我們在工作中會經常遇到線程同步,那么到底什么是線程同步呢,線程同步的本質是什么,線程同步的方法又有哪些,為什么會有這些方法呢?在回答這些問題之前,我們先做幾個名詞解釋,以便建立共同的概念基礎。
2022-08-25 11:49:43
464 互斥:多線程中互斥是指多個線程訪問同一資源時同時只允許一個線程對其進行訪問,具有唯一性和排它性。但互斥無法限制訪問者對資源的訪問順序,即訪問是無序的;
2023-03-20 09:09:25
1293 線程是輕量級的進程(`LWP: Light Weight Process`),在`Linux`環境下線程的本質仍是`進程`,進程是資源分配的`最小單位`,線程是操作系統調度執行的`最小單位`。
2023-07-14 16:41:43
450 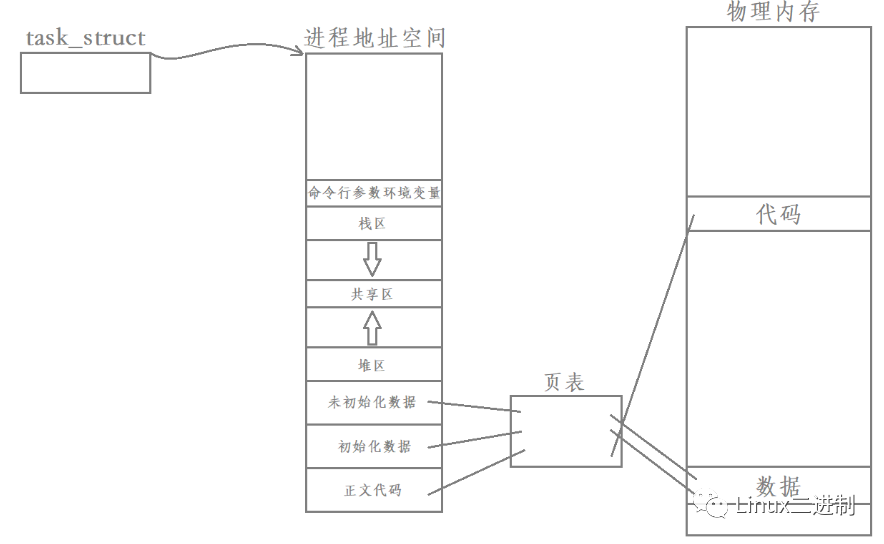
本文將介紹一下Java多線程的用法。 基礎介紹 什么是多線程 指的是在一個進程中同時運行多個線程,每個線程都可以獨立執行不同的任務或操作。 與單線程相比,多線程可以提高程序的并發性和響應
2023-09-30 17:07:00
561 Linux中最流行的線程機制為LinuxThreads,下面以一個多線程編程實例來介紹該線程庫的編程接口: 示例1:線程的創建和退出,等待線程結束和獲取線程的返回值 #include
2018-10-17 09:32:16
Linux c多線程編程的4個實例 在主流的操作系統中,多任務一般都提供了進程和線程兩種實現方式,進程享有獨立的進程空間,而線程相對于進程來說是一種更加輕量級的多任務并行,多線程之間一般都是共享
2020-06-09 04:35:40
。同一進程內的線程共享進程的地址空間。通信:進程間通信IPC,線程間可以直接讀寫進程數據段(如全局變量)來進行通信——需要進程同步和互斥手段的輔助,以保證數據的一致性。調度和切換:線程上下文切換比進程
2017-12-08 14:14:06
Linux多線程編程手冊
2016-11-07 10:17:40
1 線程不能獨立運行,要依附于進程2 如果創建一個子線程只需要重新分配棧空間3 多個線程可以并行運行4 線程之間可以有共同的全局變量(全局區,任何線程都可以訪問)5 多線程效率高如何創建子線程(在
2016-11-11 09:53:39
1 線程不能獨立運行,要依附于進程 2 如果創建一個子線程只需要重新分配??臻g 3 多個線程可以并行運行 4 線程之間可以有共同的全局變量(全局區, 任何線程都可以訪問) 5 多線程效率高
2017-01-10 14:59:47
最近研究MySQL源碼,各種鎖,各種互斥,好在我去年認真學了《unix環境高級編程》, 雖然已經忘得差不多了,但是學過始終是學過,拿起來也快。寫這篇文章的目的就是總結Linux 下多線程編程,作為日后的參考資料。
2019-07-23 08:17:14
Linux下提供了多種方式來處理線程同步,最常用的是互斥鎖、條件變量和信號量。
2019-07-19 07:24:51
linux多線程環境下gettid() pthread_self() 兩個函數都獲得線程ID,但這2個ID有所不同gettid是內核中的線程的ID:POSIX thread ID可以在一個進程內唯一
2019-07-09 08:36:48
*arg) 用法:#include 功能:pthread_create是類Unix操作系統(Unix、Linux、Mac OS X等)的創建線程的函數 說明:函數若線程創建成功,則返回0,若線程創建失敗
2018-06-27 08:36:01
最近研究mysql源碼,各種鎖,各種互斥,好在我去年認真學了《unix環境高級編程》, 雖然已經忘得差不多了,但是學過始終是學過,拿起來也快。寫這篇文章的目的就是總結linux 下多線程編程,作為日后的參考資料。
2019-08-06 06:15:28
在linux下運行多線程程序,想查看各個線程的運行情況,怎么辦?
