相機組件支持相機業務的開發,開發者可以通過已開放的接口實現相機硬件的訪問、操作和新功能開發,最常見的操作如:預覽、拍照和錄像等。
基本概念
- 拍照
此功能用于拍攝采集照片。 - 預覽
此功能用于在開啟相機后,在緩沖區內重復采集攝像幀,支持在拍照或錄像前進行攝像幀預覽顯示。 - 錄像
此功能用于在開始錄像后和結束錄像前的時間段內,在緩沖區內重復采集攝像幀,支持視頻錄制。
圖 1 相機組件架構圖
目錄
倉目錄結構如下:
/foundation/multimedia/camera_framework # 相機組件業務代碼
├── frameworks # 框架代碼
│ ├── native # 內部接口實現
│ │ ├── camera # 相機框架實現
│ │ └── metadata # 元數據實現
│ └── js # 外部接口實現
│ └── camera_napi # 相機NAPI實現
├── interfaces # 接口代碼
│ ├── inner_api # 內部接口
│ └── kits # 外部接口
├── LICENSE # 許可證文件
├── ohos.build # 構建文件
├── sa_profile # 服務配置文件
└── services # 服務代碼
├── camera_service # 相機服務實現
└── etc # 相機服務配置
使用說明
拍照
拍照的步驟:
- 創建緩沖區消費者端監聽器(CaptureSurfaceListener)以保存圖像。
class CaptureSurfaceListener : public IBufferConsumerListener { public: int32_t mode_; sptr< Surface > surface_; void OnBufferAvailable() override { int32_t flushFence = 0; int64_t timestamp = 0; OHOS::Rect damage; // initialize the damage OHOS::sptr< OHOS::SurfaceBuffer > buffer = nullptr; surface_- >AcquireBuffer(buffer, flushFence, timestamp, damage); if (buffer != nullptr) { void* addr = buffer- >GetVirAddr(); int32_t size = buffer- >GetSize(); // Save the buffer(addr) to a file. surface_- >ReleaseBuffer(buffer, -1); } } };
- 獲取相機管理器實例并獲取相機對象列表。
sptr< CameraManager > camManagerObj = CameraManager::GetInstance(); std::vector< sptr< CameraInfo >> cameraObjList = camManagerObj- >GetCameras();
- 使用相機對象創建相機輸入來打開相機。
sptr< CaptureInput > cameraInput = camManagerObj- >CreateCameraInput(cameraObjList[0]);
- 創建采集會話。
sptr< CaptureSession > captureSession = camManagerObj- >CreateCaptureSession();
- 開始配置采集會話。
int32_t result = captureSession- >BeginConfig();
- 將相機輸入添加到采集會話。
result = captureSession- >AddInput(cameraInput);
- 創建消費者 Surface 并注冊監聽器以監聽緩沖區更新。拍照的寬和高可以配置為所支持的 1280x960 分辨率。
sptr< Surface > photoSurface = Surface::CreateSurfaceAsConsumer(); int32_t photoWidth = 1280; int32_t photoHeight = 960; photoSurface- >SetDefaultWidthAndHeight(photoWidth, photoHeight); photoSurface- >SetUserData(CameraManager::surfaceFormat, std::to_string(OHOS_CAMERA_FORMAT_JPEG)); sptr< CaptureSurfaceListener > capturelistener = new(std::nothrow) CaptureSurfaceListener(); capturelistener- >mode_ = MODE_PHOTO; capturelistener- >surface_ = photoSurface; photoSurface- >RegisterConsumerListener((sptr< IBufferConsumerListener > &)capturelistener);
- 使用上面創建的 Surface 創建拍照輸出。
sptr< CaptureOutput > photoOutput = camManagerObj- >CreatePhotoOutput(photoSurface);
