
for (int i = 0; i < 5; i++)
{
vtr.push_back(i);
}
PrintVector(vtr);
//指定容器長度
vtr.resize(10,666);//指定長度為10,超出則用666填充
PrintVector(vtr);
vtr.resize(3);//指定長度小于實際長度,則會刪除超出的元素,但空間超出的空間還是存在
PrintVector(vtr);
vectorv2;
v2.resize(5);//沒有指定填充值則默認為0
if (v2.empty())
{
cout
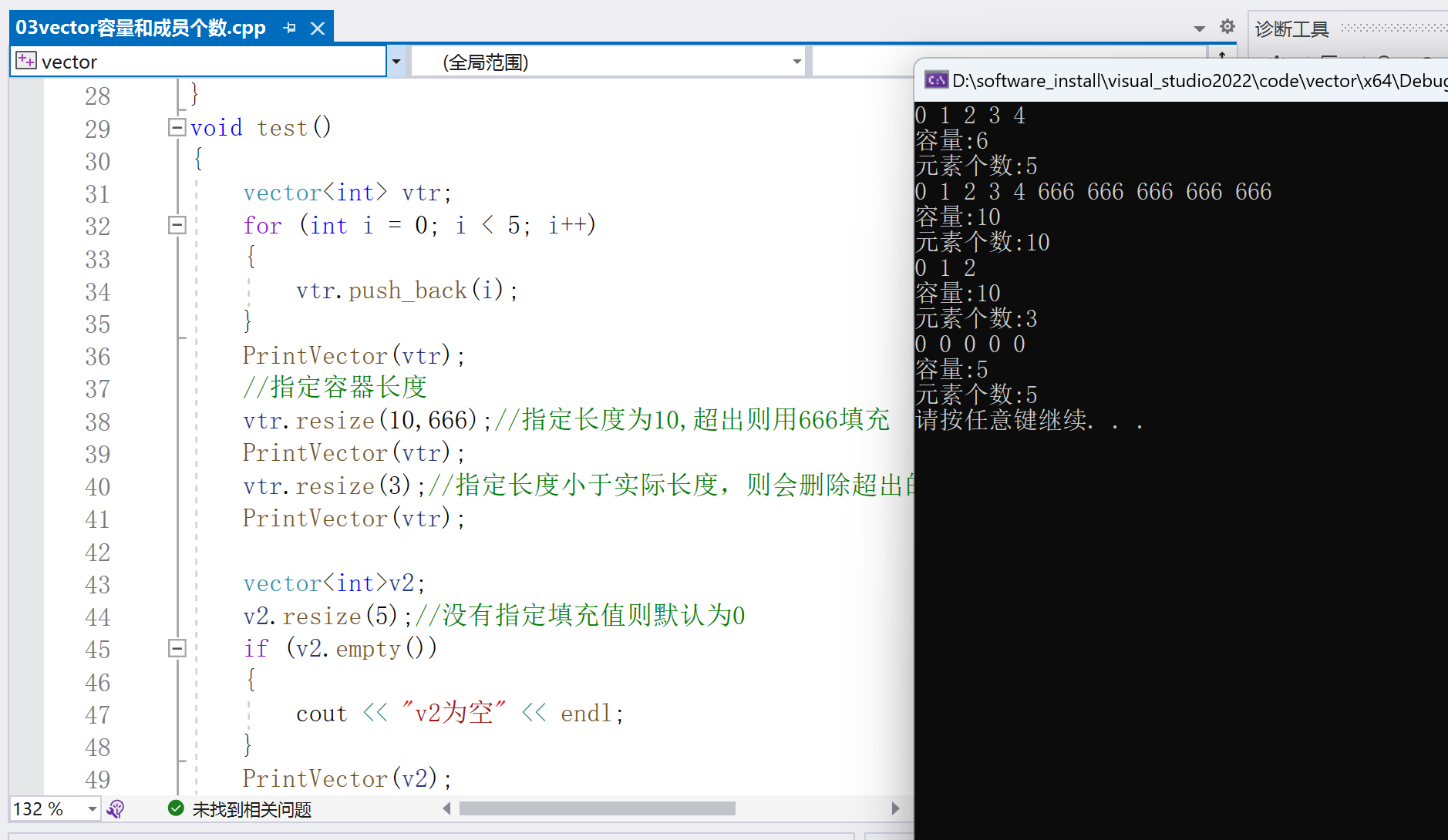
注意:在resize()函數時,若指定的大小比原空間大,則容器會進行擴充;若指定的大小比原空間小,則會將超出的成員刪除,但空間大小不就刪除。
5.vector容器成員刪除與插入
vector容器是一個單端數組,通過bush_back()函數可以實現從末尾插入數據。
pop_back()從末尾刪除數據;
從指定位置插入數據可以使用insert()成員函數,該函數有多個重載版本。
要從指定位置刪除數據可以使用erase()成員函數,該函數有多個重載版本;
clear()函數實現清空容器。
vector插入與刪除:
push_back();//尾插
pop_back();//尾刪
insert(const_iterator pos,elem);//迭代器指向位置pos插入元素elem
