問:Arduino Uno的函數示例
我決定自己編寫函數示例代碼,因為這應該是Arduino中的基本示例。網絡上確實有關于使用函數的文檔,但是,如果要嘗試使用代碼環境,則必須得訪問Arduino網站,而且他們的示例擴展的效果并不好。本帖將分成以下幾個部分:以不包含任何函數的起始代碼為例、使用一個函數轉換相同的程序、展示可以利用函數做些什么的擴展示例、與沒有函數的代碼版本進行比較,表明更少的代碼更易于使用。注意,本例假設已知一些基本的Arduino示例。
無函數的起始代碼
/*
* This code is the starting code for my functionExample, I want to turn pins 13, 12, 11 high; wait for half a second; turn all the pins off; wait for half a second;
* and turn pins 13 and 11 on while keeping 12 off and wait for another half second.
*/
void setup() {
pinMode(13, OUTPUT);
pinMode(12, OUTPUT);
pinMode(11, OUTPUT);
}
boolean writePin = HIGH; //set a global variable to make it easier to turn things on and off in the function, use !writePin for LOW
void loop() {
//first, let's see how cumbersome writing code can be when trying to run several LEDs
//write all output pins high:
digitalWrite(13, writePin);
digitalWrite(12, writePin);
digitalWrite(11, writePin);
delay(500); //delay half a second
//write all pins low
digitalWrite(13, !writePin);
digitalWrite(12, !writePin);
digitalWrite(11, !writePin);
delay(500); //delay half a second
//write 13 and 11 high, keep 12 low
digitalWrite(13, writePin);
digitalWrite(12, !writePin);
digitalWrite(11, writePin);
delay(500);//delay half a second
//doing that sequence alone takes 12 lines of code to accomplish something that could probably be done in less
}
// 20 lines of code not including comments
向上滑動查看完整代碼
我通常會計算包含{}的重要代碼行的數量,而不計算注釋,因為這些注釋無論如何都會在編譯器中被忽略。這篇代碼看起來非常笨拙,包含很多重復的行,因此如果需要執行不同的操作,你就必須進行更改。在進行故障排除或開發更為復雜的程序時,其效率會非常低下。
利用函數重新改造起始代碼
/*
TheArduino home page does
try toexplain funcitons using a math example and reading an analog pin, which iscool, but there are more things one can
do withfunctions. The anatomy of a function in Arduino is as follows:
dataTypevariableName(dataType parameterVariable1, dataType parameterVariable2, ...){}
Allfunctions have to have a valid Arduino dataType at the beginning of the line ofcode because we use a variable to reference the entire code that will be runbetween the {}
If youwant numbers or some sort of result with measurable values, you will have touse a data type that can store those values; examples are
int,double, unsigned long, long, boolean, float (and all the other types that canstore data)
The onlything to mention is that functions with these dataTypes must have a returnstatement; Example:
intaddTwoNumb(int one, int two){
intresult = one + two;
returnresult;
}
Thefunction above returns the calculation of one and two and can be stored in anew variable when called/used in the main loop.
Thevalues inside the () are called parameters, functions don't always requireparameters (most do though), so the () can be empty.
Parameters are placeholder variables with anydata type valid in Arduino. These variables do not have to be assigned valuesuntil the function is used/called in the main loop, values are passed as soonas they are called. Any number of new variables can be added into a functionand any amount of code can be added to a function, even functions can callother functions! (gets a little confusing at that point)
*/
void setup() {
pinMode(13, OUTPUT);
pinMode(12, OUTPUT);
pinMode(11, OUTPUT);
}
boolean writePin = HIGH; //set a globalvariable to make it easier to turn things on and off in the function, use!writePin for LOW
void loop() {
sequenceLights(13, 12, 11, writePin, writePin, writePin, 500);//call/use sequence lights on pins 13, 12, 11; use the default value forwritePin (HIGH), delay for half a second (500 mS)
sequenceLights(13, 12, 11, !writePin, !writePin, !writePin, 500);
sequenceLights(13, 12, 11, writePin, !writePin, writePin, 500);
}
/* Functions are typically defined or writtenbelow the loop statement for clarity*/
//notice that the function below uses the exactsame code but uses different variables and that we don't use a datatype (void),we aren't calculating anything, so there doesn't need to be a datatype
//the function takes 3 integers to begin with:pin13, pin12, pin11; these represent the values for the pins we want to control
//then the next three values are writeFunc1,writeFunc2, writeFunc3 which are boolean values used to control the pins (HIGHor LOW), we want to be able to use unique instructions per light as that is howthe original sequence worked
//finaly delayPeriod is used in place of 500,because what if we want to change the timing, why not?
