Rust語言是一種系統(tǒng)級(jí)、高性能的編程語言,其設(shè)計(jì)目標(biāo)是確保安全和并發(fā)性。
Rust語言以C和C++為基礎(chǔ),但是對(duì)于安全性和并發(fā)性做出了很大的改進(jìn)。
在Rust語言中,操作文件是非常重要的一個(gè)功能,本教程將介紹如何在Rust中高效地操作文件,并提供多個(gè)實(shí)際應(yīng)用示例。
文件讀取
Rust語言中操作文件的第一步就是文件讀取,使用Rust內(nèi)置的std::fs::File
類型即可。使用File
類型可以打開一個(gè)文件,并且從中讀取數(shù)據(jù)。
use std::fs::File;
use std::io::prelude::*;
fn main() - > std::io::Result< () > {
let mut f = File::open("file.txt")?;
let mut contents = String::new();
f.read_to_string(&mut contents)?;
println!("{}", contents);
Ok(())
}
上面的代碼中調(diào)用File::open()
函數(shù)打開文件,然后向其中讀取數(shù)據(jù)。讀取的數(shù)據(jù)存儲(chǔ)在contents
變量中,并使用println!()
函數(shù)將其輸出到控制臺(tái)。
注意,read_to_string()
函數(shù)是阻塞式的,因此當(dāng)文件非常大時(shí),應(yīng)該使用每次讀取一小塊數(shù)據(jù)這種方式讀取,而不是將整個(gè)文件讀入內(nèi)存。
文件追加寫入
在Rust語言中,將數(shù)據(jù)寫入文件的方法是使用write_all()
函數(shù)。write_all()
函數(shù)的作用是寫入一個(gè)字節(jié)數(shù)組或字符串到文件中。但是使用此函數(shù)寫入,是直接覆蓋文件內(nèi)容,即覆蓋原有文件內(nèi)容。如果要進(jìn)行文件追加寫入,應(yīng)該使用Rust內(nèi)置的std::fs::OpenOptions
類型。
use std::fs::OpenOptions;
use std::io::prelude::*;
fn main() - > std::io::Result< () > {
let mut file = OpenOptions::new()
.append(true)
.create(true)
.open("file.txt")?;
file.write_all(b"Hello, world!")?;
Ok(())
}
上面的代碼中,使用OpenOptions
打開文件,并使用append()
函數(shù)將文件的打開方式設(shè)置為追加。使用create()
函數(shù)則用于創(chuàng)建不存在的文件,如果文件存在,仍然可以正常打開。然后使用write_all()
函數(shù)將數(shù)據(jù)寫入文件中。
注意:文件追加寫入是在原文件內(nèi)容后追加,而不是從文件尾部開始寫入。因此,如果在追加寫入數(shù)據(jù)時(shí)需要將數(shù)據(jù)寫入最后,應(yīng)該先使用seek()
函數(shù)將指針移動(dòng)到文件尾部。
文件寫入
要在Rust語言中進(jìn)行文件寫入,首先需要?jiǎng)?chuàng)建一個(gè)新文件或覆蓋現(xiàn)有文件內(nèi)容。這可以通過std::fs::File
類型和std::fs::OpenOptions
類型中的create()
函數(shù)實(shí)現(xiàn)。另外,要將數(shù)據(jù)寫入文件中,write_all()
函數(shù)是不錯(cuò)的選擇。
use std::fs::OpenOptions;
use std::io::prelude::*;
fn main() - > std::io::Result< () > {
let mut file = OpenOptions::new()
.write(true)
.create(true)
.open("file.txt")?;
file.write_all(b"Hello, world!")?;
Ok(())
}
上面的代碼中使用OpenOptions
打開文件,并使用write()
函數(shù)將文件的打開方式設(shè)置為寫入(即覆蓋原有內(nèi)容)。使用create()
函數(shù)則用于創(chuàng)建不存在的文件,如果文件存在,仍然可以正常打開。然后使用write_all()