2019-07-22 07:27:33
嗎? 怎么設計??若互鎖的話。。。就不能實現 B.D線程間的數據實時 共享了(在B 進程中 while循環采集數據,在采樣時是會阻塞在那 , 獲取數據 返回給 結構體相應變量,若互鎖的話。D就會 等B采樣完畢,才 更新屏幕數據的?。。?????怎么辦?還有這么好的線程間共享數據的辦法??????
2013-05-17 13:26:19
1、多線程了解線程之前我們必須要先了解(程序—>進程—>線程)的過程程序:是一組計算機能識別和執行的指令,運行于電子計算機上,滿足人們某種需求的信息化工具。進程:進程指正在運行的程序
2021-08-24 08:28:00
(6000); //延時6秒} 編譯并運行應用程序,單擊“延時6秒”按鈕,你就會發現在這6秒期間程序就象“死機”一樣,不在響應其它消息。為了更好地處理這種耗時的操作,我們有必要學習——多線程編程。二
2008-10-22 11:41:12
多線程編程之三 線程間通訊七、線程間通訊 一般而言,應用程序中的一個次要線程總是為主線程執行特定的任務,這樣,主線程和次要線程間必定有一個信息傳遞的渠道,也就是主線程和次要線程間要進行通信。這種
2008-10-22 11:43:09
多線程編程之四 線程的同步八、線程的同步 雖然多線程能給我們帶來好處,但是也有不少問題需要解決。例如,對于像磁盤驅動器這樣獨占性系統資源,由于線程可以執行進程的任何代碼段,且線程的運行是由系統調度
2008-10-22 11:43:42
使用方法節點實現多線程,兩個線程之間的數據傳輸也都使用方法節點的方式實現。1、初始化時打開另一個線程。2、程序運行過程中實現對被調線程的實施讀寫。3、Write data按下后寫入對應的指令到被調線程得指令接收端。4、主程序停止時關掉被調線程。
2020-07-06 17:21:20
rt_thread,下面要介紹線程間的同步與通信,線程間同步對象rt_sem / rt_mutex / rt_event和線程間通信對象rt_mb / rt_mq都直接繼承自rt_ipc_objec...
2021-07-02 06:15:04
進行的更快。事實上,他們可能運行的更慢,因為操作系統要花額外的時間進行線程間的切換。在多處理器系統中,多線程在多數情況下都是利大于弊的,因為它允許多個耗CPU的線程同時運行。但是,在處理器上調度線程
2022-02-01 13:14:37
LabView的多線程語言以前只會照貓畫虎的寫一些簡單的程序,一些基本原理不是很清晰。從網上找了一些資料,這里總結一下。1。一般情況下,運行一個 VI,至少有兩個線程:一個界面線程(UI
2009-06-08 10:13:49
C++ 多線程(一)Multi-Threaded多線程編程術語線程更確切地說,是執行線程,它是最小的處理單元。由操作系統調度。通常它包含在進程中。因此,同一個進程中可以存在多個線程。它與進程共享資源
2021-08-24 08:31:05
Python多線程類似于同時執行多個不同程序,但其執行過程中和進程還是有區別的,每個獨立的線程有一個程序運行的入口、順序執行序列和程序的出口,但是線程不能夠獨立執行,必須依存在應用程序中,由應用程序
2018-11-22 14:01:58
,對ASR程序結構有一定的了解。前面有些代碼已經使用了線程,可見線程在ASR程序設計里面的重要性。不禁心里會有些疑問,線程工作原理是什么?多線程誰會先執行?多線程之間是否可以進行信息交換?信息交換是不是隊列
2021-07-02 16:27:40
labview多線程技術
2017-01-10 16:00:37
子曰:何為labview多線程編程?