void sequenceLights(int pin13, int pin12, intpin11, boolean writeFunc1, boolean writeFunc2, boolean writeFunc3, intdelayPeriod){
digitalWrite(pin13, writeFunc1);
digitalWrite(pin12, writeFunc2);
digitalWrite(pin11, writeFunc3);
delay(delayPeriod);
}
//17 lines of code not including comments
void setup() {
//Serial.begin(9600); //I often use the serial monitor to debug myprogram or to do some testing, I then comment the line of code to increase someruntime speed (counted this line of commented code as it was essential to myprocess)
pinMode(13, OUTPUT);
pinMode(12, OUTPUT);
pinMode(11, OUTPUT);
pinMode(10, OUTPUT);
pinMode(9, OUTPUT);
pinMode(8, OUTPUT);
}
int countRndSeq = 0; //set initial variable forcounting variable for the random sequence to "reset"
int randPin = random(8, 14); //set the randomPin variable to a random number between 8 and 13 (14 for including 13 as achoice); we only need 8-13 as those are the pins I use
void loop() {
randSequenceLightSlow(5, 125, 1200); //run function for a randomsequence, set counting interval at 5, set minimum delay at 125, set the maxdelay to 1200 (1.2 seconds) (I decided to move the randMode into the functionbecause it was easier to accomplish)
countRndSeq = 0; //reset the counter for the next sequence
delay(3000); //wait three seconds then turn off all the lights (made afunction for that)
allPinsWrite(13, 12, 11, 10, 9, 8 , LOW);
randSequenceLightSlow(7, 125, 1450); //run function for random sequenceusing counting interval at 7, minimum delay at 125, set max delay to 1450(1.450 seconds)
countRndSeq = 0; //reset counter for next sequence
delay(3000); //wait three seconds and turn off all the lights
allPinsWrite(13, 12, 11, 10, 9, 8, LOW);
}
/*
* Thefollowing uses a while loop for control, it does random lights and slows downdelay by a set interval between steps (larger interval will make it count andslow down much quicker)
* Aslong as that counting number (countRndSeq) is less than the max delay (maxDel),it will do the following in order:
* 1.chose a random pin 8-13 and set it equal to a variable
* 2.Pick the random Mode value each time the while loop runs again (increaseschance to get modes 0-2)
* 2.turn off the pin associated to that number using the delay amount of the samenumber that countrRndSeq is currently at
* 3.Increase countRndSeq by the interval amount set by calling the function (can beany integer)
* 4.Check if the current count is greater than the minimum delay and then checkwhich mode (remember I set it to random above); there are 3 modes 0, 1, 2
* NOTE:For #4, 0 has a high chance of adding less to countRndSeq, 1 has a mediumchance of adding a bit more to countRndSeq, 2 has a low chance of adding quitea bit to countRndSeq
* 5. Setthe final pin after the sequence has finished to high to simulate that is thefinal pin the program landed on
* SeecalcThreshChance for an explanation of how I made weighted random events forthe chance for the lights to slow faster
*/