函數(shù)將數(shù)據(jù)寫入文件中。
文件復(fù)制
Rust語言中可以使用std::fs::copy()
函數(shù)將一個(gè)文件復(fù)制到另一個(gè)文件中。
use std::fs;
fn main() - > std::io::Result< () >{
fs::copy("file.txt", "file_copy.txt")?;
Ok(())
}
上面的代碼中,Copy
函數(shù)將file.txt
的所有內(nèi)容復(fù)制到file_copy.txt
文件中。如果文件已經(jīng)存在,則原有文件內(nèi)容將被覆蓋。
文件元數(shù)據(jù)
在Rust語言中,File
類型還提供了一些用于獲取文件元數(shù)據(jù)的函數(shù),如metadata()
函數(shù)。此函數(shù)返回一個(gè)std::fs::Metadata
類型的元數(shù)據(jù)結(jié)構(gòu)體,該結(jié)構(gòu)體包含了文件的大小、創(chuàng)建時(shí)間、修改時(shí)間、權(quán)限等信息。
use std::fs::metadata;
use std::time::SystemTime;
fn main() - > std::io::Result< () > {
let metadata = metadata("file.txt")?;
let created = metadata.created()?;
let modified = metadata.modified()?;
let size = metadata.len();
let perms = metadata.permissions();
println!("Created: {:?}", created);
println!("Modified: {:?}", modified);
println!("Size: {} bytes", size);
println!("Permissions: {:?}", perms);
Ok(())
}
上面的代碼中,metadata()
函數(shù)返回文件file.txt
的元數(shù)據(jù),并使用元數(shù)據(jù)中的created()
函數(shù)和modified()
函數(shù)獲取創(chuàng)建時(shí)間和修改時(shí)間,使用len()
函數(shù)來獲取文件大小(字節(jié)數(shù)),使用permissions()
函數(shù)獲取文件的權(quán)限。
文件重命名和移動(dòng)
在Rust語言中,使用std::fs::rename()
函數(shù)可以將文件重命名或者移動(dòng)到其他文件夾中。
use std::fs::rename;
fn main() - > std::io::Result< () > {
rename("file.txt", "new_file.txt")?;
Ok(())
}
上面的代碼中,rename()
函數(shù)將文件file.txt
重命名為new_file.txt
,如果new_file.txt
文件已經(jīng)存在,則重命名將失敗。
此外,如果要移動(dòng)文件到其他文件夾中,則可以在目標(biāo)文件名中指定文件夾路徑。例如,如果我們將文件移動(dòng)到子文件夾/path/to/subdir/
中,則可以在目標(biāo)文件名中指定路徑:/path/to/subdir/new_file.txt
。
多種操作組合
在Rust語言中,可以將多種文件操作組合使用,例如讀取文件,刪除文件內(nèi)容,然后將新數(shù)據(jù)寫入文件中。
use std::fs::OpenOptions;
use std::io::prelude::*;
fn main() - > std::io::Result< () > {
let mut file = OpenOptions::new()
.read(true)
.write(true)
.open("file.txt")?;
let mut contents = String::new();
file.read_to_string(&mut contents)?;
contents = contents.replace("Hello", "World");
file.set_len(0)?; // 清空文件
file.write_all(contents.as_bytes())?;
Ok(())
}
上面的代碼中,使用OpenOptions
打開文件,并使用read()