insert(const_iterator pos,int count,elem)//迭代器指向位置pos插入count個元素elem
erase(const_iterator pos);//刪除迭代器指向的元素
erase(const_iterator start,const_iterator end);//刪除迭代器start~end之間的元素
clear();//刪除容器中所有元素
實現示例:
#include
using namespace std;
#include
class Person
{
friend ostream& operatorage = p.age;
this->name = p.name;
return *this;
}
private:
int age;
string name;
};
ostream& operator& p)
{
for (vector::const_iterator ptr = p.begin(); ptr != p.end(); ptr++)
{
cout vtr;
//尾插
for (int i = 0; i < 5; i++)
{
vtr.push_back(v[i]);//賦值
}
PrintVector(vtr);
//尾刪
cout ::iterator ptr = vtr.begin();
ptr += 3;
vtr.insert(ptr, 3,temp);
PrintVector(vtr);
cout
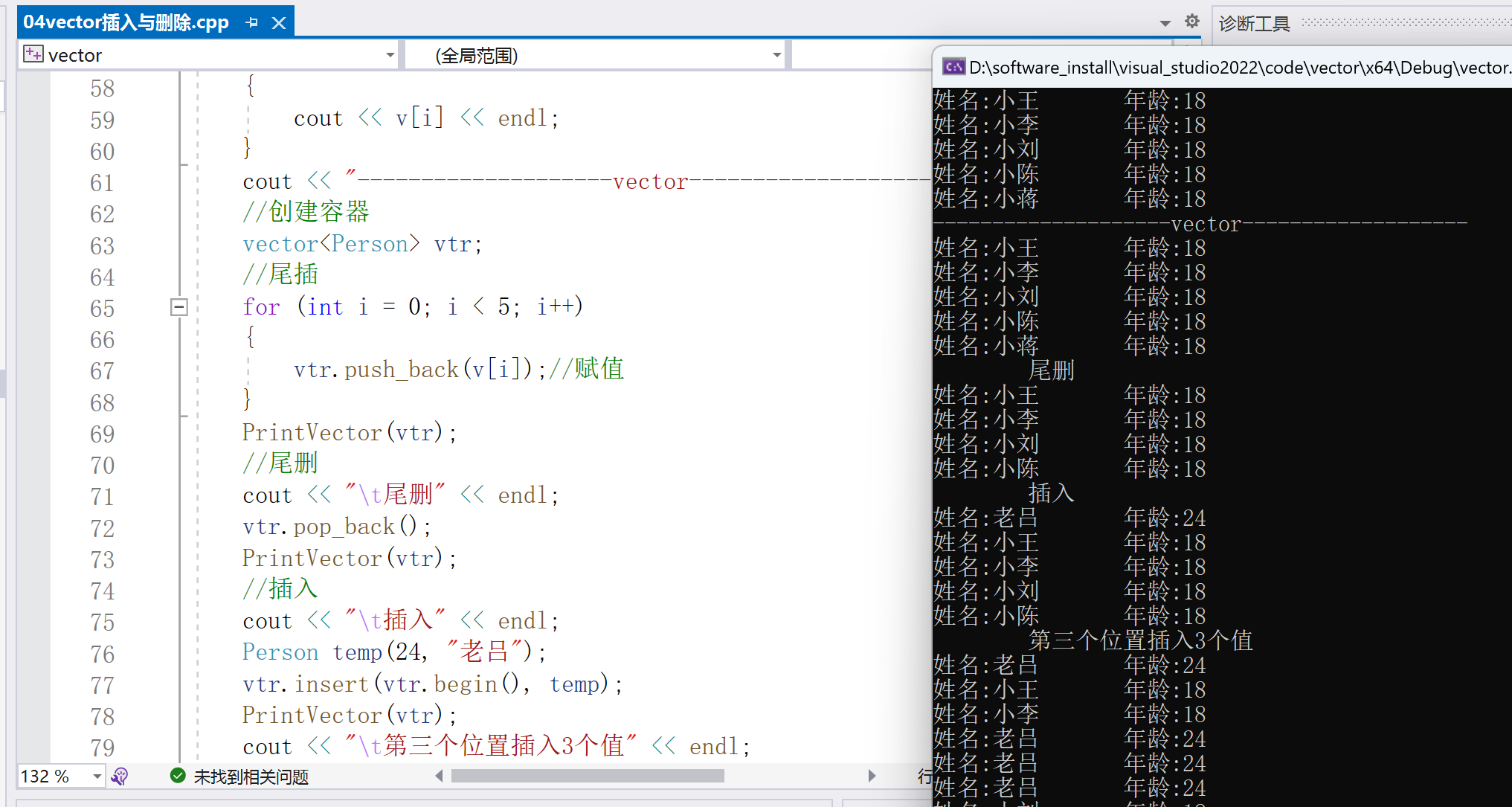