2015-03-11 15:46:07
段可以干多件事,譬如可以邊吃飯邊看電視;在Python中,多線程 和 協程 雖然是嚴格上來說是串行,但卻比一般的串行程序執行效率高得很。 一般的串行程序,在程序阻塞的時候,只能干等著,不能去做其他事
2022-03-15 16:42:20
利用線程的互斥實現串口多線程收發數據從而達到流水燈的效果。多線程串口編程主要分為三步,第一部分,連接串口及開發板,確定設備號;第二部分為串口參數的設置;第三部分為多線程數據的收發。下方有完整代碼實現
2022-01-07 08:08:26
Linux系統編程第07期:多線程編程入門 6年嵌入式開發經驗,在多家半導體...
2021-12-23 08:08:42
嵌入式Linux多線程編程-學習資源-華清遠見清遠見嵌入式學院:清遠見嵌入式學院:《嵌入式應用程序設計》——第5 章 嵌入式Linux 多線程編程第5 章 嵌入式Linux 多線程編程本章
2021-11-05 06:54:35
是對 RT-Thread 多線程學習后的總結,并嘗試從如圖所示的以下幾個方面進行總結。多線程之間同步是繼多線程學習之后,需要重點掌握的又一個重要內容。一個實時操作系統里面,如果只有多線程而沒有線程間同步,各個線程都無序
2022-03-18 15:46:09
首先Linux并不存在真正的線程,Linux的線程是使用進程模擬的。當我們需要在一個進程中同時運行多個執行流時,我們并不可以開辟多個進程執行我們的操作(32位機器里每個進程認為它 獨享 4G的內存
2019-07-23 06:10:56
問題,那就沒有這么簡單了,選的不好,會讓你深受其害。 經常在網絡上看到有的XDJM問“多進程好還是多線程好?”、“Linux下用多進程還是多線程?”等等期望一勞永逸的問題,我只能說:沒有最好,只有更好。根據實際...
2021-08-24 07:38:57
如何使用多線程
2020-11-10 08:08:25
C++面向對象多線程編程共分13章,全面講解構建多線程架構與增量多線程編程技術。第1章介紹了
2008-09-25 09:39:36
0 介紹了QNX 實時操作系統和多線程編程技術,包括線程間同步的方法、多線程程序的分析步驟、線程基本程序結構以及實用編譯方法。QNX 是由加拿大QNX 軟件有限系統公司開發的
2009-08-12 17:37:19
30 采用多進程處理多個任務,會占用很多系統資源(主要是CPU 和內存的使用)。在LINUX 中,則對這種弊端進行了改進,在用戶態實現了多線程處理多任務。本文系統論述了多線程間
2009-08-13 08:31:15
20 本文通過一機房監控系統程序中串口通信對多線程的應用來介紹Windows 9X/NT操作系統中多線程的應用和VC++對多線程的支持。關健詞: 多線程,串口通信在現代的各種實時監控系
2009-09-03 11:45:29