函數(shù)將文件的打開方式設(shè)置為讀取,同時(shí)打開文件寫入的功能。讀取文件的內(nèi)容,并使用replace()
函數(shù)將文本中的“Hello”替換為“World”。然后使用set_len()
函數(shù)將文件長度重置為0(即清空文件)。使用write_all()
函數(shù)將新數(shù)據(jù)寫入文件。
擴(kuò)展閱讀 - 讀取帶BOM頭的文件
BOM (Byte Order Mark) 是一個(gè)Unicode字符,用于標(biāo)識(shí)文件的編碼格式(UTF-8, UTF-16LE, UTF-16BE, UTF-32LE, UTF-32BE…)。BOM通常是在文件開頭的位置插入的,用于確定字符的順序和字節(jié)順序。
源于Unicode編碼,目前被廣泛使用于自定義字符集。例如:GB18030-2022
讀取帶BOM頭的文件
pub trait BOMReader {
fn has_bom(&self) - > bool;
fn read_content(&mut self) - > Result< String, std::io::Error >;
}
pub struct FileBOMReader {
file: std::fs::File,
bom: Option< Vec< u8 >>,
}
impl FileBOMReader {
pub fn new(file: std::fs::File) - > Self {
Self { file, bom: None }
}
fn read_bom(&mut self) - > Result< (), std::io::Error > {
let mut bom_buf = [0u8; 3];
let bytes_read = self.file.read(&mut bom_buf)?;
if bytes_read >= 3 && bom_buf[..3] == [0xEF, 0xBB, 0xBF] {
self.bom = Some(bom_buf[..3].to_vec());
} else if bytes_read >= 2 && bom_buf[..2] == [0xFE, 0xFF] {
self.bom = Some(bom_buf[..2].to_vec());
} else if bytes_read >= 2 && bom_buf[..2] == [0xFF, 0xFE] {
self.bom = Some(bom_buf[..2].to_vec());
} else if bytes_read >= 4 && bom_buf[..4] == [0x00, 0x00, 0xFE, 0xFF] {
self.bom = Some(bom_buf[..4].to_vec());
} else if bytes_read >= 4 && bom_buf[..4] == [0xFF, 0xFE, 0x00, 0x00] {
self.bom = Some(bom_buf[..4].to_vec());
}
Ok(())
}
}
impl BOMReader for FileBOMReader {
fn has_bom(&self) - > bool {
self.bom.is_some()
}
fn read_content(&mut self) - > Result< String, std::io::Error > {
if self.bom.is_none() {
self.read_bom()?;
}
let mut buf = String::new();
self.file.read_to_string(&mut buf)?;
if self.has_bom() {
match &self.bom {
Some(bom) if bom.starts_with([0xEF, 0xBB, 0xBF].as_ref()) = > {
buf.drain(..3);
}
Some(bom) if bom.starts_with([0xFF, 0xFE].as_ref()) = > {
buf = buf.as_bytes().chunks_exact(2).map(|c| c[1]).collect();
}
Some(bom) if bom.starts_with([0xFE, 0xFF].as_ref()) = > {
buf = buf.as_bytes().chunks_exact(2).map(|c| c[0]).collect();
}
Some(bom) if bom.starts_with([0x00, 0x00, 0xFE, 0xFF].as_ref()) = > {