- 將拍照輸出添加到采集會話。
result = captureSession- >AddOutput(photoOutput);
- 將配置提交到采集會話。
result = captureSession- >CommitConfig();
- 拍攝照片。
result = ((sptr PhotoOutput > &)photoOutput)- >Capture();
- 釋放采集會話資源。
captureSession- >Release();
- 釋放相機輸入關閉相機。
cameraInput- >Release();
開始和停止預覽
開始和停止預覽的步驟:
- 獲取相機管理器實例并獲取相機對象列表。
sptr< CameraManager > camManagerObj = CameraManager::GetInstance(); std::vector< sptr< CameraInfo >> cameraObjList = camManagerObj- >GetCameras();
- 使用相機對象創建相機輸入來打開相機。
sptr< CaptureInput > cameraInput = camManagerObj- >CreateCameraInput(cameraObjList[0]);
- 創建采集會話。
sptr< CaptureSession > captureSession = camManagerObj- >CreateCaptureSession();
- 開始配置采集會話。
int32_t result = captureSession- >BeginConfig();
- 將相機輸入添加到采集會話。
result = captureSession- >AddInput(cameraInput);
- 使用從窗口管理器獲得的 Surface 創建預覽輸出用以在顯示上渲染。預覽的寬和高可以配置為所支持的 640x480 或 832x480 分辨率,如果想保存到文件,可以按照拍照流程提到步驟,創建 Surface,注冊監聽器以監聽緩沖區更新。
int32_t previewWidth = 640; int32_t previewHeight = 480; previewSurface- >SetUserData(CameraManager::surfaceFormat, std::to_string(OHOS_CAMERA_FORMAT_YCRCB_420_SP)); sptr< CaptureOutput > previewOutput = camManagerObj- >CreateCustomPreviewOutput(previewSurface, previewWidth, previewHeight);
- 將預覽輸出添加到采集會話。
result = captureSession- >AddOutput(previewOutput);
- 將配置提交到采集會話。
result = captureSession- >CommitConfig();
- 開始預覽。
result = captureSession- >Start();
- 需要時停止預覽。
result = captureSession- >Stop();
- 釋放采集會話資源。
captureSession- >Release();
- 釋放相機輸入關閉相機。
cameraInput- >Release();
視頻錄像
視頻錄像的步驟:
- 獲取相機管理器實例并獲取相機對象列表。
sptr< CameraManager > camManagerObj = CameraManager::GetInstance(); std::vector< sptr< CameraInfo >> cameraObjList = camManagerObj- >GetCameras();
- 使用相機對象創建相機輸入來打開相機。
sptr< CaptureInput > cameraInput = camManagerObj- >CreateCameraInput(cameraObjList[0]);
- 創建采集會話。
sptr< CaptureSession > captureSession = camManagerObj- >CreateCaptureSession();
- 開始配置采集會話。
int32_t result = captureSession- >BeginConfig();
- 將相機輸入添加到采集會話。
result = captureSession- >AddInput(cameraInput);
- 通過 Surface 創建一個視頻輸出,來與音頻合成并保存到文件,Surface 通過 Recoder 獲取。如果想僅保存視頻緩沖數據到文件里,可以按照拍照流程提到步驟,創建 Surface,注冊監聽器以監聽緩沖區更新。錄像的分辨率可以在錄制器內配置為所支持的 1280x720 或 640x360 分辨率。
videoSurface- >SetUserData(CameraManager::surfaceFormat, std::to_string(OHOS_CAMERA_FORMAT_YCRCB_420_SP)); sptr< CaptureOutput > videoOutput = camManagerObj- >CreateVideoOutput(videoSurface);