27 首先介紹了多線程技術的基本原理,然后討論了多線程技術在串口通信中的應用,并給出了實現的方法和步驟。關鍵詞:多線程;串口通信;事件
2009-09-04 09:10:17
18 在多線程偵聽I/O 事件時,我們必須處理好多線程和多I/O 句柄之間的關系,既要盡量減少線程同步的開銷,有要保證I/O 的安全性,傳統技術在這里遇到巨大的困難。為了解決這個
2010-01-09 13:50:27
9 介紹了一個多串口通信模塊,該模塊采用VC++6.0并結合多線程技術編寫,用來處理從遠程終端站上傳來數據。同時良好的線程同步解決方法也保證了模塊程序能夠運行更可靠,數據
2010-02-21 15:52:21
35 介紹了一個多串口通信模塊,該模塊采用VC++6.0并結合多線程技術編寫,用來處理從遠程終端站上傳來數據。同時良好的線程同步解決方法也保證了模塊程序能夠運行更可靠,數據的
2010-07-22 17:38:24
37 介紹了在基于 SWT 的C / S 結構的項目開發中,當用UI 主線程進行后臺數據讀取或交換時導致的UI 線程堵塞現象的解決方案。通過對UI 線程的深入了解,利用多線程技術,將前臺顯示和后
2011-06-07 17:08:14
0 電子發燒友為您提供了linux多線程編程課件,希望對您學習 linux 有所幫助。部分內容如下: *1、多線程模型在單處理器模型和多處理器系統上,都能改善響應時間和吞吐量。 *2、線程包
2011-07-10 11:58:43
0
在線程對共享相同內存操作時,就會出現多個線程對同一資源的使用,為此,需要對這些線程進行同步,以確保它們在訪問共享內存的時候不會訪問到無效的數值。
2011-08-08 14:17:16
1946 簡要介紹了在Win32環境下多線程訪問共享資源時的同步機制,討論了主要的4種同步對象(臨界區、互斥元、事件、信號量),并描述了它們的優缺點,給出了使用Win32 API函數操控這4種對
2011-11-14 10:55:54
31 本文中我們針對 Linux 上多線程編程的主要特性總結出 5 條經驗,用以改善 Linux 多線程編程的習慣和避免其中的開發陷阱。在本文中,我們穿插一些 Windows 的編程用例用以對比 Linux 特性
2011-12-26 14:24:44
55 計算機上的上位機制作工具語言之MFC下的多線程編程
2016-09-01 14:55:49
0 多線程集合及IO面試
2017-02-27 19:11:17
0 Linux下多線程的視頻圖像平滑度評價算法_饒鴻
2017-03-19 11:27:34
0 多線程程序的編寫,多線程應用中容易出現的問題?;コ鈱ο蟮闹v解,如何采用互斥對象來實現多線程的同步。如何利用命名互斥對象保證應用程序只有一個實例運行。應用多線程編寫網絡聊天室程序。
2017-05-16 15:22:53