6.vector容器數據存取
vector容器也可以像普通數組一樣同[]訪問成員,此外還可以使用at()成員函數實現數據讀寫;
vector數據存取
at(int idx);//返回下標對應的內容
operator[];//重載[]
front();//返回容器中第一個元素
back();//返回容器中最后一個元素
實現示例:
#include
using namespace std;
#include
#include
void PrintVector(int val)
{
cout vtr;
for (int i = 0; i < 5; i++)
{
vtr.push_back(i);
}
for_each(vtr.begin(), vtr.end(), PrintVector);
cout
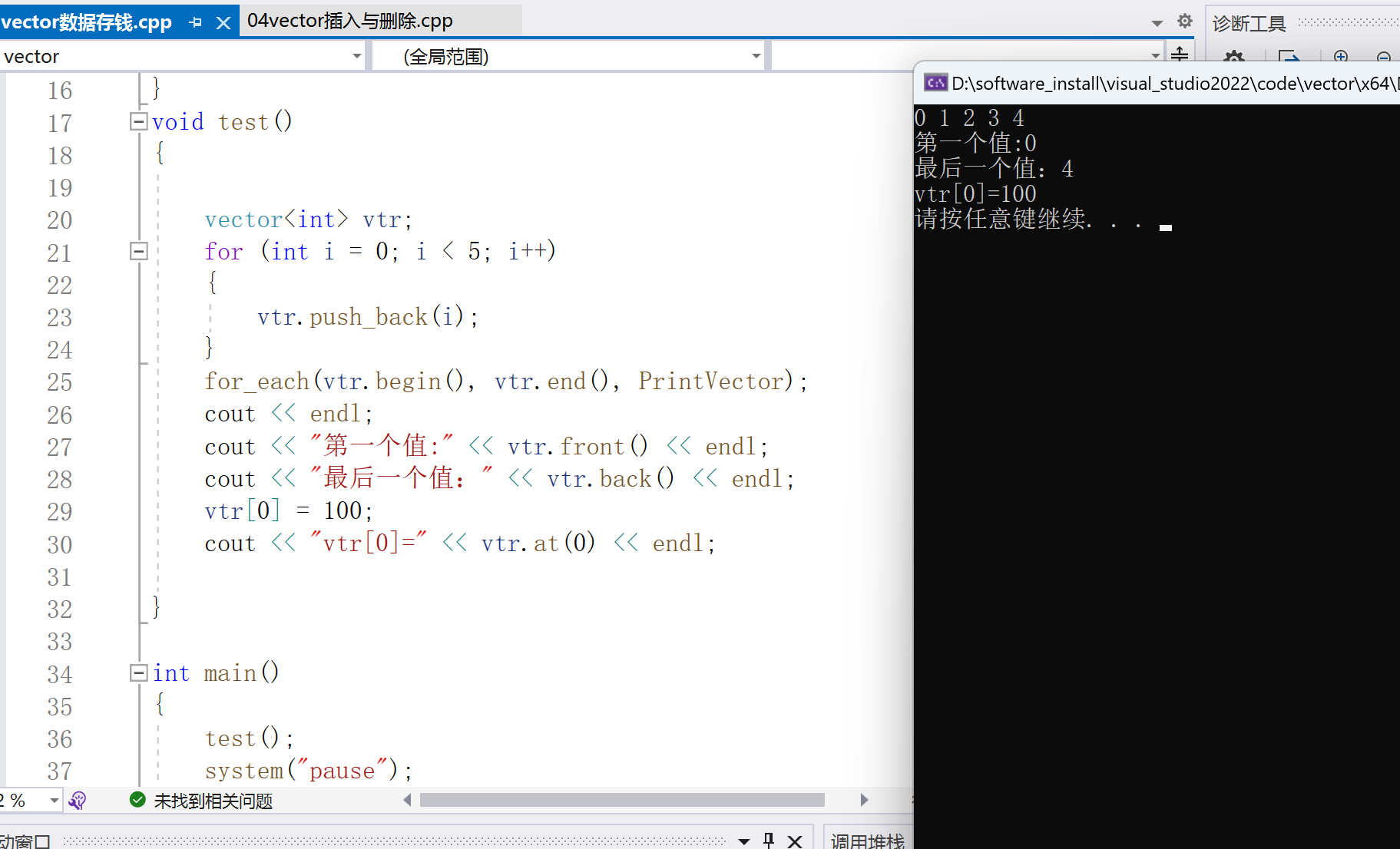
7.vector容器互換元素
在vector容器中,可以通過成員函數swap()函數實現兩個容器的成員互換。
注意:swap互換元素同時也可將空間大小進行互換。
vector容器互換
swap(vec);//將vec中的元素和本身的元素互換
注意:swap互換元素同時也可將空間大小進行互換
使用示例:
#include
#include
using namespace std;
void PrintVector(const vector& p)
{