- 將視頻輸出添加到采集會話。
result = captureSession- >AddOutput(videoOutput);
- 將配置提交到采集會話。
result = captureSession- >CommitConfig();
- 開始視頻錄制。
result = ((sptr VideoOutput > &)videoOutput)- >Start();
- 需要時停止錄制。
result = ((sptr VideoOutput > &)videoOutput)- >Stop();
- 釋放采集會話的資源。
captureSession- >Release();
- 釋放相機輸入關閉相機。
cameraInput- >Release();
切換多個照相機設備
以下演示如何切換多個照相機設備。最初在采集會話中有一個視頻輸出(video output)。如果用戶想要切換其他 照相機,現存的相機輸入和輸出需要先移除并加入新的相機輸入和輸出(示例中使用的是photo output)。
- 獲取相機管理器實例并獲取相機對象列表。
sptr< CameraManager > camManagerObj = CameraManager::GetInstance(); std::vector< sptr< CameraInfo >> cameraObjList = camManagerObj- >GetCameras();
- 使用相機對象創建相機輸入來打開相機。
sptr< CaptureInput > cameraInput = camManagerObj- >CreateCameraInput(cameraObjList[0]);
- 創建采集會話。
sptr< CaptureSession > captureSession = camManagerObj- >CreateCaptureSession();
- 開始配置采集會話。
int32_t result = captureSession- >BeginConfig()
- 將相機輸入添加到采集會話。
result = captureSession- >AddInput(cameraInput);
- 通過Surface創建一個視頻輸出。
sptr< CaptureOutput > videoOutput = camManagerObj- >CreateVideoOutput(videoSurface);
- 將視頻輸出添加到采集會話。
result = captureSession- >AddOutput(videoOutput);
- 將配置提交到采集會話。
result = captureSession- >CommitConfig();
- 開始錄制視頻。
result = ((sptr VideoOutput > &)videoOutput)- >Start();
- 需要時停止錄制。
result = ((sptr VideoOutput > &)videoOutput)- >Stop();
- 重新配置會話并移除相機輸入和輸出。
int32_t result = captureSession- >BeginConfig();
- 在新的會話配置中移除相機輸入。
int32_t result = captureSession- >RemoveInput(cameraInput);
- 同樣移除相機輸出。
int32_t result = captureSession- >RemoveOutut(videoOutput);
- 創建新的相機輸入,并把它添加到采集會話。
sptr< CaptureInput > cameraInput2 = camManagerObj- >CreateCameraInput(cameraObjList[1]);
result = captureSession- >AddInput(cameraInput2);
- 創建拍照輸出,成功創建后將拍照輸出添加到采集會話。創建消費者 Surface 并注冊監聽器以監聽新的拍照輸出緩沖區更新。這個 Surface 用于新創建的拍照輸出。
// Get the surface
sptr< Surface > photoSurface = Surface::CreateSurfaceAsConsumer();
int32_t photoWidth = 1280;
int32_t photoHeight = 960;
photoSurface- >SetDefaultWidthAndHeight(photoWidth, photoHeight);
photoSurface- >SetUserData(CameraManager::surfaceFormat, std::to_string(OHOS_CAMERA_FORMAT_JPEG));
sptr< CaptureSurfaceListener > capturelistener = new(std::nothrow) CaptureSurfaceListener();
capturelistener- >mode_ = MODE_PHOTO;
capturelistener- >surface_ = photoSurface;
photoSurface- >RegisterConsumerListener((sptr< IBufferConsumerListener > &)capturelistener);