void randSequenceLightSlow(int interval, intminDel, int maxDel){
while(countRndSeq < maxDel){
randPin = random(8, 14);
intmode = random(0, 3);
digitalWrite(randPin, HIGH);
delay(countRndSeq);
digitalWrite(randPin, LOW);
delay(countRndSeq);
countRndSeq += interval;
if(countRndSeq > minDel && mode == 0){
countRndSeq += calcThreshChance(mode, interval);
}else if(countRndSeq > minDel && mode == 1){
countRndSeq += calcThreshChance(mode, interval);
}else if(countRndSeq > minDel && mode == 2){
countRndSeq += calcThreshChance(mode, interval);
}
}
digitalWrite(randPin, HIGH);
}
/*
* Simplefunction that uses integers 8-13 and a boolean value to turn on or off all theLEDs at once
*/
void allPinsWrite(int p1, int p2, int p3, intp4, int p5, int p6, boolean writeInst){
digitalWrite(p1, writeInst);
digitalWrite(p2, writeInst);
digitalWrite(p3, writeInst);
digitalWrite(p4, writeInst);
digitalWrite(p5, writeInst);
digitalWrite(p6, writeInst);
}
/*
* Thefollowing function takes an integer 0-2 and calculates the chance for it to addmore to the number countRndSeq
* Firstit sets up three variables local to the function (chance for around 50, achance for around 200, and a chance for around 300)
* Thenthe following first check is made: check which mode is selected, 0, 1, or 2 (ifsomeone entered something else or not calculated it will return 0 and count bythe regular interval in the randomSequence)
* If itis mode 0, use a relatively short random range from 45 to 69 and check if thatrandom number falls between 50 and 60 which is a pretty high chance based onthe short range, so it will more than likely add 1000 plus that random numberto countRndSeq
* If itis mode 1, use a larger range from 200 to 299 and check if that random numberlies between 250 and 255, which is a medium chance, so it may or may not usethat random number + 2000
* If itis mode 2, use a much larger range from 300 to 599 and check if that randomnumber lies between 320 and 324, which is a low chance, so it has lesslikelihood of using that random number and adding 3000
* Allother cases it will just add the regular interval
*/
int calcThreshChance(int mode, int interval){
intrandCh50 = 0;
intrandCh200 = 0;
intrandCh300 = 0;
if(mode == 0){
randCh50 = random(45, 70);
if(randCh50 >= 50 && randCh50 <= 60){
return randCh50 + 1000;
}else {
return interval;
}
}else if (mode == 1){
randCh200 = random(200, 300);
if(randCh200 >= 250 && randCh200 <= 255){
return randCh200 + 2000;
}else {
return interval;
}
}else if (mode == 2){
randCh300 = random(300, 600);
if(randCh300 >= 320 && randCh300 <= 324){
return randCh300 + 3000;
}else {
return interval;
}
}else {
return interval;
}
}
//77 lines of code not including comments
向上滑動查看完整代碼
即使整篇代碼只減少了3行,但在希望試驗延遲值或者引腳是變高還是變低等情況下,你就能輕松快速地調整相關值。如果沒有函數,你每次都需要手動添加四行代碼。
初始代碼接線圖
使用函數的擴展代碼
void setup() {//Serial.begin(9600); //I often use the serial monitor to debug my program or to do some testing, I then comment the line of code to increase some runtime speed (counted this line of commented code as it was essential to my process)