for (vector::const_iterator ptr = p.begin(); ptr != p.end(); ptr++)
{
cout vtr(10,666);
vector vtr2;
for (int i = 0; i < 5; i++)
{
vtr2.push_back(i);
}
cout (vtr).swap(vtr);
/*
vector(vtr) --使用匿名對象,將匿名對象初始化為vtr
vector(vtr).swap(vtr); --在通過swap函數和匿名對象互換,此時即可實現收縮內存
*/
cout
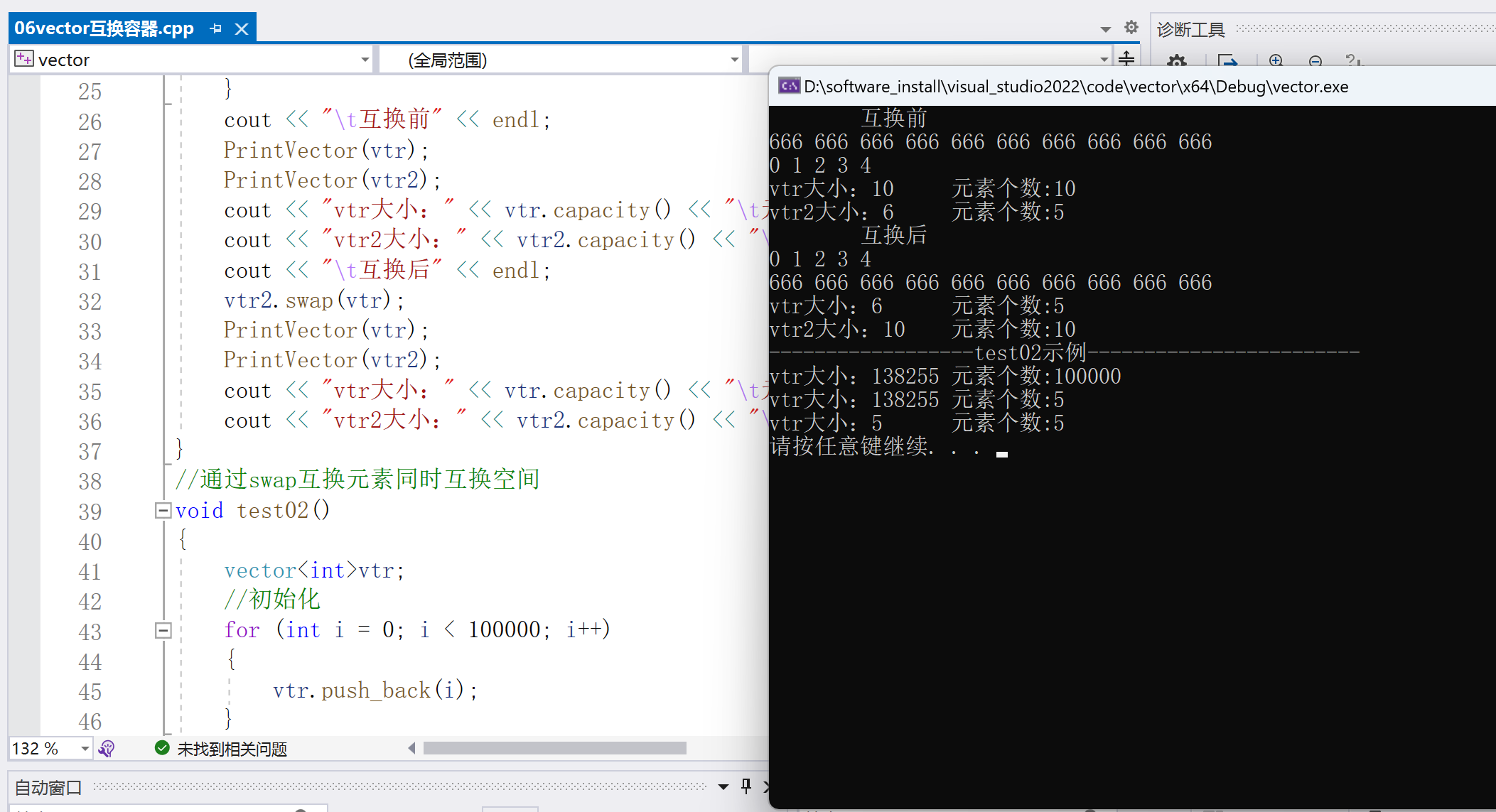
由于swap函數不僅可以互換元素,而且空間也大小也是可以互換的,所以有些清空下可以使用swap()函數來合理使用空間,避免空間資源浪費。
8.vector容器預留空間
reserve()成員函數可以指定空間大小,為vector容器預留空間。
reserve函數和resize()函數區別:
resize()函數指定大小后會直接初始化空間;
reserve()函數指定的大小不會初始化空間,預留的空間不能直接訪問,必須在賦值之后采用訪問。
reserve(int len);//容器預留len長度的元素,預留位置初始化,元素不可訪問
使用示例:
#include
using namespace std;
#include
void test()
{
vectorvtr;
vtr.reserve(10);
cout vtr;
int* p=NULL;
cout ()