0 如果您的微控制器應用程序需要處理數字音頻,請考慮采用多線程方法。使用多線程設計方法可以使設計者以簡單的方式重用其部分設計。
2017-08-14 15:42:12
9 操作,一個取100塊,一個存錢100塊。假設賬戶原本有0塊,如果取錢線程和存錢線程同時發生,會出現什么結果呢?取錢不成功,賬戶余額是100.取錢成功了,賬戶余額是0.那到底是哪個呢?很難說清楚。因此多線程同步就是要解決這個
2017-09-27 13:19:40
0 多處理機、多核心處理器以及芯片級多處理或同時多線程處理器。本文為大家介紹多線程在Linux環境下的編程及在實際環境中的應用。 多線程技術在數據實時采集分析中的應用 本文介紹的多線程、內存映射文件和兩級緩沖的方法在高速
2017-10-16 16:46:55
0 9.2 Linux線程編程 9.2.1 線程基本編程 這里要講的線程相關操作都是用戶空間中的線程的操作。在Linux中,一般pthread線程庫是一套通用的線程庫,是由POSIX提出的,因此具有很好
2017-10-18 15:55:26
3 處理器都朝同時具有多核多線程的路線發展邁進。 雖然兩詞到處可見,但可有人知此二者的實際差異?在執行設計時又是以何者為重?到底是該多核優先還是多線程提前?關于此似乎大家都想進一步了解,本文以下試圖對此進行個中差異
2017-10-19 16:26:52
0 (process)中只允許有一個線程,這樣多線程就意味著多進程?,F在,多線程技術已經被許多操作系統所支持,包括Windows/NT,當然,也包括Linux。 為什么有了進程的概念后,還要再引入線程呢?使用多線程到底有哪些好處?什么的系統應該選用多線程?我們首先必須回答這些問題。 使
2017-10-24 16:01:39
5 這一次我們要說下關于final在多線程的作用,原子性的使用,死鎖以及Java中的應對方案,線程的局部變量 和 讀寫鎖的介紹 。關于final變量在多線程的使用 我們如今已經了解到,除非
2017-11-28 15:34:30
991 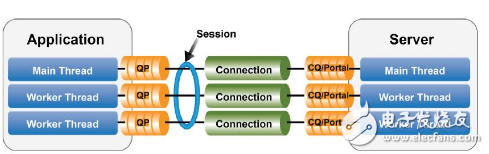
摘要:如今單線程與多線程已經得到普遍運用,那么到底多線程好還是單線程好呢?單線程和多線程的區別又是什么呢?下面我們來看看它們的區別以及優缺點分析。
2017-12-08 09:33:15
79710 摘要:本文主要以MFC多線程為中心,分別對MFC多線程的實例、MFC多線程之間的通信展開的一系列研究,下面我們來看看原文。
2017-12-08 15:23:43
17054 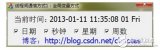
摘要:多線程編程是現代軟件技術中很重要的一個環節。要弄懂多線程,這就要牽涉到多進程。本文主要以多線程編程以及多線程編程相關知識而做出的一些結論。
2017-12-08 16:30:08