buf = buf.as_bytes().chunks_exact(2).skip(2).map(|c| c[1]).collect();
}
Some(bom) if bom.starts_with([0xFF, 0xFE, 0x00, 0x00].as_ref()) = > {
buf = buf.as_bytes().chunks_exact(4).skip(1).flat_map(|c| &c[2..]).collect();
}
_ = > {}
}
}
Ok(buf)
}
}
該trait定義了一個(gè)BOMReader
并提供了一個(gè)FileBOMReader
的實(shí)現(xiàn),可檢測和讀取文件中的 BOM(Byte Order Mark)。BOM 通常用于標(biāo)識(shí)文件的編碼格式,因?yàn)槟承┚幋a格式的字符集在讀取時(shí)可能有不同的字節(jié)序。
示例代碼
use std::fs::File;
use std::io::{Read, Write};
fn main() {
let mut file = File::create("test_utf8.txt").unwrap();
let content = "Hello, World!n";
file.write_all(content.as_bytes()).unwrap();
let mut reader = FileBOMReader::new(File::open("test_utf8.txt").unwrap());
let result = reader.read_content().unwrap();
assert_eq!(result, content);
let mut file = File::create("test_utf16be.txt").unwrap();
let bom = [0xFE, 0xFF];
file.write_all(&bom).unwrap();
let content = "Hello, World!n";
file.write_all(content.as_bytes()).unwrap();
let mut reader = FileBOMReader::new(File::open("test_utf16be.txt").unwrap());
let result = reader.read_content().unwrap();
assert_eq!(result, content);
let mut file = File::create("test_utf16le.txt").unwrap();
let bom = [0xFF, 0xFE];
file.write_all(&bom).unwrap();
let content = "Hello, World!n";
file.write_all(content.as_bytes()).unwrap();
let mut reader = FileBOMReader::new(File::open("test_utf16le.txt").unwrap());
let result = reader.read_content().unwrap();
assert_eq!(result, content);
let mut file = File::create("test_utf32be.txt").unwrap();
let bom = [0x00, 0x00, 0xFE, 0xFF];
file.write_all(&bom).unwrap();
let content = "Hello, World!n";
file.write_all(content.as_bytes()).unwrap();
let mut reader = FileBOMReader::new(File::open("test_utf32be.txt").unwrap());
let result = reader.read_content().unwrap();
assert_eq!(result, content);
let mut file = File::create("test_utf32le.txt").unwrap();
let bom = [0xFF, 0xFE, 0x00, 0x00];
file.write_all(&bom).unwrap();
let content = "Hello, World!n";
file.write_all(content.as_bytes()).unwrap();