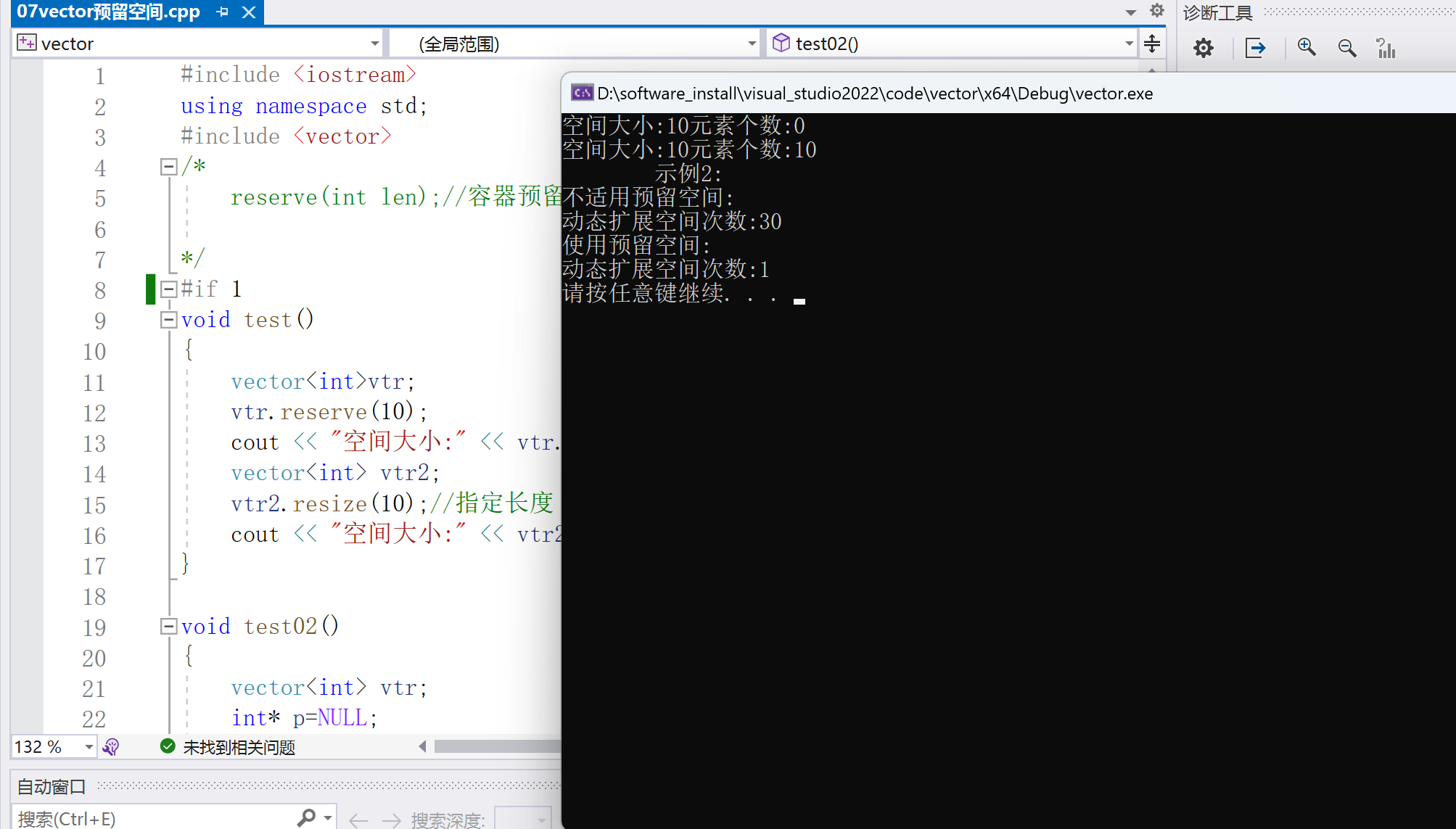
vector容器空間是根據成員來動態擴展,若一開始就知道使用的空間大概需要多大,則可以使用reserve()函數來指定,從而可以減少中間動態擴展的次數。
聲明:本文內容及配圖由入駐作者撰寫或者入駐合作網站授權轉載。文章觀點僅代表作者本人,不代表電子發燒友網立場。文章及其配圖僅供工程師學習之用,如有內容侵權或者其他違規問題,請聯系本站處理。
舉報投訴
-
編程
+關注
關注
88
文章
3689
瀏覽量
95330
-
函數
+關注
關注
3
文章
4383
瀏覽量
65000
-
容器
+關注
關注
0
文章
511
瀏覽量
22481
-
C++
+關注
關注
22
文章
2119
瀏覽量
75398
-
Vector
+關注
關注
3
文章
76
瀏覽量
9308
發布評論請先 登錄
相關推薦
熱點推薦
ccs如何使用C語言的“容器”?
c++/c標準庫有一個“容器”的東西,“vector”。我現在用的是ccs3.3,已經發現在安裝路徑下有“vector.h”的頭文件,以及相
發表于 05-08 19:49
C++ vector刪除符合條件元素的編程技巧
C++ vector中實際刪除元素使用的是容器vecrot中std::vector::erase()方法。 C++ 中std::remove
C++中vector的定義與初始化
C++中的vector vector(向量)是一種序列式容器,類似于數組,但比數組更優越。一般來說數組不能動態拓展,因此在程序運行的時候不是浪費內存,就是造成越界。而