11929 本文對多線程服務器的常用編程模型進行了一個詳細的解讀,本文中的多線程服務器是運行在 Linux 操作系統上網絡應用程序。介紹了典型的單線程服務器編程模型和典型的多線程服務器的線程模型以及進程間通信與線程間通信等相關內容。
2018-02-19 08:29:00
6891 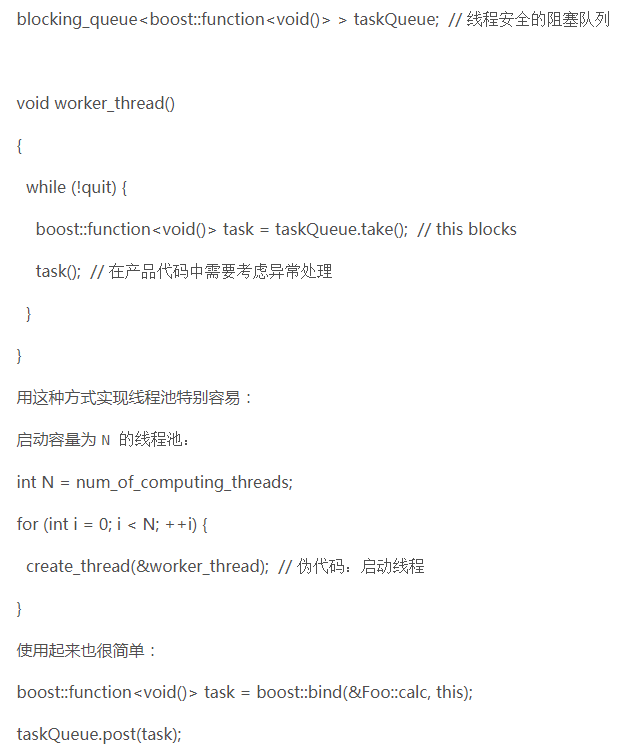
Linux下多線程編程技術 作為一個IT人員,不斷的學習和總結是我們這個職業習慣,所以我會將每個階段的學習都會通過一點的總結來記錄和檢測自己的學習效果,今天為大家總結了關于Linux下多線程編程技術。
2018-04-22 03:12:02
2051 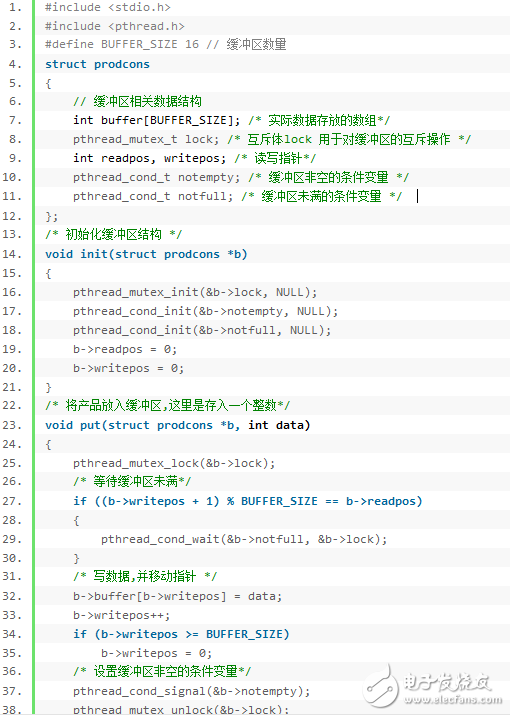
C#和.NET類庫為開發多線程應用程序提供了很方便的支持,本章首先簡要介紹.NET類庫中的Thread類及各種線程支持,再通過示例說明線程使用中需要掌握的規則,最后論述線程同步時出現的問題。 多線程
2018-04-23 11:32:05
14 在單線程程序中,整個程序都是順序執行的,一個函數在同一時刻只能被一個函數調用,但在多線程中,由于并發性,一個函數可能同時被多個函數調用,此時這個函數就成了臨界資源,很容易造成調用函數處理結果
2019-05-16 17:41:58
813 ,線程調度、同步與互斥都需要用戶程序自己完成。內核級線程需要內核參與,由內核完成線 程調度并提供相應的系統調用,用戶程序可以通過這些接口函數對線程進行一定的控制和管理。Linux操作系統提供
2019-04-02 14:42:43
329 嵌入式Linux中文站,關于多進程和多線程,教科書上最經典的一句話是“進程是資源分配的最小單位,線程是CPU調度的最小單位”。這句話應付考試基本上夠了,但如果在工作中遇到類似的選擇
2019-04-02 14:42:58
352 一個進程(process)中只允許有一個線程,這樣多線程就意味著多進程?,F在,多線程技術已經被許多操作系統所支持,包括Windows/NT,當然,也包括Linux。 為什么有了進程的概念后,還要再引入
2019-04-02 14:43:07
465 多線程開發在 Linux 平臺上已經有成熟的 Pthread 庫支持。其涉及的多線程開發的最基本概念主要包含三點:線程,互斥鎖,條件。其中,線程操作又分線程的創建,退出,等待
2019-04-02 14:45:11
227 的UNIX系統,但Linux的多線程在邏輯和使用上與真正的多線程并沒有差別。?多線程我們先來看一下什么是多線程。在Linux從程序到進程中,我們看到了一個程序在內存中的表示。這個程序的整個運行過程中,只有
2019-04-02 14:47:58
316 嵌入式linux中文站給大家介紹三種Linux中的常用多線程同步方式:互斥量,條件變量,信號量。