let mut reader = FileBOMReader::new(File::open("test_utf32le.txt").unwrap());
let result = reader.read_content().unwrap();
assert_eq!(result, content);
}
通過編寫這樣的例子,我們可以測試我們的代碼,確保它能正確地讀取各種類型的文件。
使用encoding_rs
讀取帶BOM頭的文件
在Rust中,可以使用std::fs::File
和std::io::BufReader
模塊讀取文件,并使用encoding_rs
模塊解析BOM頭以獲取文件的編碼信息。
use std::fs::File;
use std::io::BufReader;
use encoding_rs::Encoding;
fn main() {
let filename = "example.txt";
let file = File::open(filename).unwrap();
let mut reader = BufReader::new(file);
// 按照Utf8讀取文件 let decoder = Encoding::utf8().new_decoder_with_bom_handling();
let (result, _, _) = decoder.decode(&mut reader);
match result {
Some(s) = > {
println!("Content: {}", s);
}
None = > {
println!("Error decoding file");
}
}
}
這個(gè)示例使用了Utf8
編碼格式,但是在實(shí)現(xiàn)中使用了new_decoder_with_bom_handling()
函數(shù)以自動(dòng)檢測和處理BOM頭。
如果需要支持其他編碼類型,則需要使用不同的編碼器(比如GBK
)和相應(yīng)的 decoder。
// 按照GBK讀取文件
let decoder = Encoding::GBK.new_decoder_with_bom_handling();
// 解碼
let (result, _, _) = decoder.decode(&mut reader);
根據(jù)具體的編碼類型來選擇對(duì)應(yīng)的編碼器,就可以正常讀取文件內(nèi)容了。
總結(jié)
以上是在Rust語言中操作文件的實(shí)際應(yīng)用示例,涵蓋了文件讀取、追加寫入、重命名和移動(dòng)、復(fù)制、寫入、獲取元數(shù)據(jù)等操作。這些操作非常基礎(chǔ),但往往也是程序開發(fā)中必不可少的操作。在以后的程序開發(fā)中,讀者可以根據(jù)需求將這些操作進(jìn)行各種組合,以實(shí)現(xiàn)更為復(fù)雜的文件操作需求。
-
編程語言
+關(guān)注
關(guān)注
10文章
1942瀏覽量
34707 -
函數(shù)
+關(guān)注
關(guān)注
3文章
4327瀏覽量
62573 -
C++
+關(guān)注
關(guān)注
22文章
2108瀏覽量
73623 -
rust語言
+關(guān)注
關(guān)注
0文章
57瀏覽量
3009 -
Rust
+關(guān)注
關(guān)注
1文章
228瀏覽量
6601
發(fā)布評(píng)論請(qǐng)先 登錄
相關(guān)推薦
如何在Go中操作文本文件
如何在Rust中使用Memcached
Rust語言如何與 InfluxDB 集成
如何在Rust中連接和使用MySQL數(shù)據(jù)庫
如何在Rust中讀寫文件
電氣CAD文件中高效的工作流程
STM32F407使用FatFS,發(fā)現(xiàn)跟文件夾相關(guān)函數(shù)命令都可操作,但不能操作文件的函數(shù)
只會(huì)用Python?教你在樹莓派上開始使用Rust
如何在STM32 (Cortex M3)和GD32(RISC-V)上用Rust開發(fā)
Rust開發(fā)操作系統(tǒng)教程之如何自制一個(gè)操作系統(tǒng)
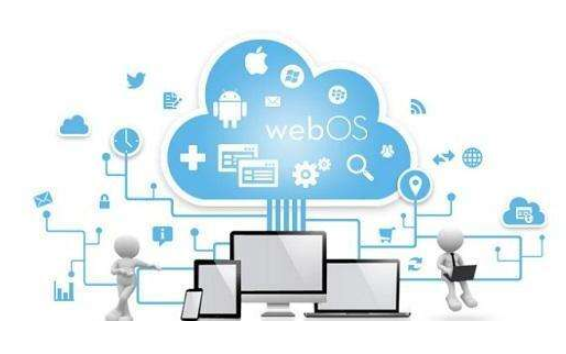
WeLink協(xié)作文檔,高效協(xié)同辦公的重要選擇
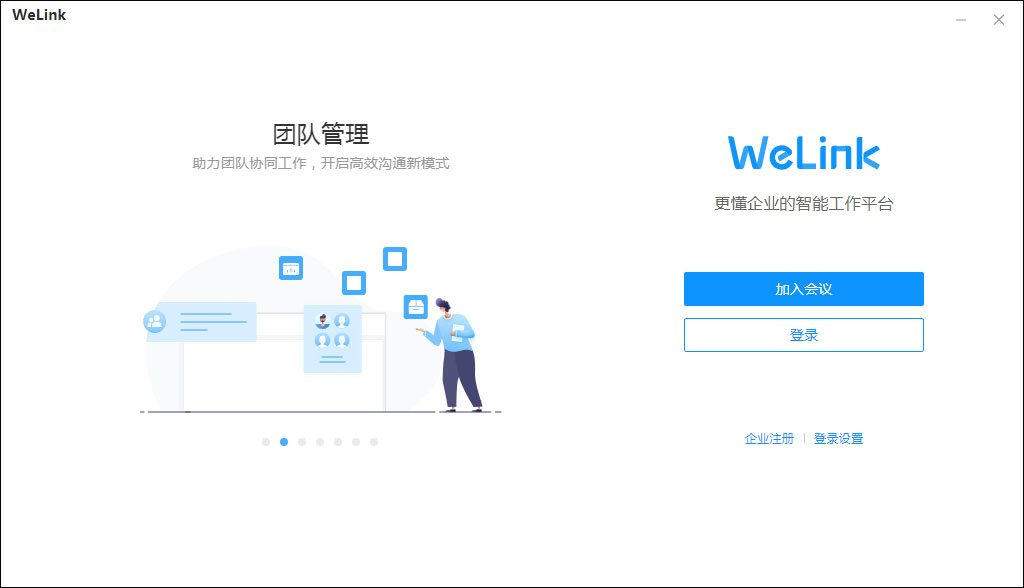
華為云WeLink協(xié)作文檔,助您開啟職場高效辦公
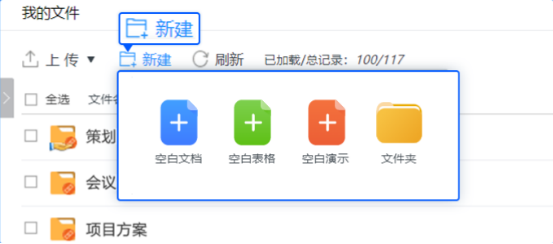
評(píng)論