C++入門之string
前一篇文章我們已經了解了C++中的基本類型,C++還提供了很多抽象數據類型,例如字符串string,string包含多個字符,以及可變長度的vector,vector可以包含多個同一類
C++入門之數組的概念
上一篇文章我們介紹了C++中的迭代器,這篇文章將會介紹C++中數組的概念,數組是一種和vector類似的數據結構,但是其在性能和靈活性上的權衡中選擇了性能而放棄了一定的靈活性,其與vector
C++學習筆記之順序容器
C++中的順序容器是一種用于存儲和管理元素序列的數據結構。它們提供了一組有序的元素,并支持在序列的任意位置插入和刪除元素。C++標準庫中提供了多種順序容器,包括
C++入門之通用算法
C++ 是一種強大的編程語言,它提供了許多通用算法,可以用于各種容器類型。這些算法是通過迭代器來操作容器中的元素,因此它們是通用的,可以用于不同類型的容器。在本篇博客中,我們將詳細介紹
動態數組和C++ std::vector詳解
std::vector是C++的默認動態數組,其與array最大的區別在于vector的數組是動態的,即其大小可以在運行時更改。std::vector是封裝動態數組的順序
C++之父新作帶你勾勒現代C++地圖
為了幫助大家解決這些痛點問題,讓大家領略現代C++之美,掌握其中的精髓,更好地使用C++,C++之父Bjarne Stroustrup坐不住了,他親自操刀寫就了這本《
評論