pinMode(13, OUTPUT);
pinMode(12, OUTPUT);
pinMode(11, OUTPUT);
pinMode(10, OUTPUT);
pinMode(9, OUTPUT);
pinMode(8, OUTPUT);
}
int countRndSeq = 0; //set initial variable for counting variable for the random sequence to "reset"
int randPin = random(8, 14); //set the random Pin variable to a random number between 8 and 13 (14 for including 13 as a choice); we only need 8-13 as those are the pins I use
void loop() {
randSequenceLightSlow(5, 125, 1200); //run function for a random sequence, set counting interval at 5, set minimum delay at 125, set the max delay to 1200 (1.2 seconds) (I decided to move the randMode into the function because it was easier to accomplish)
countRndSeq = 0; //reset the counter for the next sequence
delay(3000); //wait three seconds then turn off all the lights (made a function for that)
allPinsWrite(13, 12, 11, 10, 9, 8 , LOW);
randSequenceLightSlow(7, 125, 1450); //run function for random sequence using counting interval at 7, minimum delay at 125, set max delay to 1450 (1.450 seconds)
countRndSeq = 0; //reset counter for next sequence
delay(3000); //wait three seconds and turn off all the lights
allPinsWrite(13, 12, 11, 10, 9, 8, LOW);
}
/*
* The following uses a while loop for control, it does random lights and slows down delay by a set interval between steps (larger interval will make it count and slow down much quicker)
* As long as that counting number (countRndSeq) is less than the max delay (maxDel), it will do the following in order:
* 1. chose a random pin 8-13 and set it equal to a variable
* 2. Pick the random Mode value each time the while loop runs again (increases chance to get modes 0-2)
* 2. turn off the pin associated to that number using the delay amount of the same number that countrRndSeq is currently at
* 3. Increase countRndSeq by the interval amount set by calling the function (can be any integer)
* 4. Check if the current count is greater than the minimum delay and then check which mode (remember I set it to random above); there are 3 modes 0, 1, 2
* NOTE: For #4, 0 has a high chance of adding less to countRndSeq, 1 has a medium chance of adding a bit more to countRndSeq, 2 has a low chance of adding quite a bit to countRndSeq
* 5. Set the final pin after the sequence has finished to high to simulate that is the final pin the program landed on
* See calcThreshChance for an explanation of how I made weighted random events for the chance for the lights to slow faster
*/
void randSequenceLightSlow(int interval, int minDel, int maxDel){
while(countRndSeq < maxDel){
randPin = random(8, 14);
int mode = random(0, 3);
digitalWrite(randPin, HIGH);
delay(countRndSeq);
digitalWrite(randPin, LOW);
delay(countRndSeq);
countRndSeq += interval;
if(countRndSeq > minDel && mode == 0){
countRndSeq += calcThreshChance(mode, interval);
} else if(countRndSeq > minDel && mode == 1){
countRndSeq += calcThreshChance(mode, interval);