2019-05-02 14:49:00
2873 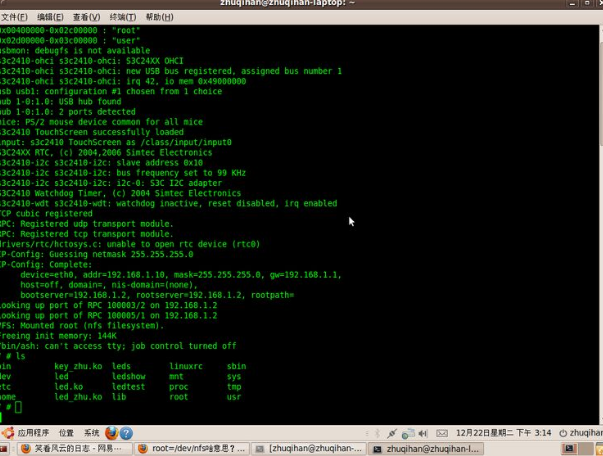
從這篇開始將會開啟高性能、高并發系列,本篇是該系列的開篇,主要關注多線程以及線程池。 一切要從CPU說起 你可能會有疑問,講多線程為什么要從CPU說起呢?原因很簡單,在這里沒有那些時髦的概念,你可以
2021-03-02 13:48:12
1756 嵌入式Linux多線程編程-學習資源-華清遠見清遠見嵌入式學院:清遠見嵌入式學院:《嵌入式應用程序設計》——第5 章 嵌入式Linux 多線程編程第5 章 嵌入式Linux 多線程編程本章
2021-11-02 13:36:16
7 arduino scoop多線程實際上arduino沒有多線程,所謂的多線程可能只是多任務。在同時運行兩個較為簡單的或者是執行周期很短的程序時應該是沒問題的。程序較為復雜,或者需要放到某一線程
2021-12-06 09:51:10
8 多線程之間同步是繼多線程學習之后,需要重點掌握的又一個重要內容。一個實時操作系統里面,如果只有多線程而沒有線程間同步,各個線程...
2022-01-25 18:52:59
0 MFC中有兩類線程,分別稱之為工作者線程和用戶界面線程。二者的主要區別在于工作者線程沒有消息循環,而用戶界面線程有自己的消息隊列和消息循環。
2022-06-01 17:03:38
0 單核CPU上所謂的”多線程”那是假的多線程,同一時間處理器只會處理一段邏輯,只不過線程之間切換得比較快,看著像多個線程”同時”運行罷了。
2022-08-11 15:47:17
1498 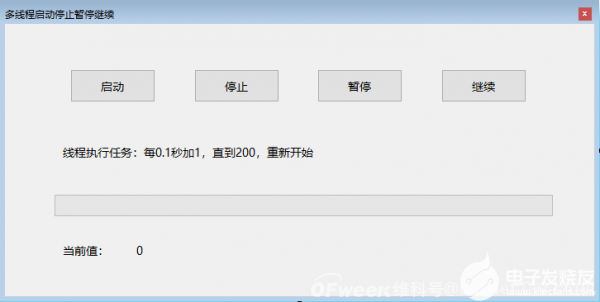
1. 功能說明 2. 多線程任務示例 2.1 線程池 2.2 單個任務 2.3 任務入口 2.4 結果分析 2.5 源碼地址 3. 寫在最后 大家好,今天教大家擼一個 Java 的多線程永動任務
2022-10-19 11:46:28
753 SpringBoot實現多線程
2023-01-12 16:59:22
1241 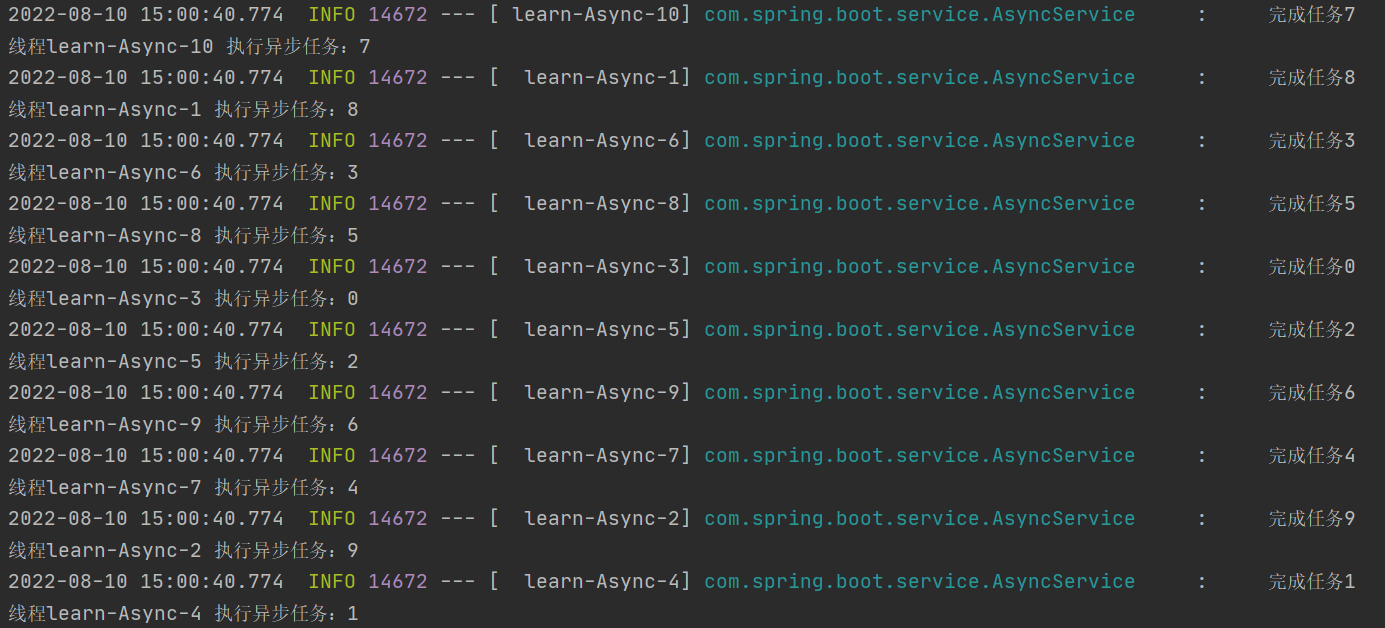
線程池通常用于服務器應用程序。 每個傳入請求都將分配給線程池中的一個線程,因此可以異步處理請求,而不會占用主線程,也不會延遲后續請求的處理
2023-02-28 09:53:49
420 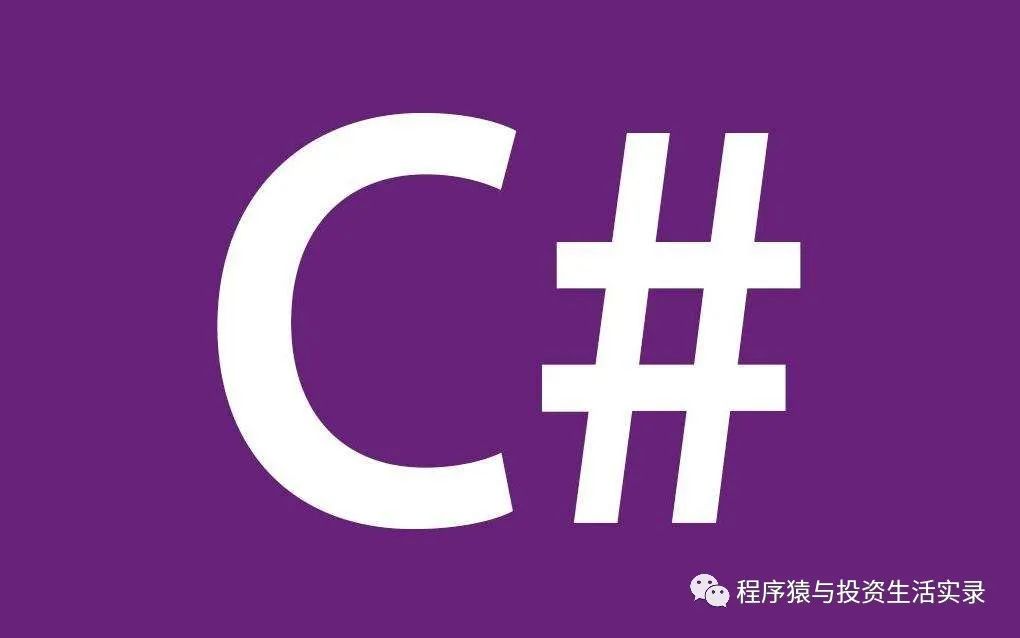
學習多線程最典型的問題就是如何在多個線程之間傳遞消息與寫作,PyQT5的線程支持在不同線程之間傳遞信號觸發事件,實現多個線程之間的協助,完成諸如生產者-消費者這樣經典的多線程協作。本文將通過QThread與信號槽機制構建一個生產者-消費者模型,演示多個線程之間的協作。