// Create the Photo Output
sptr< CaptureOutput > photoOutput = camManagerObj- >CreatePhotoOutput(photoSurface);
// Add the output to the capture session
result = captureSession- >AddOutput(photoOutput);
- 將配置提交到采集會話。
result = captureSession- >CommitConfig();
- 釋放被移出會話的相機輸入。
cameraInput- >Release();
- 拍攝照片。
result = ((sptr PhotoOutput > &)photoOutput)- >Capture();
- 釋放采集會話資源。
captureSession- >Release();
- 釋放相機輸入關閉相機。
cameraInput2- >Release();
設置閃光燈
拍照和錄像前可以在相機輸入里設置閃光燈。
在照相中設置閃光燈。
cameraInput- >LockForControl(); cameraInput- >SetFlashMode(OHOS_CAMERA_FLASH_MODE_OPEN); cameraInput- >UnlockForControl();
在錄像中設置閃光燈。
cameraInput- >LockForControl(); cameraInput- >SetFlashMode(OHOS_CAMERA_FLASH_MODE_ALWAYS_OPEN); cameraInput- >UnlockForControl();
關閉閃光燈。
cameraInput- >LockForControl(); cameraInput- >SetFlashMode(OHOS_CAMERA_FLASH_MODE_CLOSE); cameraInput- >UnlockForControl();
聲明:本文內容及配圖由入駐作者撰寫或者入駐合作網站授權轉載。文章觀點僅代表作者本人,不代表電子發燒友網立場。文章及其配圖僅供工程師學習之用,如有內容侵權或者其他違規問題,請聯系本站處理。
舉報投訴
-
Camera
+關注
關注
0文章
79瀏覽量
20838 -
鴻蒙
+關注
關注
57文章
2369瀏覽量
42900
發布評論請先 登錄
相關推薦
鴻蒙Flutter實戰:14-現有Flutter 項目支持鴻蒙 II
分別安裝官方的3.22版本,以及鴻蒙社區的 3.22.0 版本
3.搭建 Flutter鴻蒙開發環境
參考文章《鴻蒙Flutter實戰:0
發表于 12-26 14:59
鴻蒙Flutter實戰:08-如何調試代碼
# 鴻蒙Flutter實戰:如何調試代碼
## 1.環境搭建
參考文章[鴻蒙Flutter實戰:01-搭建開發環境](https://g
發表于 10-23 16:29
鴻蒙Flutter實戰:07混合開發
# 鴻蒙Flutter實戰:混合開發
鴻蒙Flutter混合開發主要有兩種形式。
## 1.基于har
將flutter module
發表于 10-23 16:00
HarmonyOS實戰開發-如何使用全局狀態保留能力彈窗來實現評論組件。
開發有幫助,我想邀請大家幫我三個小忙:
點贊,轉發,有你們的 『點贊和評論』,才是我創造的動力。
關注小編,同時可以期待后續文章ing?,不定期分享原創知識。
更多鴻蒙最新技術知識點,請關注作者博客:鴻蒙
發表于 05-07 15:06
OpenHarmony實戰開發-如何實現組件動畫。
ArkUI為組件提供了通用的屬性動畫和轉場動畫能力的同時,還為一些組件提供了默認的動畫效果。例如,List的滑動動效,Button的點擊動效,是組件自帶的默認動畫效果。在組件默認動畫效
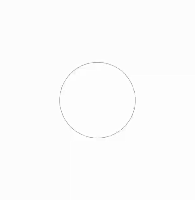
鴻蒙開發實戰:【藍牙組件】
藍牙服務組件為設備提供接入與使用Bluetooth的相關接口,包括BLE設備gatt相關的操作,以及BLE廣播、掃描等功能。
鴻蒙實戰項目開發:【短信服務】
、OpenHarmony 多媒體技術、Napi組件、OpenHarmony內核、Harmony南向開發、鴻蒙項目實戰等等)鴻蒙(Harmon
發表于 03-03 21:29
鴻蒙開發實戰-(ArkUI)List組件和Grid組件的使用
一系列相同寬度的列表項,連續、多行呈現同類數據,例如圖片和文本。常見的列表有線性列表(List列表)和網格布局(Grid列表):
為了幫助開發者構建包含列表的應用,ArkUI提供了List組件和Grid
發表于 01-18 20:18
鴻蒙開發OpenHarmony組件復用案例
)
}
}, item => item)
}
}
}
本文主要是對鴻蒙開發基礎當中的OpenHarmony技術組件復用示例, 更多鴻蒙開發
發表于 01-15 17:37
鴻蒙開發基礎-Web組件之cookie操作
})
...
}
...
本文章主要是對鴻蒙開發當中ArkTS語言的基礎應用實戰,Web組件里的cookie操作。更多的鴻蒙應用
發表于 01-14 21:31
鴻蒙基礎開發實戰-(ArkTS)像素轉換
的使用。通過像素轉換案例,向開發者講解了如何使用像素單位設置組件的尺寸、字體的大小以及不同像素單位之間的轉換方法。更多鴻蒙4.0的學習,可以前往主頁學習或前往《鴻蒙4.0
發表于 01-11 16:53
評論