} else if(countRndSeq > minDel && mode == 2){
countRndSeq += calcThreshChance(mode, interval);
}
}
digitalWrite(randPin, HIGH);
}
/*
* Simple function that uses integers 8-13 and a boolean value to turn on or off all the LEDs at once
*/
void allPinsWrite(int p1, int p2, int p3, int p4, int p5, int p6, boolean writeInst){
digitalWrite(p1, writeInst);
digitalWrite(p2, writeInst);
digitalWrite(p3, writeInst);
digitalWrite(p4, writeInst);
digitalWrite(p5, writeInst);
digitalWrite(p6, writeInst);
}
/*
* The following function takes an integer 0-2 and calculates the chance for it to add more to the number countRndSeq
* First it sets up three variables local to the function (chance for around 50, a chance for around 200, and a chance for around 300)
* Then the following first check is made: check which mode is selected, 0, 1, or 2 (if someone entered something else or not calculated it will return 0 and count by the regular interval in the randomSequence)
* If it is mode 0, use a relatively short random range from 45 to 69 and check if that random number falls between 50 and 60 which is a pretty high chance based on the short range, so it will more than likely add 1000 plus that random number to countRndSeq
* If it is mode 1, use a larger range from 200 to 299 and check if that random number lies between 250 and 255, which is a medium chance, so it may or may not use that random number + 2000
* If it is mode 2, use a much larger range from 300 to 599 and check if that random number lies between 320 and 324, which is a low chance, so it has less likelihood of using that random number and adding 3000
* All other cases it will just add the regular interval
*/
int calcThreshChance(int mode, int interval){
int randCh50 = 0;
int randCh200 = 0;
int randCh300 = 0;
if(mode == 0){
randCh50 = random(45, 70);
if(randCh50 >= 50 && randCh50 <= 60){
return randCh50 + 1000;
} else {
return interval;
}
} else if (mode == 1){
randCh200 = random(200, 300);
if(randCh200 >= 250 && randCh200 <= 255){
return randCh200 + 2000;
} else {
return interval;
}
} else if (mode == 2){
randCh300 = random(300, 600);
if(randCh300 >= 320 && randCh300 <= 324){
return randCh300 + 3000;
} else {
return interval;
}
} else {
return interval;
}
}
//77 lines of code not including comments
向上滑動查看完整代碼
這篇代碼的主題是在一定程度上模擬六面骰子的滾動,該骰子起先快速滾動,后來逐漸減慢到靜止不動。該代碼包含內置隨機函數,該函數使用了起始編號(包含在生成中)和在生成中排除的結束編號。它不僅隨機化了引腳(8-13),還隨機化了和一些骰子一樣明顯減速的幾率。我用了三種幾率“模式”來實現這一效果:高幾率:計數變量的增量較少;中等幾率:計數變量的增量稍多;低幾率:計數變量的增量較多(請參見代碼中的注釋,了解完整的代碼環境)。我創建了三個自定義函數,使這個概念更加易于理解和試驗。
無自定義函數的擴展代碼
void setup() {
pinMode(13, OUTPUT);
pinMode(12, OUTPUT);
pinMode(11, OUTPUT);
pinMode(10, OUTPUT);
pinMode(9, OUTPUT);
pinMode(8, OUTPUT);
}
int countRndSeq = 0;
int randPin = random(8,14);
int minDel1 = 125;
int maxDel1 = 1200;
int interval1 = 5;
int minDel2 = 125;
int maxDel2 = 1450;
int interval2 = 7;
int randCh50 = 0;
int randCh200 = 0;
int randCh300 = 0;
void loop() {
while(countRndSeq < maxDel1){
randPin = random(8, 14);