2023-03-08 14:58:00
802 Hello、Hello大家好,我是木榮,今天我們繼續來聊一聊Linux中多線程編程中的重要知識點,詳細談談多線程中同步和互斥機制。
2023-04-26 17:27:44
466 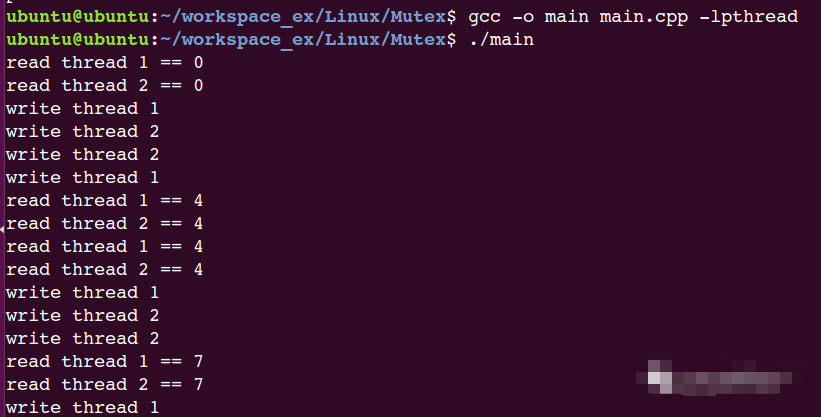
前言:應用程序在某些情況下需要處理比較復雜的邏輯,例如常規的圖傳上位機,如果在傳輸圖片跑到較高碼流或對圖像執行一些處理任務是,引用多線程可以明顯 改善響應度和反饋速度。
QT多線程使用的注意事項
2023-05-09 11:47:34
1 在spring中可以使用@Transactional注解去控制事務,使出現異常時會進行回滾,在多線程中,這個注解則不會生效,如果主線程需要先執行一些修改數據庫的操作,當子線程在進行處理出現異常時,主線程修改的數據則不會回滾,導致數據錯誤。
2023-08-09 12:22:05
360 
labview_AMC多線程
2023-08-21 10:31:44
20 多線程idm下載軟件
2023-10-23 09:23:27
0 在Linux系統中提供了多種同步機制,本文主要講講如何使用pthread_barrier_xxx系列函數來實現多線程之間進行同步的方法。
2023-10-23 14:43:06
237 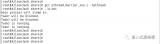
多線程同步是指在多個線程并發執行的情況下,為了保證線程執行的正確性和一致性,需要采用特定的方法來協調線程之間的執行順序和共享資源的訪問。下面將介紹幾種常見的多線程同步方法。 互斥鎖(Mutex
2023-11-17 14:16:19
412 多線程編程是一種并發編程的方法,意味著程序中同時運行多個線程,每個線程可獨立執行不同的任務,共享同一份數據。由于多線程并發執行的特點,會引發數據同步的問題,即保證多個線程對共享數據的訪問順序和正確性
2023-11-17 14:22:09
240 (圖形用戶界面)應用程序的開發。在這篇文章中,我們將重點介紹MFC中的多線程編程。 多線程編程在軟件開發中非常重要,它可以實現程序的并發執行,提高程序的效率和響應速度。MFC提供了豐富的多線程支持,可以輕松地實現多線程編程,并解決線程間的同步和通信問題。 首先,讓我們看一個簡單的MFC多線程
2023-12-01 14:29:20
400 linux線程
2024-02-15 21:16:35
13 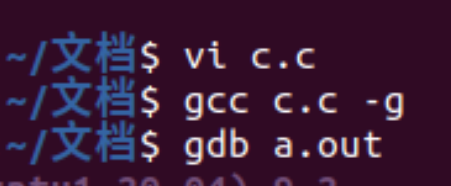
Java實現多線程的幾種方式 多線程是指程序中包含了兩個或以上的線程,每個線程都可以并行執行不同的任務或操作。Java中的多線程可以提高程序的效率和性能,使得程序可以同時處理多個任務。 Java提供
2024-03-14 16:55:02
99
正在加载...
評論