int randMode = random(0, 3);
digitalWrite(randPin, HIGH);
delay(countRndSeq);
digitalWrite(randPin, LOW);
delay(countRndSeq);
countRndSeq += interval1;
if(countRndSeq > minDel1 &&randMode == 0){
randCh50 = random(45, 70);
if(randCh50 >= 50 && randCh50<= 60){
countRndSeq += randCh50 + 1000;
} else {
countRndSeq += interval1;
}
} else if(countRndSeq > minDel1&& randMode == 1){
randCh200 = random(200, 300);
if(randCh200 >= 250 &&randCh200 <= 255){
countRndSeq += randCh200 + 2000;
} else {
countRndSeq += interval1;
}
} else if(countRndSeq > minDel1&& randMode == 2){
randCh200 = random(300, 600);
if(randCh200 >= 320 &&randCh200 <= 324){
countRndSeq += randCh200 + 3000;
} else {
countRndSeq += interval1;
}
} else {
countRndSeq += interval1;
}
}
digitalWrite(randPin, HIGH);
countRndSeq = 0;
delay(3000);
digitalWrite(13, LOW);
digitalWrite(12, LOW);
digitalWrite(11, LOW);
digitalWrite(10, LOW);
digitalWrite(9, LOW);
digitalWrite(8, LOW);
while(countRndSeq < maxDel2){
randPin = random(8, 14);
int randMode = random(0, 3);
digitalWrite(randPin, HIGH);
delay(countRndSeq);
digitalWrite(randPin, LOW);
delay(countRndSeq);
countRndSeq += interval2;
if(countRndSeq > minDel2 &&randMode == 0){
randCh50 = random(45, 70);
if(randCh50 >= 50 && randCh50<= 60){
countRndSeq += randCh50 + 1000;
} else {
countRndSeq += interval2;
}
} else if(countRndSeq > minDel2&& randMode == 1){
randCh200 = random(200, 300);
if(randCh200 >= 250 &&randCh200 <= 255){
countRndSeq += randCh200 + 2000;
} else {
countRndSeq += interval2;
}
} else if(countRndSeq > minDel2&& randMode == 2){
randCh200 = random(300, 600);
if(randCh200 >= 320 &&randCh200 <= 324){
countRndSeq += randCh200 + 3000;
} else {
countRndSeq += interval2;
}
} else {
countRndSeq += interval2;
}
}
digitalWrite(randPin, HIGH);
countRndSeq = 0;
delay(3000);
digitalWrite(13, LOW);
digitalWrite(12, LOW);
digitalWrite(11, LOW);
digitalWrite(10, LOW);
digitalWrite(9, LOW);
digitalWrite(8, LOW);
}
//105 lines of code not includingcomments
向上滑動查看完整代碼
注意,在實現相同效果的情況下,此部分的代碼數量顯著增加(函數版本的代碼少了28行,這在構建更大的程序時非常重要)。它不僅減少了代碼數量,而且這些函數的使用方式非常便于利用不同的值進行試驗。而手動編碼版本的代碼需要在代碼中更改若干變量才能進行試驗。想象一下,在需要進行大量試驗的情況下,如果進行每個操作都需要更改代碼,那將是多么的恐怖:而如果使用函數,那么只需編輯一個代碼塊即可快速測試功能。
擴展代碼接線圖
-
適合Arduino 和 Raspberry Pi 匹配的攝像頭
-
Arduino 睡眠模式示例
-
如何選擇適用于Arduino的IR、UV和可見光放射體
-
Arduino 擴展板
- Arduino setup() 和 loop() 函數的目的是什么?

提示點擊菜單設計支持:工程師錦囊,獲取更多工程師小貼士
秘技知識學不停 專屬福利享不停
就等您加入!
點此登記
賺積分、換好禮
立即到「會員權益」查看您的禮遇! 如有任何問題,歡迎聯系得捷電子DigiKey的客服團隊中國(人民幣)客服




中國(美金)/ 香港客服

400-882-4440


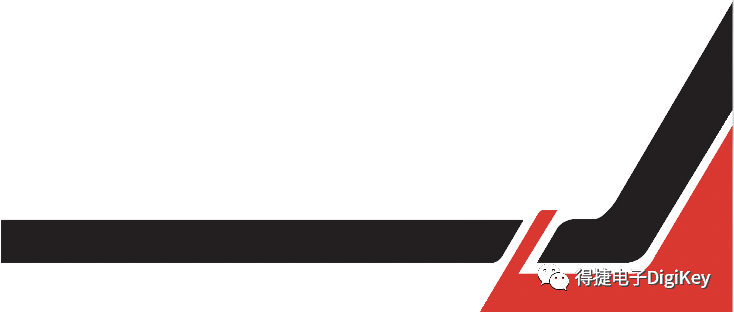
點擊下方“閱讀原文”查看更多
讓我知道你在看喲
原文標題:自己編寫函數示例代碼很難嗎?分享幾個示例!
文章出處:【微信公眾號:得捷電子DigiKey】歡迎添加關注!文章轉載請注明出處。
-
得捷電子
+關注
關注
1文章
255瀏覽量
8782
原文標題:自己編寫函數示例代碼很難嗎?分享幾個示例!
文章出處:【微信號:得捷電子DigiKey,微信公眾號:得捷電子DigiKey】歡迎添加關注!文章轉載請注明出處。
發布評論請先 登錄
相關推薦
HAL庫的函數調用示例
編寫高效Testbench的指南和示例
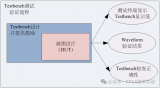
關于STM32 DMAMUX模塊具體的應用示例代碼
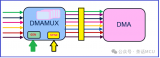
瑞薩Flash示例程序01型SC版本(代碼Flash)應用說明
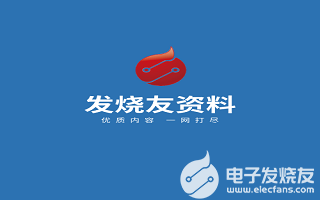
評論